Simpe Mobile Signature Pad with jQuery and Html5 Canvas
File Size: | 2.2 KB |
---|---|
Views Total: | 27481 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
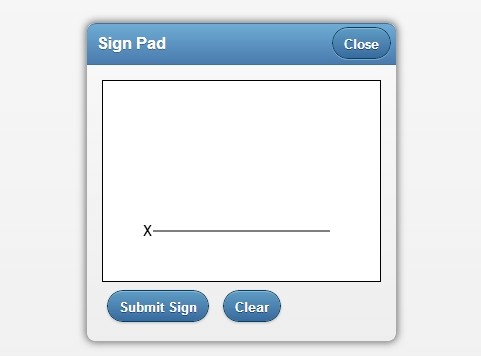
Signature Pad HTML5 is a jQuery, jQuery mobile and Html5 canvas
based mobile signature pad that allows to draw signature and save it as a PNG for later download.
See also:
Basic Usage:
1. Load the jQuery javascript library and jQuery mobile's script and stylesheet files in the page.
<link rel="stylesheet" href="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.css" /> <script src="http://code.jquery.com/jquery-1.10.2.min.js"></script> <script src="http://code.jquery.com/mobile/1.3.2/jquery.mobile-1.3.2.min.js"></script>
2. Create a button to trigger the signature pad.
<div id="page" data-role="content"> <a href="#divPopUpSignContract" data-rel="popup" data-position-to="window" data-role="button" data-inline="true">Open</a> </div>
3. Create the html for the signature pad.
<div data-role="popup" id="divPopUpSignContract"> <div data-role="header" data-theme="b"> <a data-role="button" data-rel="back" data-transition="slide" class="ui-btn-right" onclick="closePopUp()"> Close </a> <p class="popupHeader">Sign Pad</p> </div> <div class="ui-content popUpHeight"> <div id="div_signcontract"> <canvas id="canvas">Canvas is not supported</canvas> <div> <input id="btnSubmitSign" type="button" data-inline="true" data-mini="true" data-theme="b" value="Submit Sign" onclick="fun_submit()" /> <input id="btnClearSign" type="button" data-inline="true" data-mini="true" data-theme="b" value="Clear" onclick="init_Sign_Canvas()" /> </div> </div> </div> </div>
4. The javascript.
<script type="text/javascript"> var isSign = false; var leftMButtonDown = false; jQuery(function(){ //Initialize sign pad init_Sign_Canvas(); }); function fun_submit() { if(isSign) { var canvas = $("#canvas").get(0); var imgData = canvas.toDataURL(); jQuery('#page').find('p').remove(); jQuery('#page').find('img').remove(); jQuery('#page').append(jQuery('<p>Your Sign:</p>')); jQuery('#page').append($('<img/>').attr('src',imgData)); closePopUp(); } else { alert('Please sign'); } } function closePopUp() { jQuery('#divPopUpSignContract').popup('close'); jQuery('#divPopUpSignContract').popup('close'); } function init_Sign_Canvas() { isSign = false; leftMButtonDown = false; //Set Canvas width var sizedWindowWidth =$('#div_signcontract').width(); if(sizedWindowWidth > 700) sizedWindowWidth = $(window).width() / 2; else if(sizedWindowWidth > 400) sizedWindowWidth = sizedWindowWidth - 50; else sizedWindowWidth = sizedWindowWidth - 20; $("#canvas").width(sizedWindowWidth); $("#canvas").height(200); $("#canvas").css("border","1px solid #000"); var canvas = $("#canvas").get(0); canvasContext = canvas.getContext('2d'); if(canvasContext) { canvasContext.canvas.width = sizedWindowWidth; canvasContext.canvas.height = 200; canvasContext.fillStyle = "#fff"; canvasContext.fillRect(0,0,sizedWindowWidth,200); canvasContext.moveTo(50,150); canvasContext.lineTo(sizedWindowWidth-50,150); canvasContext.stroke(); canvasContext.fillStyle = "#000"; canvasContext.font="20px Arial"; canvasContext.fillText("x",40,155); } $(canvas).on('vmousedown', function (e) { if(e.which === 1) { leftMButtonDown = true; canvasContext.fillStyle = "#000"; var x = e.pageX - $(e.target).offset().left; var y = e.pageY - $(e.target).offset().top; canvasContext.moveTo(x, y); } e.preventDefault(); return false; }); $(canvas).on('vmouseup', function (e) { if(leftMButtonDown && e.which === 1) { leftMButtonDown = false; isSign = true; } e.preventDefault(); return false; }); // draw a line from the last point to this one $(canvas).bind('vmousemove', function (e) { if(leftMButtonDown == true) { canvasContext.fillStyle = "#000"; var x = e.pageX - $(e.target).offset().left; var y = e.pageY - $(e.target).offset().top; canvasContext.lineTo(x,y); canvasContext.stroke(); } e.preventDefault(); return false; }); } </script>
Change log:
2014-06-03
- update.
This awesome jQuery plugin is developed by ndce06. For more Advanced Usages, please check the demo page or visit the official website.