jQuery Plugin For Touch & Drag Events - touch.js
File Size: | 19.1 KB |
---|---|
Views Total: | 8532 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
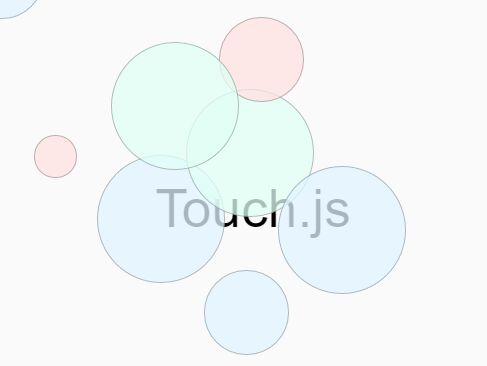
touch.js is a small (9kb minified) JavaScript library that provides additional touch gesture and drag/drop events for your cross-platform jQuery project.
Additional events supported:
- tap
- doubleTap
- tapAndHold
- dragStart
- drag
- dragEnd
- dragEnter
- dragOver
- dragLeave
- drop
- swipe
- swipeUp
- swipeDown
- swipeLeft
- swipeRight
Basic usage:
1. Download & load the minified version of the jQuery touch.js and we're ready to go.
<script src="//code.jquery.com/jquery.min.js"></script> <!--<script src="//code.jquery.com/jquery-3.1.1.min.js"></script>--> <script src="jquery.touch.js"></script>
2. Bind drag/drop/tap/swipe events as follows:
// Bind events. $. .touch({ // Turn on document tracking so stuff works even if the cursor leaves the trackpad. trackDocument: true, // Normalize coordinates when/if the cursor leaves the trackpad. trackDocumentNormalize: true, // Prevent default events for drag/swipe (so the page doesn't scroll when you do those gestures). preventDefault: { drag: true, swipe: true } }) // Tap events. .on('tap', function(e, o) { _name('tap'); _status('Pos: ' + Math.round(o.ex) + ', ' + Math.round(o.ey)); }) .on('doubleTap', function(e, o) { _name('doubleTap'); _status('Pos: ' + Math.round(o.ex) + ', ' + Math.round(o.ey)); }) .on('tapAndHold', function(e, o) { _name('tapAndHold'); _status('Pos: ' + Math.round(o.ex) + ', ' + Math.round(o.ey)); }) // Drag events. //.on('dragStart', function(e, o) { _name('dragStart'); _status('Pos: ' + o.ex + ', ' + o.ey); }) //.on('drag', function(e, o) { _name('drag'); _status('Pos: ' + o.ex + ', ' + o.ey); }) //.on('dragEnd', function(e, o) { _name('dragEnd'); _status('S: ' + o.start.ex + ', ' + o.start.ey + ', E: ' + o.end.ex + ', ' + o.end.ey + ', Dist: ' + Math.round(o.distance) + ', Dur: ' + o.duration + ', V: ' + Math.round(o.velocity)); }) // Swipe event. //.on('swipe', function(e, o) { _name('swipe'); _status('Dist: ' + o.distance + ', Dur: ' + o.duration + ', V: ' + Math.round(o.velocity)); }) // Swipe events. .on('swipeUp', function(e, o) { _name('swipeUp'); _status('Dist: ' + Math.round(o.distance) + ', Dur: ' + o.duration + ', V: ' + Math.round(o.velocity)); }) .on('swipeDown', function(e, o) { _name('swipeDown'); _status('Dist: ' + Math.round(o.distance) + ', Dur: ' + o.duration + ', V: ' + Math.round(o.velocity)); }) .on('swipeLeft', function(e, o) { _name('swipeLeft'); _status('Dist: ' + Math.round(o.distance) + ', Dur: ' + o.duration + ', V: ' + Math.round(o.velocity)); }) .on('swipeRight', function(e, o) { _name('swipeRight'); _status('Dist: ' + Math.round(o.distance) + ', Dur: ' + o.duration + ', V: ' + Math.round(o.velocity)); });
3. All default configuration options.
// If true, mouse clicks and movements will also trigger touch events. useMouse: true, // If true, certain events (like drag) can continue to track even if the mouse cursor leaves the originating element. trackDocument: false, // If true, when "trackDocument" is enabled, coordinates will be normalized to the confines of the originating element. trackDocumentNormalize: false, // Disables "click" event (prevents both "tap" and "click" firing on certain elements like <label>). noClick: false, // Distance from tap to register a drag (lower = more sensitive, higher = less sensitive). dragThreshold: 10, // Time to wait before registering a drag (needs to be high enough to not interfere with scrolling). dragDelay: 200, // Distance from tap to register a swipe (lower = more sensitive, higher = less sensitive). swipeThreshold: 30, // Delay between taps. tapDelay: 250, // Time to wait before triggering "tapAndHold". tapAndHoldDelay: 500, // If defined, delegates touch events to descendants matching this selector. delegateSelector: null, // Filters drop target elements. Can be any of the following: // - "selector" Target element must match this selector. // - function(element, target) { ... } Use boolean return value of a custom callback. // - true Target element must be a sibling of dragged element. // - false No filtering. dropFilter: false, // Prevent or allow default actions for certain event classes. Can be any of the following: // - true Prevent default actions for this event class. // - false Allow default actions for this event class. // - function(state) { ... } Use boolean return value of a custom callback (state = touch state object) preventDefault: { drag: false, swipe: false, tap: false }
Change log:
2017-03-03
- v1.1.0
This awesome jQuery plugin is developed by ajlkn. For more Advanced Usages, please check the demo page or visit the official website.