Simple Text Slider/Rotator with jQuery and CSS
File Size: | 2.58 KB |
---|---|
Views Total: | 108193 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
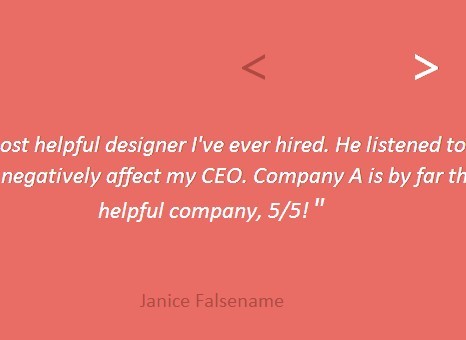
A jQuery based content carousel/slider which enables you to rotate a list of fix positioned text content with click or automatically.
How to use it:
1. Create prev/next links to navigate through your text content.
<div class="btn-bar"> <div id="buttons"> <a id="prev" href="#"><</a> <a id="next" href="#">></a> </div> </div>
2. Create a list of text content for the slider.
<div id="slides"> <ul> <li class="slide"> ... </li> <li class="slide"> ... </li> <li class="slide"> ... </li> </ul> </div>
3. The basic style rules for the slider.
#slides { overflow: hidden; position: relative; width: 100%; height: 250px; } #slides ul { list-style: none; width: 100%; height: 250px; margin: 0; padding: 0; position: relative; } #slides li { width: 100%; height: 250px; float: left; text-align: center; position: relative; font-family: lato, sans-serif; }
4. Styling for prev and next buttons.
.btn-bar { width: 60%; margin: 0 auto; display: block; position: relative; top: 40px; } #buttons { padding: 0 0 5px 0; float: right; } #buttons a { text-align: center; display: block; font-size: 50px; float: left; outline: 0; margin: 0 60px; color: #b14943; text-decoration: none; display: block; padding: 9px; width: 35px; } a#prev:hover, a#next:hover { color: #FFF; text-shadow: .5px 0px #b14943; }
5. The core JavaScript (jQuery) to enable the slider.
$(document).ready(function () { //rotation speed and timer var speed = 5000; var run = setInterval(rotate, speed); var slides = $('.slide'); var container = $('#slides ul'); var elm = container.find(':first-child').prop("tagName"); var item_width = container.width(); var previous = 'prev'; //id of previous button var next = 'next'; //id of next button slides.width(item_width); //set the slides to the correct pixel width container.parent().width(item_width); container.width(slides.length * item_width); //set the slides container to the correct total width container.find(elm + ':first').before(container.find(elm + ':last')); resetSlides(); //if user clicked on prev button $('#buttons a').click(function (e) { //slide the item if (container.is(':animated')) { return false; } if (e.target.id == previous) { container.stop().animate({ 'left': 0 }, 1500, function () { container.find(elm + ':first').before(container.find(elm + ':last')); resetSlides(); }); } if (e.target.id == next) { container.stop().animate({ 'left': item_width * -2 }, 1500, function () { container.find(elm + ':last').after(container.find(elm + ':first')); resetSlides(); }); } //cancel the link behavior return false; }); //if mouse hover, pause the auto rotation, otherwise rotate it container.parent().mouseenter(function () { clearInterval(run); }).mouseleave(function () { run = setInterval(rotate, speed); }); function resetSlides() { //and adjust the container so current is in the frame container.css({ 'left': -1 * item_width }); } }); //a simple function to click next link //a timer will call this function, and the rotation will begin function rotate() { $('#next').click(); }
This awesome jQuery plugin is developed by TyStelmach. For more Advanced Usages, please check the demo page or visit the official website.