jQuery Plugin For Adding Notes and Markers To An Image - imgNotes
File Size: | 804 KB |
---|---|
Views Total: | 36030 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
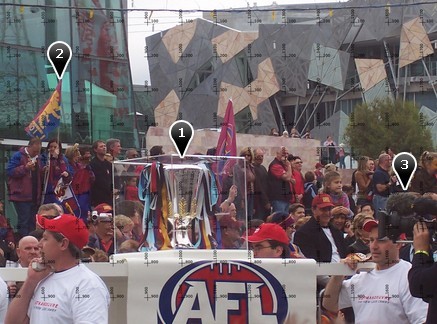
imgNotes an extension of the jQuery imgViewer plugin that adds markers and notes to an image that can be zoomed in and out with the mousewheel and panned around by click and drag. Methods are provided to import and export notes from and to a javascript array.
In edit mode (canEdit: true) the default action associated with clicking on the image is to insert a marker and open a dialog with a textarea to enter a note. The "Delete" button on this dialog deletes the marker and note. Callbacks are provided for developers to override the default marker and the note editor.
In view mode (canEdit: false) markers cannot be changed. By default, clicking on a marker displays the associated note in a dialog window. This default action can be overriden by a callback option.
Basic Usage:
1. Include necessary javascript files on your web page
<script type="text/javascript" src="../libs/jquery/jquery.js"></script> <script type="text/javascript" src="../libs/jquery/jquery-ui.js"></script> <script type="text/javascript" src="../libs/jquery.mousewheel.min.js"></script> <script type="text/javascript" src="../libs/imgViewer.js"></script> <script type="text/javascript" src="../src/imgNotes.js"></script>
2. Include required CSS files on the page
<link rel="stylesheet" href="http://code.jquery.com/ui/1.10.2/themes/smoothness/jquery-ui.css" media="screen">
3. Create a container for the image
<div id-"imgdiv"> <img id="image" src="../image/test_image.jpg" /> <br/> <button id="toggleEdit">Edit</button> <button id="export">Export</button> </div>
4. The CSS
.marker{ width: 27px; height: 40px; position: absolute; left: -13px; top: -35px; font-size: 12px; font-weight: bold; line-height: 25px; letter-spacing: -1px; text-align: center; color: #fff; } .marker.black{ background: url(marker_black.png); }
5. The javascript
<script type="text/javascript"> ;(function($) { $(document).ready(function() { var $img = $("#image").imgNotes(); $img.one("load",function(){ $img.imgNotes("import", [{x: "0.5", y:"0.5", note:"AFL Grand Final Trophy"}, {x: "0.322", y:"0.269", note: "Brisbane Lions Flag"}, {x: "0.824", y: "0.593", note: "Fluffy microphone"}]); }); var $toggle = $("#toggleEdit"); if ($img.imgNotes("option","canEdit")) { $toggle.text("View"); } else { $toggle.text("Edit"); } $toggle.on("click", function() { var $this = $(this); if ($this.text()=="Edit") { $this.text("View"); $img.imgNotes("option", "canEdit", true); } else { $this.text('Edit'); $img.imgNotes('option', 'canEdit', false); } }); }); })(jQuery); </script>
6. Available options.
// Get/Set the current zoom level of the image // must be >= 1 zoom: 1, // How much the zoom changes for each mousewheel click // must be a positive number zoomStep: 0.1, // Get/Set the limit on the maximum zoom level of the image zoomMax: 0 // Controls if image will be zoomable zoomable: true, // Controls if image will be dragable dragable: true, // Controls if notes can be added and edited canEdit: false, // Controls the vertical positioning of the marker // relative to the marker location A change only affects markers subsequently inserted vAll: "middle", // Controls the horizontal positioning of the marker // relative to the marker location A change only affects markers subsequently inserted hAll: "middle", // Callback triggered when a marker/note is added onAdd: function() {}, // Callback triggered when the widget is in edit mode onEdit: function() {}, // Callback triggered when the widget is in view mode onShow: function() {}, // Callback triggered when the markers are repainted onUpdateMarker: function() {}, // Callback triggered when the image view is repainted onUpdate: function() {},
7. API methods.
// Add a note to the image $("#image").imgNotes("addNote", 0.4,0.3,"Note <b>Text</b>"); // Get the number of notes in the widget $("#image").imgNotes("count"); // Delete all the notes from the widget $("#image").imgNotes("clear"); // Delete all the notes and removes the widget $("#image").imgNotes("destroy"); // Add notes from a javascript array to the widget $("#image1").imgNotes("import", [ {x: "0.5", y:"0.5", note:"AFL Grand Final Trophy"}, {x: "0.322", y:"0.269", note: "Brisbane Lions Flag"}, {x: "0.824", y: "0.593", note: "Fluffy microphone"} ]); // Export notes in the widget to a javascript array $("#image").imgNotes("export"); // Pan the view to be centred at the given relative image coordinates $("#image").imgNotes("panTo", 0.5,0.0);
More examples:
Change logs:
v1.0.0 (2017-03-15)
- update
v0.9.1 (2016-12-31)
- Add zoomMax option
v0.9.0 (2016-08-09)
- Add dragable option
v0.8.0 (2016-08-01)
- Update to imgViewer 0.8.0
v0.7.6 (2015-06-13)
- Fix bug affecting display of newly inserted markers and position of edit dialog
- Turn events off before removing note markers
v0.7.5 (2014-11-01)
- Add onUpdate callback
- Add onUpdate callback example that links the markers with a line
v0.7.4 (2014-08-05)
- Simplify signature for onUpdateMarker callback
- Fix call of onUpdateMarker in addNote method - fixes bug with markers not displayed on first load
v0.7.3 (2014-07-30)
- Add onUpdateMarker callback
- Add example with custom onUpdateMarker
- Fix so that zoom option value reflects zoom of underlying imgViewer
v0.7.2 (2014-07-22)
- Upgrade for imgViewer 0.7.2
- Fix dialog close function call
v0.7.1 (2014-06-22)
- update.
v0.7 (2014-06-19)
- Update to work with imgViewer 0.7
- Add zoomable option to disable zooming
- Updated Grunfile.js to include tests against latest version (2.1.0) of jQuery.
This awesome jQuery plugin is developed by waynegm. For more Advanced Usages, please check the demo page or visit the official website.