Creating A Togglable Bottom Content Panel Using jQuery
File Size: | 57.6 KB |
---|---|
Views Total: | 8136 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
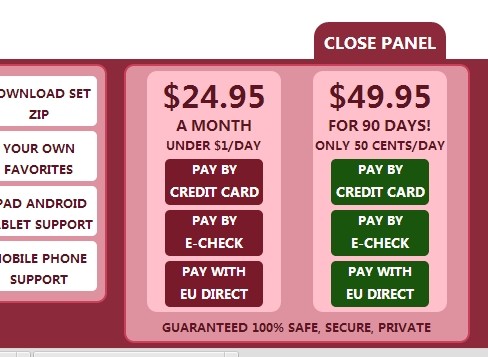
A tiny jQuery script for creating a sticky content panel that will pull out from the bottom of the web page when you click the panel tab.
How to use it:
1. Create the Html for a bottom panel.
<div class="panel-wrapper"> <div class="panel-controller"> <div class="tab-controller"> <span class="close">CLOSE PANEL</span> <span class="show">OPEN PANEL</span> </div> </div> <div class="panel-content"> Panel content goes here </div> </div>
2. The CSS rules to style the bottom panel and make it sticky at the bottom of the page.
.panel-wrapper * { -webkit-box-sizing: border-box; -moz-box-sizing: border-box; box-sizing: border-box; } .panel-wrapper { position: fixed; left: 0; bottom: 0; overflow: hidden; width: 100%; font-family: sans-serif; } .panel-controller { position: relative; overflow: hidden; width: 100%; } .tab-controller { float: right; margin-right: 50px; padding: 10px 10px 5px; background-color: #8C293B; -webkit-border-radius: 15px 15px 0 0; -moz-border-radius: 15px 15px 0 0; border-radius: 15px 15px 0 0; } .tab-controller * { display: block; font-family: sans-serif; font-size: 16px; font-weight: bold; color: white; cursor: pointer; } .tab-controller .show { display: none; } .panel-content { overflow: hidden; width: 100%; background-color: #8C293B; }
3. Include the jQuery javascript library at the bottom of the document so the pages load faster.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.0/jquery.min.js"></script>
4. The jQuery script to enable the toggable bottom panel.
(function($) { jQuery(document).ready(function() { Panel.init(); $(document).on('click', '.tab-controller', function() { Panel.togglePanel(); }); }); var Panel = { isVisible : true, showMessage : null, hideMessage : null, animationDuration : 650, animationEasing : 'linear', init : function() { }, hidePanel : function() { $('.panel-wrapper').animate({ bottom : -(Panel.getAnimationOffset()) }, Panel.animationDuration, Panel.animationEasing, function() { Panel.isVisible = false; Panel.updateTabMessage(); }); }, showPanel : function() { $('.panel-wrapper').animate({ bottom : 0 }, Panel.animationDuration, Panel.animationEasing, function() { Panel.isVisible = true; Panel.updateTabMessage(); }); }, togglePanel : function() { ((this.isVisible) ? this.hidePanel : this.showPanel)(); }, updateTabMessage : function() { if (this.isVisible) { $('.tab-controller .close').show(); $('.tab-controller .show').hide(); } else { $('.tab-controller .close').hide(); $('.tab-controller .show').show(); } }, getAnimationOffset : function() { return $('.panel-content').height(); } } })(jQuery);
This awesome jQuery plugin is developed by DojoGeekRA. For more Advanced Usages, please check the demo page or visit the official website.