jQuery Plugin for Tree Widget - jqTree
File Size: | 1.24 MB |
---|---|
Views Total: | 31577 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
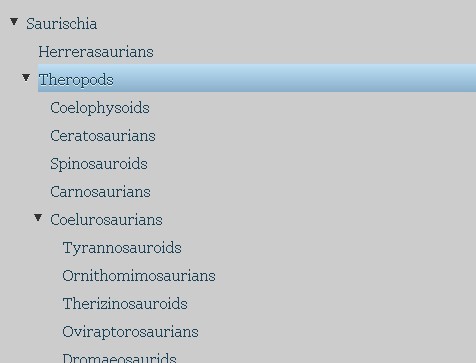
jqTree is a jQuery based Tree Widget that allows you to create folder tree from JSON data with some fancy animations.
Licensed under the Apache License 2.0.
Features:
- Load JSON data from local
- Load JSON data from server
- Drag and drop supported
- Keyboard interactions
- Single & Multiple Selection
- Auto scroll and auto escape supported
- Save state supported
- Lazy load for better performance
You might also like:
- Powerful and Multi-Functional jQuery Folder Tree Plugin - zTree
- jQuery Flat Folder Tree Plugin - simplefolders
How to use it:
1. Include jQuery library and the jqTree.js plugin's files in the HTML page.
<link rel="stylesheet" href="/path/to/jqtree.css" /> <script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/tree.jquery.js"></script>
2. Create a container to hold the tree view.
<div id="myTree"></div>
3. Prepare your data in JSON or in an array of objects as follows:
const data = [ { label: "node 1", id: 1, children: [{ label: "node 1-1", id: 2 }, { label: "node 1-2", id: 3 } // ... ] }, { label: "node2", id: 4, children: [{ label: "node 2-1", id: 5 }] } // ... ];
4. Visualize the data in a tree.
$("#myTree").tree({ data: data });
5. All default options to config the tree.
// Define the contents of the tree. data: data, // or Load the node data from this url. dataUrl: null, // Animation speed animationSpeed: "fast", // Open nodes initially. autoOpen: false, // Save and restore the state of the tree automatically. saveState: false, // Turn on dragging and dropping of nodes. dragAndDrop: false, // Turn on selection of nodes. selectable: true, // Bind the context menu event (true/false). useContextMenu: true, // callback functions. onCanSelectNode: null, onSetStateFromStorage: null, onGetStateFromStorage: null, onCreateLi: null, onIsMoveHandle: null, onCanMove: null, onCanMoveTo: null, onLoadFailed: null, onDragMove: null, onDragStop: null, onLoading: null // The position of the toggle button buttonLeft: true, // Shows empty folders showEmptyFolder: false, // The tabindex of the tree. tabIndex: 0 // Determine if text is autoescaped. autoEscape: true, // A character or symbol to display on closed nodes. closedIcon: '►', // A character or symbol to display on opened nodes. openedIcon: '▼', // Turn slide animation on or off. slide: true, // Node class nodeClass: Node, // Process the tree data from the server. dataFilter: null, // Enable or disable keyboard support. keyboardSupport: true, // Set the delay for opening a folder during drag-and-drop. openFolderDelay: 500, // rtl or ltr rtl: null, // sets the delay before drag-and-drop is starte startDndDelay: 300,
6. API methods.
// Add a new node as parent var newNode = $('#myTree').tree('getNodeByName', 'newNode'); $('#myTree').tree( 'addParentNode', { name: 'new_parent', id: 987 }, newNode ); // Add a new node after an existing node var nodeExample = $('#myTree').tree('getNodeByName', 'nodeExample'); $('#myTree').tree( 'addNodeAfter', { name: 'new_node', id: 456 }, nodeExample ); // Add a new node before an existing node var nodeExample = $('#myTree').tree('getNodeByName', 'nodeExample'); $('#myTree').tree( 'addNodeBefore', { name: 'new_node', id: 456 }, nodeExample ); // Add a node to parent node. var nodeExample = $('#myTree').tree('getNodeByName', 'nodeExample'); $('#myTree').tree( 'appendNode', { name: 'new_node', id: 456, children: [ { name: 'child1', id: 457 }, { name: 'child2', id: 458 } ] }, nodeExample ); // Add a root node $('#myTree').tree( 'appendNode', { name: 'new_node', id: 456 } ); // Prepend a node $('#myTree').tree( 'prependNode', { name: 'new_node', id: 456, children: [ { name: 'child1', id: 457 }, { name: 'child2', id: 458 } ] }, nodeExample ); // Collapse a node with or without animation $('#myTree').tree('closeNode', node, true/false); // Destroy the instance $('#myTree').tree('destroy'); // Get node information $('#myTree').tree('getNodeByCallback', function(node) { if (node.name == 'abc') { // Node is found; return true return true; } else { // Node not found; continue searching return false; } }); $('#myTree').tree('getNodeById', id); $('#myTree').tree('getNodeByHtmlElement', element); $('#myTree').tree('getSelectedNode'); $('#myTree').tree('getState'); $('#myTree').tree('getTree'); // Detect if is dragging $('#myTree').tree('isDragging'); // Load new data $('#myTree').tree('loadData', new_data); // Load a sub tree $('#myTree').tree('loadData', data, node); // Load data from an URL $('#myTree').tree('loadDataFromUrl', '/category/tree/'); $('#myTree').tree('loadDataFromUrl', '/category/tree/', node); $('#myTree').tree('loadDataFromUrl', '/category/tree/', null, function(){ // ... }); // Select the next/prev node $('#myTree').tree('moveDown'); $('#myTree').tree('moveUp'); // Move a node $('#myTree').tree('moveNode', node, target_node, 'after'); // Open a node $('#myTree').tree('openNode', node); $('#myTree').tree('openNode', node, slide(TRUE/FALSE)); $('#myTree').tree('openNode', node, slide(TRUE/FALSE)); $('#myTree').tree('openNode', node, slide(TRUE/FALSE), function(node){ // fired when finished }); // Reload data $('#myTree').tree('reload'); $('#myTree').tree('reload', function(){ // fired when loaded }); // Remove a node $('#myTree').tree('removeNode', node); // Select a node $('#myTree').tree('selectNode', node); $('#myTree').tree('selectNode', node, null); // deselect $('#myTree').tree('selectNode', node, { mustToggle, mustSetFocus }); // Scroll to a node $('#myTree').tree('scrollToNode', node); // Set options $('#myTree').tree('setOption', 'keyboardSupport', false); // Set state $('#myTree').tree('setState', state); // Expand/collapse a node $('#myTree').tree('toggle', node); $('#myTree').tree('toggle', node, slide(TRUE/FALSE)); // Convert data to JSON $('#myTree').tree('toJson'); // refresh $('#myTree').tree('refresh'); // Update data $('#myTree').tree( 'updateNode', node, { name: 'new name', id: 1, children: [ { name: 'child1', id: 2 } ] } );
7. Node functions.
// Add a node to the selection. var node = $('#myTree').tree('getNodeById', 123); $('#myTree').tree('addToSelection', node); // Return a list of selected nodes. var nodes = $('#myTree').tree('getSelectedNodes'); // Return if this node is selected. var node = $('#tree1').tree('getNodeById', 123); var isSelected = $('#tree1').tree('isNodeSelected', node); // Remove a node from the selection. var node = $('#tree1').tree('getNodeById', 123); $('#tree1').tree('removeFromSelection', node); // Get the subtree of a node. var node = $('#tree1').tree('getNodeById', 123); var data = node.getData(); // Get the level of a node. var node = $('#tree1').tree('getNodeById', 123); var level = node.getLevel(); // Get the next node in the tree. var node = node.getNextNode(); // Get the next sibling of a node. var node = node.getNextSibling(); // Return the previous node in the tree. var node = node.getPreviousNode(); // Get the previous sibling of this node. var node = node.getPreviousSibling(); // Get the previous visible node in the tree. var node = node.getPreviousVisibleNode(); // Access the parent of a node. var parentNode = node.parent; // Access the children of a node. for (var i=0; i < node.children.length; i++) { var child = node.children[i]; }
8. Event handlers.
$('#myTree').on('tree.click', function(event) { // click a node var node = event.node; alert(node.name); }); $('#myTree').on('tree.dblclick', function(event) { // double-click a node var node = event.node; alert(node.name); }); $('#myTree').on('tree.close', function(e) { // close a node console.log(e.node); }); $('#myTree').on('tree.contextmenu', function(event) { // right-click a node var node = event.node; alert(node.name); }); $('#myTree').on('tree.init', function() { // on init }); $('#myTree').on('tree.load_data', function(e) { // load data console.log(e.tree_data); }); $('#myTree').on('tree.loading_data', function(e) { // loading data console.log(e.isLoading, e.node, e.$el); }); $('#myTree').on('tree.move', function(event) { // move a node console.log('moved_node', event.move_info.moved_node); console.log('target_node', event.move_info.target_node); console.log('position', event.move_info.position); console.log('previous_parent', event.move_info.previous_parent); }); $('#myTree').on('tree.refresh', function(e) { // on refresh }); $('#myTree').on('tree.select', function(event) { if (event.node) { // node was selected var node = event.node; alert(node.name); } else { // event.node is null // a node was deselected // e.previous_node contains the deselected node } });
More Examples:
- Example 1 - load json data
- Example 2 - load json data from the server
- Example 3 - drag and drop
- Example 4 - save the state
- Example 5 - load nodes on demand from the server
- Example 6 - autoEscape
- Example 7 - autoscroll
Changelog:
1.8.4 (2024-07-20)
- update braces package to fix security issue
1.8.3 (2024-05-07)
- bugfixes
1.8.2 (2024-03-25)
- fix dnd regression
1.8.1 (2024-03-17)
- fix onCanMoveTo
1.8.0 (2023-12-27)
- Open parents in addToSelection
- This release drops support for very old browsers (like IE 11).
- Use a mouse handler
1.7.5 (2023-10-22)
- Update
1.7.4 (2023-09-25)
- Update
1.7.3 (2023-09-17)
- Update
1.7.2 (2023-09-15)
- improve types of closedIcon and openedIcon
1.7.1 (2023-08-07)
- Improve accessibility
1.7.0 (2022-12-24)
- dispatch the tree.load_data after initializing the tree
- Rename getPreviousNode to getPreviousVisibleNode and getNextNode to getNextVisibleNode.
- Add new getPreviousNode and getNextNode functions that ignores if a node is visible.
1.6.3 (2022-08-08)
- Bugfixes
1.6.2 (2021-12-16)
- Bugfixes
1.6.1 (2021-12-14)
- Add refresh method
- Update package
1.6.0 (2021-02-09)
- Improve performance of internal node lookup
- Changed: getNodeById doesn’t convert strings to numbers anymore
- Fix drag-and-drop on ie11
1.5.3 (2021-01-13)
- Add startDndDelay option
1.5.2 (2020-10-25)
- Update
1.5.1 (2020-09-24)
- Fix autoescape
1.5.0 (2020-09-07)
- Fix definition of onLoading
1.4.12 (2020-06-26)
- Improve performance of title rendering
1.4.12 (2019-11-11)
- updated
1.4.11 (2018-04-06)
- updated
1.4.11 (2018-04-06)
- updated
1.4.10 (2018-04-06)
- updated
1.4.8 (2018-07-24)
- update to the latest version.
1.4.7 (2018-06-16)
- update to the latest version.
1.4.6 (2018-05-08)
- update to the latest version.
1.4.5 (2018-03-15)
- update to the latest version.
1.4.4 (2017-12-21)
- update to the latest version.
0.18.0 (2013-09-17)
- Added 'custom html' example
- _getDataUrlInfo: add selected_node parameter
0.17.0 (2013-07-14)
- Fixed don't return from the click event prematurely when Ctrl is pressed
0.16.0 (2013-05-18)
- Fixed
_selectCurrentNode
method by removing @tree_widget
This awesome jQuery plugin is developed by mbraak. For more Advanced Usages, please check the demo page or visit the official website.