Responsive Expanding Column Layout with jQuery and CSS3
File Size: | 2.98 KB |
---|---|
Views Total: | 3867 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
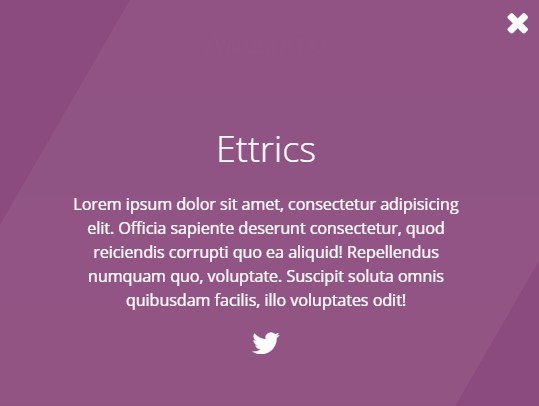
An Html5/CSS3/jQuery powered responsive column layout that will expand to a fullscreen modal-style content panel once you clicked on a child item.
How to use it:
1. Build the Html structure for the column layout.
<section class="strips"> <article class="strips__strip"> <div class="strip__content"> <h1 class="strip__title" data-name="column__1">Column 1</h1> <div class="strip__inner-text"> <h2>Section 1</h2> <p>Content 1</p> </div> </div> </article> <article class="strips__strip"> <div class="strip__content"> <h1 class="strip__title" data-name="column__2">Column 2</h1> <div class="strip__inner-text"> <h2>Section 2</h2> <p>Content 2</p> </div> </div> </article> <article class="strips__strip"> <div class="strip__content"> <h1 class="strip__title" data-name="column__3">Column 3</h1> <div class="strip__inner-text"> <h2>Section 3</h2> <p>Content 3</p> </div> </div> </article> <article class="strips__strip"> <div class="strip__content"> <h1 class="strip__title" data-name="column__4">Column 4</h1> <div class="strip__inner-text"> <h2>Section 4</h2> <p>Content 4</p> </div> </div> </article> <article class="strips__strip"> <div class="strip__content"> <h1 class="strip__title" data-name="column__5">Column 5</h1> <div class="strip__inner-text"> <h2>Section 5</h2> <p>Content 5</p> </div> </div> </article> <i class="fa fa-close strip__close"></i> </section>
2. Include the Font Awesome for the close icon (OPtional).
<link href="http://netdna.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
3. The primary CSS/CSS3 styles.
* { box-sizing: border-box; } .strips { min-height: 100vh; text-align: center; overflow: hidden; color: white; } .strips__strip { will-change: width, left, z-index, height; position: absolute; width: 20%; min-height: 100vh; overflow: hidden; cursor: pointer; -webkit-transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); } .strips__strip:nth-child(1) { left: 0; } .strips__strip:nth-child(2) { left: 20vw; } .strips__strip:nth-child(3) { left: 40vw; } .strips__strip:nth-child(4) { left: 60vw; } .strips__strip:nth-child(5) { left: 80vw; } .strips__strip:nth-child(1) .strip__content { background: #244F75; -webkit-transform: translate3d(-100%, 0, 0); transform: translate3d(-100%, 0, 0); -webkit-animation-name: strip1; animation-name: strip1; -webkit-animation-delay: 0.1s; animation-delay: 0.1s; } .strips__strip:nth-child(2) .strip__content { background: #60BFBF; -webkit-transform: translate3d(0, 100%, 0); transform: translate3d(0, 100%, 0); -webkit-animation-name: strip2; animation-name: strip2; -webkit-animation-delay: 0.2s; animation-delay: 0.2s; } .strips__strip:nth-child(3) .strip__content { background: #8C4B7E; -webkit-transform: translate3d(0, -100%, 0); transform: translate3d(0, -100%, 0); -webkit-animation-name: strip3; animation-name: strip3; -webkit-animation-delay: 0.3s; animation-delay: 0.3s; } .strips__strip:nth-child(4) .strip__content { background: #F8BB44; -webkit-transform: translate3d(0, 100%, 0); transform: translate3d(0, 100%, 0); -webkit-animation-name: strip4; animation-name: strip4; -webkit-animation-delay: 0.4s; animation-delay: 0.4s; } .strips__strip:nth-child(5) .strip__content { background: #F24B4B; -webkit-transform: translate3d(100%, 0, 0); transform: translate3d(100%, 0, 0); -webkit-animation-name: strip5; animation-name: strip5; -webkit-animation-delay: 0.5s; animation-delay: 0.5s; } .strips .strip__content { -webkit-animation-duration: 1s; animation-duration: 1s; -webkit-animation-timing-function: cubic-bezier(0.23, 1, 0.32, 1); animation-timing-function: cubic-bezier(0.23, 1, 0.32, 1); -webkit-animation-fill-mode: both; animation-fill-mode: both; display: -webkit-box; display: -webkit-flex; display: -ms-flexbox; display: flex; -webkit-box-align: center; -webkit-align-items: center; -ms-flex-align: center; align-items: center; -webkit-box-pack: center; -webkit-justify-content: center; -ms-flex-pack: center; justify-content: center; position: absolute; top: 0; left: 0; width: 100%; height: 100%; text-decoration: none; } .strips .strip__content:hover:before { -webkit-transform: skew(-30deg) scale(3) translate(0, 0); -ms-transform: skew(-30deg) scale(3) translate(0, 0); transform: skew(-30deg) scale(3) translate(0, 0); opacity: 0.1; } .strips .strip__content:before { content: ""; position: absolute; z-index: 1; top: 0; left: 0; width: 100%; height: 100%; background: white; opacity: 0.05; -webkit-transform-origin: center center; -ms-transform-origin: center center; transform-origin: center center; -webkit-transform: skew(-30deg) scaleY(1) translate(0, 0); -ms-transform: skew(-30deg) scaleY(1) translate(0, 0); transform: skew(-30deg) scaleY(1) translate(0, 0); -webkit-transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); } .strips .strip__inner-text { will-change: transform, opacity; position: absolute; z-index: 5; top: 50%; left: 50%; width: 70%; -webkit-transform: translate(-50%, -50%) scale(0.5); -ms-transform: translate(-50%, -50%) scale(0.5); transform: translate(-50%, -50%) scale(0.5); opacity: 0; -webkit-transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); } .strips__strip--expanded { width: 100%; top: 0 !important; left: 0 !important; z-index: 3; cursor: default; } .strips__strip--expanded .strip__title { opacity: 0; } .strips__strip--expanded .strip__inner-text { opacity: 1; -webkit-transform: translate(-50%, -50%) scale(1); -ms-transform: translate(-50%, -50%) scale(1); transform: translate(-50%, -50%) scale(1); } .strip__title { display: block; margin: 0; position: relative; z-index: 2; width: 100%; font-size: 3.5vw; color: white; -webkit-transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); } .strip__close { position: absolute; right: 3vw; top: 3vw; opacity: 0; z-index: 10; -webkit-transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); transition: all 0.6s cubic-bezier(0.23, 1, 0.32, 1); cursor: pointer; -webkit-transition-delay: 0.5s; transition-delay: 0.5s; } .strip__close--show { opacity: 1; }
4. The CSS media queries for smaller screen devices.
@media screen and (max-width: 760px) { .strips__strip { min-height: 20vh; } .strips__strip:nth-child(1) { top: 0; left: 0; width: 100%; } .strips__strip:nth-child(2) { top: 20vh; left: 0; width: 100%; } .strips__strip:nth-child(3) { top: 40vh; left: 0; width: 100%; } .strips__strip:nth-child(4) { top: 60vh; left: 0; width: 100%; } .strips__strip:nth-child(5) { top: 80vh; left: 0; width: 100%; } } @media screen and (max-width: 760px) { .strips__strip--expanded { min-height: 100vh; } } .strips__strip--expanded .strip__content:hover:before { -webkit-transform: skew(-30deg) scale(1) translate(0, 0); -ms-transform: skew(-30deg) scale(1) translate(0, 0); transform: skew(-30deg) scale(1) translate(0, 0); opacity: 0.05; } @media screen and (max-width: 760px) { .strip__title { font-size: 28px; } }
5. Create the strip animations.
@-webkit-keyframes strip1 { 0% { -webkit-transform: translate3d(-100%, 0, 0); transform: translate3d(-100%, 0, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } } @keyframes strip1 { 0% { -webkit-transform: translate3d(-100%, 0, 0); transform: translate3d(-100%, 0, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } } @-webkit-keyframes strip2 { 0% { -webkit-transform: translate3d(0, 100%, 0); transform: translate3d(0, 100%, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } } @keyframes strip2 { 0% { -webkit-transform: translate3d(0, 100%, 0); transform: translate3d(0, 100%, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } } @-webkit-keyframes strip3 { 0% { -webkit-transform: translate3d(0, -100%, 0); transform: translate3d(0, -100%, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } } @keyframes strip3 { 0% { -webkit-transform: translate3d(0, -100%, 0); transform: translate3d(0, -100%, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } } @-webkit-keyframes strip4 { 0% { -webkit-transform: translate3d(0, 100%, 0); transform: translate3d(0, 100%, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } } @keyframes strip4 { 0% { -webkit-transform: translate3d(0, 100%, 0); transform: translate3d(0, 100%, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } } @-webkit-keyframes strip5 { 0% { -webkit-transform: translate3d(100%, 0, 0); transform: translate3d(100%, 0, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } } @keyframes strip5 { 0% { -webkit-transform: translate3d(100%, 0, 0); transform: translate3d(100%, 0, 0); } 100% { -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } }
6. Load the latest version of jQuery library and insert the following JavaScript snippet before the closing body tag.
var Expand = (function() { var tile = $('.strips__strip'); var tileLink = $('.strips__strip > .strip__content'); var tileText = tileLink.find('.strip__inner-text'); var stripClose = $('.strip__close'); var expanded = false; var open = function() { var tile = $(this).parent(); if (!expanded) { tile.addClass('strips__strip--expanded'); // add delay to inner text tileText.css('transition', 'all .6s 1s cubic-bezier(0.23, 1, 0.32, 1)'); stripClose.addClass('strip__close--show'); stripClose.css('transition', 'all .6s 1s cubic-bezier(0.23, 1, 0.32, 1)'); expanded = true; } }; var close = function() { if (expanded) { tile.removeClass('strips__strip--expanded'); // remove delay from inner text tileText.css('transition', 'all 0.15s 0 cubic-bezier(0.23, 1, 0.32, 1)'); stripClose.removeClass('strip__close--show'); stripClose.css('transition', 'all 0.2s 0s cubic-bezier(0.23, 1, 0.32, 1)') expanded = false; } } var bindActions = function() { tileLink.on('click', open); stripClose.on('click', close); }; var init = function() { bindActions(); }; return { init: init }; }()); Expand.init();
This awesome jQuery plugin is developed by ettrics. For more Advanced Usages, please check the demo page or visit the official website.