Button Selection Interaction With jQuery & CSS3
File Size: | 6.62 KB |
---|---|
Views Total: | 865 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
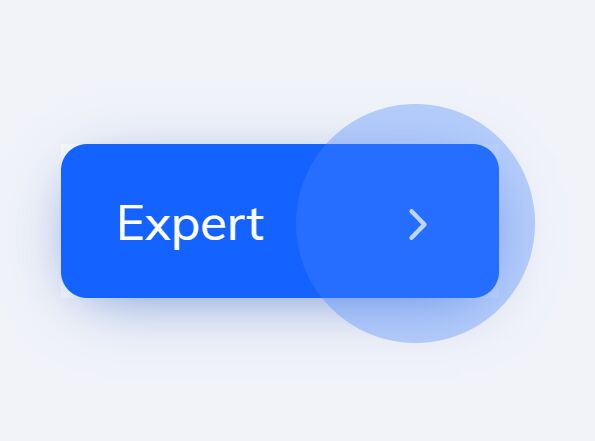
An animated button selection UI design concept that allows the user to switch between options by clicking/tapping the button.
See It In Action:
See the Pen UI // Button Selection Type by Cosimo Scarpa (@coswise) on CodePen.
How to use it:
1. Create the HTML for the button selection UI.
<div class="concept-wrap" id="mainWrap"> <div class="tap"> <div class="wave"></div> </div> <div class="btn-wrap" id="btn"> <div class="btn-cnt" id="content"> <div class="cnt cnt-1"><p class="text">jQuery</p> <i class="icon">Any Arrow Icon Here</i> </div> <div class="cnt cnt-2"><p class="text">Script</p> <i class="icon">Any Arrow Icon Here</i> </div> <div class="cnt cnt-3"><p class="text">Net</p> <i class="icon">Any Arrow Icon Here</i> </div> <div class="cnt cnt-4"><p class="text">Example</p> <i class="icon">Any Arrow Icon Here</i> </div> </div> </div> </div>
2. The necessary CSS/CSS3 styles for the button selection interaction.
.cnt, .btn-cnt { display: flex; justify-content: center; align-items: center; } .cnt, .btn-wrap, .concept-wrap { width: 200px; height: 70px; } .concept-wrap { position: relative; cursor: pointer; } .btn-wrap { cursor: pointer; position: absolute; background: #1462ff; border-radius: 12px; box-shadow: 0 6px 30px -10px #4a74c9; overflow: hidden; transform: translateX(0); } .btn-cnt { position: absolute; top: 0; right: 0; flex-direction: row; height: 70px; width: 800px; z-index: 9; } .cnt { justify-content: space-around; box-sizing: border-box; padding: 0 25px; } .cnt .text { text-align: left; font-size: 1.4em; margin-right: auto; color: #fff; } .cnt .icon { margin-top: 3px; color: #fff; } .tap { width: 140px; height: 140px; position: absolute; background: transparent; opacity: 0.4; top: calc(50% - 69px); right: -32px; border-radius: 50%; z-index: 4; display: flex; justify-content: center; align-items: center; z-index: 3; } .wave { width: 30px; height: 30px; background: #4784ff; border-radius: 50%; opacity: 0; } .wave-act { animation: t-wave 599ms cubic-bezier(0.77, 0.06, 0, 0.99) forwards; } @keyframes t-wave { 0% { width: 50px; height: 50px; opacity: 0; } 15% { opacity: 1; } 20% { width: 40px; height: 40px; } 50% { opacity: 0.9; } 80% { opacity: 0; } 100% { opacity: 0; width: 140px; height: 140px; } }
3. Load the needed jQuery, jquery.transit, and jquery.bez libraries in the document.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/cdn/jquery.transit.min.js"></script> <script src="/path/to/cdn/jquery.bez.js"></script>
4. The JavaScript (jQuery script) to enable the button selection interaction.
// Variables var btnSz = 200; var c = 0; var bezierEasing = 'cubic-bezier(.69,-0.49,0,1)'; var t1 = 299; var t2 = 1198; var bZ2 = 'cubic-bezier(.46,.56,0,.88)' $('#mainWrap').on('click', function() { c++; console.log(c); // Lock $('.lock').addClass('lock-on'); setTimeout(function() { $('.lock').removeClass('lock-on'); }, 1200); // Wave $('.wave').addClass('wave-act'); setTimeout(function() { $('.wave').removeClass('wave-act'); }, 1000); // Move Button setTimeout(function() { $('#btn').transition({ x: '+=10px' }, 289, bZ2, function() { $('#btn').transition({ x: '0px' }, 289, bZ2); }); }, 399); // Move Content setTimeout(function() { if (c <= 2) { $('#content').transition({ x: '+=220px' }, t1, bezierEasing, function() { $('#content').transition({ x: '-=20' }, 699, 'cubic-bezier(.25,.49,.2,1)'); }); } else if (c == 3) { $('#content').transition({ x: '+=220px' }, t1, bezierEasing, function() { $('#content').transition({ x: '-=20' }, 699, 'cubic-bezier(.25,.49,.2,1)'); }); setTimeout(function() { $('.cnt-1').css('order', '4'); $('#content').transition({ x: '0' }, 0); }, t2); } else if (c == 4) { $('#content').transition({ x: '+=220px' }, t1, bezierEasing, function() { $('#content').transition({ x: '-=20' }, 699, 'cubic-bezier(.25,.49,.2,1)'); }); setTimeout(function() { $('#content').transition({ x: '0' }, 0); $('.cnt-1').css('order', '0'); }, t2); c = 0; } }, 399) });
This awesome jQuery plugin is developed by coswise. For more Advanced Usages, please check the demo page or visit the official website.