Create Frame Animations In jQuery - Clockz.js
File Size: | 653 KB |
---|---|
Views Total: | 579 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
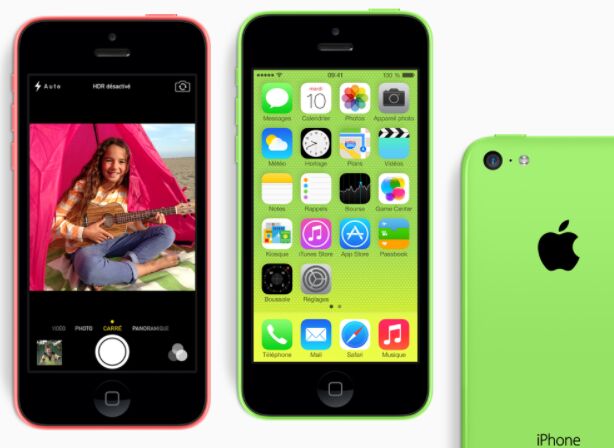
Clockz.js is a jQuery plugin to create smooth frame-by-frame animations using CSS transitions and transforms.
Each frame has its own CSS properties, duration, and easing function. Supports 3rd animation engines like rstacruz's jquery.transit plugin and greensock library.
How to use it:
1. Load the jQuery Clockz.js after jQuery and jQuery.transit (OPTIONAL).
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/cdn/transit.min.js"></script> <script src="js/clockz.js"></script>
2. Define your frame animations for each element.
<div class="element1"></div> <div class="element2"></div> <div class="element3"></div>
var element1 = { name: 'element1', loop: true, frames: [ { duration: 2000 }, { properties: { left: 768 }, duration: 800, easing: 'swing' }, { properties: { top: 200 }, duration: 3000, easing: 'swing' }, { properties: { perspective: '100px', rotateX: '180deg'}, type: 'transit', duration: 2000 }, { duration: 2000 }, { properties: { top: 10 }, duration: 3000, easing: 'swing' }, { properties: { perspective: '100px', rotateX: '0deg'}, type: 'transit', duration: 2000 } ] }; var element2 = { name: 'element2', loop: true, frames: [ { duration: 2000 }, { properties: { left: 410 }, duration: 800, easing: 'swing' }, { properties: { rotate: '+=30' }, type: 'transit', duration: 70, easing: 'swing' }, { properties: { rotate: '+=30' }, type: 'transit', duration: 70, easing: 'swing' }, { properties: { rotate: '+=30' }, type: 'transit', duration: 70, easing: 'swing' }, { properties: { rotate: '+=30' }, type: 'transit', duration: 70, easing: 'swing' }, { properties: { rotate: '+=30' }, type: 'transit', duration: 70, easing: 'swing' }, { properties: { rotate: '+=30' }, type: 'transit', duration: 70, easing: 'swing' }, { properties: { top: 600 }, duration: 2000, easing: 'swing' }, { duration: 1000 }, { properties: { top: 10 }, duration: 2000, easing: 'swing' } ] }; var element3 = { name: 'element3', loop: true, loopStart: 0, loopEnd: 3, frames: [ { duration: 2000 }, { properties: { left: 1111 }, duration: 800, easing: 'swing' }, { properties: { top: 600 }, duration: 1000, easing: 'swing' }, { properties: { top: 10 }, duration: 1000, easing: 'swing' } ] };
3. Initialize the Clockz.js and activate the frame animation using the playHook
.
// initialize var clockz = new Clockz(); // playhood clockz.playHook = function(node, frame, callback) { var duration = frame.duration || 400; var properties = frame.properties || null; if (properties) { var jnode = $(node); if (!jnode) { this.error( 'playHook(): node is invalid.' ); return; } var type = frame.type || 'jquery'; var easing = frame.easing || 'swing'; switch (type) { case 'jquery': $(node).animate(properties, duration, easing, callback); break; case 'transit': $(node).transition($.extend({ duration: duration, easing: easing, complete: callback }, properties)); break; } } else { setTimeout(callback, duration); } }; // play all clockz.create('.element1', element1); clockz.create('.element2', element2); clockz.create('.element3', element3); clockz.playAll();
This awesome jQuery plugin is developed by oOthkOo. For more Advanced Usages, please check the demo page or visit the official website.