Folded Accordion-style Carousel With jQuery
File Size: | 2.62 KB |
---|---|
Views Total: | 1470 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
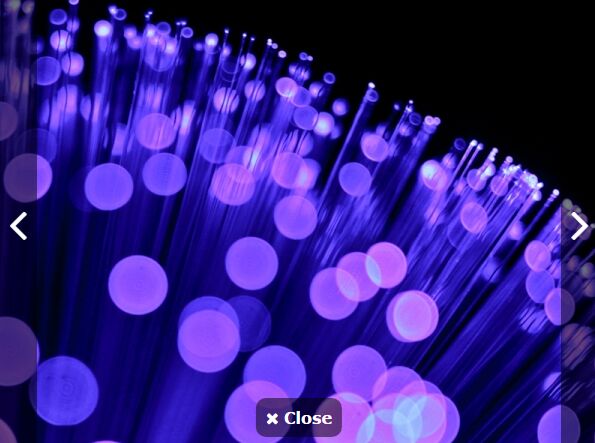
A folded accordion style photo gallery that expands the selected image into a full-size carousel with arrow navigation and collapse button.
How to use it:
1. Create an html list of images for the carousel.
<ul id="jsi-slider-container" class="slider-container"> <li><a href="javascript:void(0);"><img src="1.jpg" /></a></li> <li><a href="javascript:void(0);"><img src="2.jpg" /></a></li> <li><a href="javascript:void(0);"><img src="3.jpg" /></a></li> <li><a href="javascript:void(0);"><img src="4.jpg" /></a></li> <li><a href="javascript:void(0);"><img src="5.jpg" /></a></li> <li><a href="javascript:void(0);"><img src="6.jpg" /></a></li> ... </ul>
2. Add the navigation arrows & close button to the carousel.
<div id="jsi-close-trigger-container" class="close-trigger-container"><a href="javascript:void(0);">Close</a></div> <div id="jsi-previous-trigger-container" class="move-trigger-container previous"><a href="javascript:void(0);"><</a></div> <!-- Carousel Here --> <div id="jsi-next-trigger-container" class="move-trigger-container next"><a href="javascript:void(0);">></a></div>
3. Copy and paste the following CSS styles to your webpage.
.slider-container { position: absolute; margin: 0; padding: 0; list-style: none; } .slider-container > li { float: left; overflow: hidden; width: 100px; height: 100%; } .move-trigger-container { display: none; position: absolute; top: 0; height: 100%; padding: 0; z-index: 10; background-color: rgba(0, 0, 0, 0.5); } .move-trigger-container > a { position: relative; display: inline-block; padding: 10px; top: 50%; transform: translateY(-50%); font-size: 50px; color: #FFFFFF; text-decoration: none; } .previous { left: 0; } .next { right: 0; } .close-trigger-container { position: absolute; display: none; bottom: 10px; left: 50%; transform: translateX(-50%); font-size: 16px; font-weight: bold; z-index: 10; background-color: rgba(0, 0, 0, 0.5); border-radius: 10px; } .close-trigger-container > a { display: inline-block; width: 100%; height: 100%; padding: 10px; color: #FFFFFF; text-decoration: none; }
4. Place the latest version of the jQuery JavaScript library at the bottom of the page.
<script src="//code.jquery.com/jquery-3.2.1.min.js"></script>
5. The main function for the carousel.
var SLIDER_CONTROLLER = { ADJUST_TIME : 600, SLIDE_TIME : 600, init : function(){ this.setParameters(); this.bindEvent(); }, setParameters : function(){ this.$container = $('#jsi-slider-container'); this.$cassettes = this.$container.children('li'); this.$previousTriggerContainer = $('#jsi-previous-trigger-container'); this.$previous = this.$previousTriggerContainer.children('a'); this.$nextTriggerContainer = $('#jsi-next-trigger-container'); this.$next = this.$nextTriggerContainer.children('a'); this.$closeTriggerContainer = $('#jsi-close-trigger-container'); this.$close = this.$closeTriggerContainer.children('a'); this.initContainerWidth = this.$container.outerWidth(); this.initCassetteWidth = this.$cassettes.outerWidth(); this.cassetteCount = this.$cassettes.length; this.status = 0; this.currentIndex = 0; }, bindEvent : function(){ var myself = this; this.$cassettes.each(function(index){ var $cassette = $(this); $cassette.on('click', myself.extendCassetes.bind(myself, $cassette, index)); }); this.$close.on('click', this.shortenCassettes.bind(this)); this.$previous.on('click', this.goToPrevious.bind(this)); this.$next.on('click', this.goToNext.bind(this)); }, extendCassetes : function($cassette, index){ if(this.$container.is(':animated') || this.status == 1){ return; } $cassette.animate({width : this.initContainerWidth}, this.ADJUST_TIME); this.$container.animate( {width : this.initContainerWidth + (this.initContainerWidth - this.initCassetteWidth), left : -this.initCassetteWidth * index}, this.ADJUST_TIME, this.finishToExtendCassettes.bind(this, index) ); }, shortenCassettes : function(){ if(this.$container.is(':animated') || this.status == 0){ return; } this.$container .width(this.initContainerWidth + this.initCassetteWidth * (this.cassetteCount - 1)) .css('left', -this.currentIndex * this.initCassetteWidth) .animate( {width : this.initContainerWidth, left : 0}, this.ADJUST_TIME, this.finishToShortenCassettes.bind(this) ); this.$cassettes.width(this.initCassetteWidth); this.$cassettes.eq(this.currentIndex).width(this.initContainerWidth).animate({width : this.initCassetteWidth}, this.ADJUST_TIME); this.$previousTriggerContainer.fadeOut(); this.$nextTriggerContainer.fadeOut(); this.$closeTriggerContainer.fadeOut(); }, finishToExtendCassettes : function(index){ this.$container .width(this.initContainerWidth * this.cassetteCount) .css('left', -index * this.initContainerWidth); this.$cassettes.width(this.initContainerWidth); this.status = 1; this.currentIndex = index; if(this.currentIndex != 0){ this.$previousTriggerContainer.fadeIn(); } if(this.currentIndex != this.cassetteCount - 1){ this.$nextTriggerContainer.fadeIn(); } this.$closeTriggerContainer.fadeIn(); }, finishToShortenCassettes : function(){ this.status = 0; }, goToPrevious : function(){ if(this.currentIndex == 0 || this.$container.is(':animated')){ return; } this.currentIndex--; this.moveContainer(); }, goToNext : function(){ if(this.currentIndex == this.cassetteCount - 1 || this.$container.is(':animated')){ return; } this.currentIndex++; this.moveContainer(); }, moveContainer : function(){ this.$container.animate({left : -this.currentIndex * this.initContainerWidth}, this.SLIDE_TIME); if(this.currentIndex == 0){ this.$previousTriggerContainer.fadeOut(); }else{ this.$previousTriggerContainer.fadeIn(); } if(this.currentIndex == this.cassetteCount - 1){ this.$nextTriggerContainer.fadeOut(); }else{ this.$nextTriggerContainer.fadeIn(); } } };
6. Initialize the carousel on page load.
$(window).on('load', function(){ SLIDER_CONTROLLER.init(); });
This awesome jQuery plugin is developed by K-T. For more Advanced Usages, please check the demo page or visit the official website.