Responsive and Flexible Mobile Touch Slider - Swiper
File Size: | 1.49 MB |
---|---|
Views Total: | 283359 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
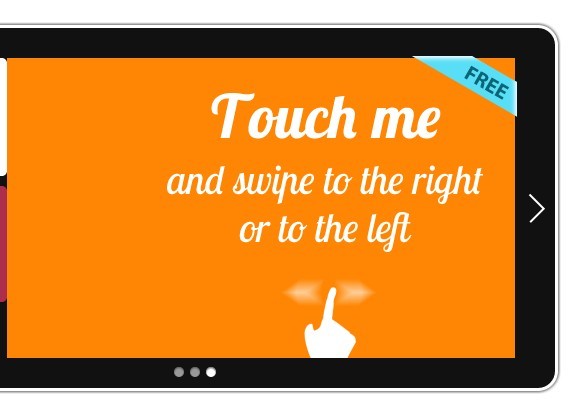
Swiper is a powerful and modular javascript library to implement responsive, accessible, flexible, touch-enabled carouses/sliders on your mobile websites and apps. Can be used as a jQuery plugin.
Features:
- Responsive design
- Small and fast
- Fully accessible
- Supports any html elements, not only images
- Supports Vertical/Horizontal animations and swipes
- Cool transition effects: Fade, Flip, 3D Cube, 3D Coverflow, Parallax
- Hash navigation
- Grid layout
- Image lazy load
- Virtual Slides for better performance
- Keyboard interactions
- RTL layout
- Works on iOS, Android and latest Desktop browsers
- Available for Angular, React, Vue, and Svelte.
View more:
Table Of Contents:
Basic Usage (v7+):
1. Install the package with NPM.
# NPM $ npm i swiper --save
2. Import the Swiper and modules of your choice as an ES module into your project. All available modules:
- a11y
- autoplay
- controller
- effect-cards
- effect-coverflow
- effect-creative
- effect-cube
- effect-fade
- effect-flip
- free-mode
- grid
- hash-navigation
- history
- keyboard
- lazy
- manipulation
- mousewheel
- navigation
- pagination
- parallax
- scrollbar
- thumbs
- virtual
- zoom
// Core import Swiper from 'swiper'; import 'swiper/css'; // With OPTIONAL modules import Swiper, { Navigation, ... } from 'swiper'; import 'swiper/css'; import 'swiper/css/navigation'; ... Swiper.use([Navigation, ...]);
3. Or load the necessary JavaScript and CSS files from a CDN.
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper/swiper-bundle.min.css" /> <script src="https://cdn.jsdelivr.net/npm/swiper/swiper-bundle.min.js"></script> <!-- OR --> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper/swiper-bundle.min.css" /> <script type="module"> import Swiper from 'https://cdn.jsdelivr.net/npm/swiper/swiper-bundle.esm.browser.min.js' </script>
4. Add slides to the swiper.
<div class="swiper"> <div class="swiper-wrapper"> <div class="swiper-slide">Slide 1</div> <div class="swiper-slide">Slide 2</div> <div class="swiper-slide">Slide 3</div> <div class="swiper-slide">Slide 4</div> ... </div> </div>
5. Initialize the swiper and done.
const swiper = new Swiper('.swiper', { // options here });
6. Add pagination & navigation controls to the swiper if needed.
<div class="swiper"> <div class="swiper-wrapper"> <div class="swiper-slide">Slide 1</div> <div class="swiper-slide">Slide 2</div> <div class="swiper-slide">Slide 3</div> <div class="swiper-slide">Slide 4</div> ... </div> <div class="swiper-pagination"></div> <div class="swiper-button-prev"></div> <div class="swiper-button-next"></div> <div class="swiper-scrollbar"></div> </div>
const swiper = new Swiper('.swiper', { pagination: { el: '.swiper-pagination', }, navigation: { nextEl: '.swiper-button-next', prevEl: '.swiper-button-prev', }, scrollbar: { el: '.swiper-scrollbar', } });
7. This is a full list of availble configurations that can be used to customize your swiper.
var swiper = new Swiper('.swiper-container',{ // auto init the intance init: true, // 'horizontal' or 'vertical' direction: 'horizontal', // target element to listen touch events on. touchEventsTarget: 'container', // initial slide index initialSlide: 0, // Inject text styles to the shadow DOM. Only for usage with Swiper Element injectStyles: '' // Inject styles <link>s to the shadow DOM. Only for usage with Swiper Element injectStylesUrls: '', // Number of next and previous slides to preload. Only applicable if using lazy loading. lazyPreloadPrevNext: 0, // CSS class name of lazy preloader lazyPreloaderClass string 'swiper-lazy-preloader', // animation speed speed: 300, // whether to use modern CSS Scroll Snap API. cssMode: false, // auto update on window resize updateOnWindowResize: true, // Overrides width: null, height: null, // allow to change slides by swiping or navigation/pagination buttons during transition preventInteractionOnTransition: false, // for ssr userAgent: null, url: null, // To support iOS's swipe-to-go-back gesture (when being used in-app). edgeSwipeDetection: false, edgeSwipeThreshold: 20, // Free mode // If true then slides will not have fixed positions freeMode: false, freeModeMomentum: true, freeModeMomentumRatio: 1, freeModeMomentumBounce: true, freeModeMomentumBounceRatio: 1, freeModeMomentumVelocityRatio: 1, freeModeSticky: false, freeModeMinimumVelocity: 0.02, // Autoheight autoHeight: false, // Set wrapper width setWrapperSize: false, // Virtual Translate virtualTranslate: false, // slide' or 'fade' or 'cube' or 'coverflow' or 'flip' effect: 'slide', // Breakpoints /* eg breakpoints: { // when window width is >= 320px 320: { slidesPerView: 2, spaceBetween: 20 }, // when window width is >= 480px 480: { slidesPerView: 3, spaceBetween: 30 }, // when window width is >= 640px 640: { slidesPerView: 4, spaceBetween: 40 } } */ breakpoints: undefined, // Slides grid spaceBetween: 0, slidesPerView: 1, slidesPerColumn: 1, slidesPerColumnFill: 'column', slidesPerGroup: 1, slidesPerGroupSkip: 0, centeredSlides: false, centeredSlidesBounds: false, slidesOffsetBefore: 0, // in px slidesOffsetAfter: 0, // in px normalizeSlideIndex: true, centerInsufficientSlides: false, // Disable swiper and hide navigation when container not overflow watchOverflow: false, // Round length roundLengths: false, // Options for touch events touchRatio: 1, touchAngle: 45, simulateTouch: true, shortSwipes: true, longSwipes: true, longSwipesRatio: 0.5, longSwipesMs: 300, followFinger: true, allowTouchMove: true, threshold: 0, touchMoveStopPropagation: false, touchStartPreventDefault: true, touchStartForcePreventDefault: false, touchReleaseOnEdges: false, // Unique Navigation Elements uniqueNavElements: true, // Resistance resistance: true, resistanceRatio: 0.85, // Use ResizeObserver (if supported by browser) on swiper container to detect container resize (instead of watching for window resize) resizeObserver: false, // Progress watchSlidesProgress: false, watchSlidesVisibility: false, // Cursor grabCursor: false, // Clicks preventClicks: true, preventClicksPropagation: true, slideToClickedSlide: false, // When enabled, will swipe slides only forward (one-way) regardless of swipe direction oneWayMovement: true, // Images preloadImages: true, updateOnImagesReady: true, // loop loop: false, loopAdditionalSlides: 0, loopedSlides: null, loopPreventsSliding: true, loopFillGroupWithBlank: false, loopPreventsSlide: true, // Swiping/no swiping allowSlidePrev: true, allowSlideNext: true, swipeHandler: null, // '.swipe-handler', noSwiping: true, noSwipingClass: 'swiper-no-swiping', noSwipingSelector: null, // Passive Listeners passiveListeners: true, // Default classes containerModifierClass: 'swiper-container-', slideClass: 'swiper-slide', slideBlankClass: 'swiper-slide-invisible-blank', slideActiveClass: 'swiper-slide-active', slideDuplicateActiveClass: 'swiper-slide-duplicate-active', slideVisibleClass: 'swiper-slide-visible', slideDuplicateClass: 'swiper-slide-duplicate', slideNextClass: 'swiper-slide-next', slideDuplicateNextClass: 'swiper-slide-duplicate-next', slidePrevClass: 'swiper-slide-prev', slideDuplicatePrevClass: 'swiper-slide-duplicate-prev', wrapperClass: 'swiper-wrapper', // Callbacks runCallbacksOnInit: true });
8. API methods.
// go to next slide at a given speed mySwiper.slideNext(speed, runCallbacks); // back to previous slide at a given speed mySwiper.slidePrev(speed, runCallbacks); // slide to a specific slide at a given speed mySwiper.slideTo(index, speed, runCallbacks); // Does the same as .slideTo but for the case when used with enabled loop. mySwiper.slideToLoop(index, speed, runCallbacks); // Reset swiper position to currently active slide mySwiper.slideReset(speed, runCallbacks); // Reset swiper position to closest slide/snap point mySwiper.slideToClosest(speed, runCallbacks); // Update height mySwiper.updateAutoHeight(speed); // Update swiper mySwiper.update(); // Changes slider direction from horizontal to vertical and back. mySwiper.changeDirection(direction); // update size mySwiper.updateSize() ; // update slides mySwiper.updateSlides(); // update progress mySwiper.updateProgress(); // update classes mySwiper.updateSlidesClasses(); // detach all event listeners mySwiper.detachEvents(); // attach all event listeners mySwiper.attachEvents(); // destroy the swiper instance mySwiper.destroy(deleteInstance, cleanStyles); // append new slides to the slider mySwiper.appendSlide(slides); // prepend new slides to the slider mySwiper.prependSlide(slides); // add new slides mySwiper.addSlide(index, slides); // remove a slide mySwiper.removeSlide(slideIndex); // remove all slides mySwiper.removeAllSlides(); // set custom CSS transforms mySwiper.setTranslate(translate); // animate custom css3 transform's translate value for swiper wrapper mySwiper.translateTo(translate, speed, runCallbacks, translateBounds); // get the current transform value mySwiper.getTranslate(); // add event listener mySwiper.on(event, handler); // that will be executed only once mySwiper.once(event, handler); // remove event listener for specified event mySwiper.off(event, handler); // remove all listeners mySwiper.off(event); // unset grab cursor mySwiper.unsetGrabCursor(); // set grab cursor mySwiper.setGrabCursor();
9. Event listeners.
mySwiper.on('beforeDestroy', function(){ // do something }) mySwiper.on('slideChange', function(){ // do something }) mySwiper.on('slideChangeTransitionStart', function(){ // do something }) mySwiper.on('slideChangeTransitionEnd', function(){ // do something }) mySwiper.on('slideNextTransitionStart', function(){ // do something }) mySwiper.on('slideNextTransitionEnd', function(){ // do something }) mySwiper.on('slidePrevTransitionStart', function(){ // do something }) mySwiper.on('slidePrevTransitionEnd', function(){ // do something }) mySwiper.on('transitionStart', function(){ // do something }) mySwiper.on('transitionEnd', function(){ // do something }) mySwiper.on('imagesReady', function(){ // do something }) mySwiper.on('reachBeginning', function(){ // do something }) mySwiper.on('reachEnd', function(){ // do something }) mySwiper.on('fromEdge', function(){ // do something }) mySwiper.on('toEdge', function(){ // do something }) mySwiper.on('resize', function(){ // do something }) mySwiper.on('observerUpdate', function(){ // do something }) mySwiper.on('touchStart', function(e){ // do something }) mySwiper.on('touchMove', function(e){ // do something }) mySwiper.on('touchMoveOpposite', function(e){ // do something }) mySwiper.on('sliderMove', function(e){ // do something }) mySwiper.on('touchEnd', function(e){ // do something }) mySwiper.on('click', function(e){ // do something }) mySwiper.on('tap', function(e){ // do something }) mySwiper.on('doubleTap', function(e){ // do something }) mySwiper.on('progress', function(progress){ // do something }) mySwiper.on('setTranslate', function(translate){ // do something }) mySwiper.on('setTransition', function(transition){ // do something }) mySwiper.on('hashChange', function(){ // do something }) mySwiper.on('hashSet', function(){ // do something }) mySwiper.on('beforeLoopFix', function(){ // do something }) mySwiper.on('loopFix', function(){ // do something }) mySwiper.on('breakpoint', function(){ // do something })
10. Available Props.
mySwiper.params mySwiper.$el mySwiper.$wrapperEl mySwiper.slides mySwiper.width mySwiper.height mySwiper.translate mySwiper.progress mySwiper.activeIndex mySwiper.realIndex mySwiper.previousIndex mySwiper.isBeginning mySwiper.isEnd mySwiper.animating mySwiper.touches mySwiper.touches.startX mySwiper.touches.startY mySwiper.touches.currentX mySwiper.touches.currentY mySwiper.touches.diff mySwiper.clickedIndex mySwiper.clickedSlide mySwiper.allowSlideNext mySwiper.allowSlidePrev mySwiper.allowTouchMove
11. Check out the Full API for more information.
Basic Usage (v5):
1. Download the Swiper v5 and then Include jQuery library (OPTIONAL) and the Swiper.js on your webpage
<script src="jquery.min.js"></script> <script src="swiper.min.js"></script>
2. Include required stylesheet on the page.
<link rel="stylesheet" href="swiper.min.css">
3. Create the html for the swiper.
<div class="swiper-container"> <div class="swiper-wrapper"> <!--First Slide--> <div class="swiper-slide"> <!-- Any HTML content of the first slide goes here --> </div> <!--Second Slide--> <div class="swiper-slide"> <!-- Any HTML content of the second slide goes here --> </div> <!--Third Slide--> <div class="swiper-slide"> <!-- Any HTML content of the third slide goes here --> </div> <!--Etc..--> </div> </div>
4. Initialize the swiper with optional settings.
var mySwiper = new Swiper ('.swiper-container', { // options here })
Changelog:
v11.1.1 (2024-04-10)
- zoom: fix zoom pan not preventing slide changes using touch
v11.1.0 (2024-03-28)
- bugfixes
v11.0.7 (2024-02-28)
- add swiper-effect-utils
- zoom: add ability to constrain max zoom to 100% of original image size
- bugfix
v11.0.6 (2024-02-05)
- bugfix
- core: prevent running .slideTo methods when Swiper is destroyed
v11.0.5 (2023-11-22)
- core: new slidesUpdated event
- Bug Fixes
v11.0.4 (2023-11-09)
- effectx: fix Safari issue with rotates even to 90deg
v11.0.3 (2023-10-26)
- bugfixes
v11.0.2 (2023-10-25)
- bugfixes
v11.0.0 (2023-10-24)
- core: add fully visible slides classes
- core: add handling for native touch events
- core: loop support for grid, new loopAddBlankSlides parameter
- core: remove loopedSlides parameter, add loopAdditionalSlides parameter
- core: reworked loop mode
- core: update loop mode logic and lowered requirements
- element: makeeventPrefix parameter default to swiper
- core: move default container overflow back to hidden
- bugfixes
v10.3.1 (2023-09-30)
- Bugfix
v10.3.0 (2023-09-21)
- core: allow createElements to process object params with {enabled: true}
- core: make slidesPerViewDynamic public
- bugfixes
v10.2.0 (2023-08-17)
- pagination: allow multiple clickableClass
v10.1.0 (2023-08-09)
- bugfixes
- element: support slides as slots
- types: make VirtualOptions generic
v10.0.4 (2023-07-09)
- bugfix
v10.0.3 (2023-07-04)
- added overflow:hidden for fallback if clip is not supported in target…
- element: use usual <style> tag if adopted stylesheet are not supported
v10.0.2 (2023-07-03)
- tweak browserslist to iOS >= 15
- rework package to use .mjs files and all scripts and styles are minified
- fully rework scripts structure in package
- rename package files .esm.js to .mjs
- browser ES modules
- change swiper container overflow to clip
- element: attributes can accept JSON stringified strings
- element: highly reworked Swiper web component
- navigation arrows use SVGs instead of font
- changed shadow DOM layout to have <div class="swiper"> inside
- component styles now added using adoptedStylesheets
- no more global styles injection
- set transform 3d on wrapper for iOS devices
- tweak types exports to be Node 16+ compatible
- bugfixes
v9.4.1 (2023-06-14)
- Bugfixes
v9.4.0 (2023-06-12)
- core: cssMode now supports freeMode
- element: add part="bullet[-active]" to pagination bullets
- bugfixes
v9.3.2 (2023-05-15)
- add string type for effect param
- mousewheel: add noMousewheelClass param
- mousewheel: support for swiper-no-mousewheel ignore class
- react: export SwiperClass type from 'swiper/react'
- Bugfixes
v9.3.1 (2023-05-10)
- element: added all events arguments in TS declarations
- element: correct extending of HTMLElementEventMap in types
v9.3.0 (2023-05-08)
- element: element events types
- element: add shadow parts
- element: more complex ts definitions
- bugfix
v9.2.4 (2023-04-21)
- Bugfixes
- hash-navigation: new getSlideIndex to specify slide index by hash
v9.2.3 (2023-04-18)
- Bugfixes
v9.2.1 (2023-04-14)
- virtual: patch for very large sliders using virtual slides
- bugfixes
v9.2.0 (2023-03-31)
- lazyPreloadPrevNext option to preload prev/next images
- zoom: highly improve pinch-zoom gestures handling
v9.1.0 (2023-03-01)
- Bugfixes
v9.0.5 (2023-02-14)
- Bugfixes
v9.0.4 (2023-02-10)
- Bugfixes
v9.0.3 (2023-02-06)
- Bugfixes
- history: allow empty string key
v9.0.2 (2023-02-04)
- Bugfixes
v9.0.1 (2023-02-02)
- Bugfixes
v9.0.0 (2023-02-01)
- add wrapperClass to swiper-wrapper in React & Vue components
- core: add loopPreventsSliding parameter
- core: new oneWayMovement parameter
- navigation: more CSS variables to control appearance and position
- pagination: more CSS variables to control appearance and position
- scrollbar: more CSS variables to control appearance
- add element core version
- export element css styles
- injectStyles and injectStylesUrls params
- parallax: support parallax rotate
- zoom: in method now accepts custom zoom ratio
- core: add loopedSlides parameter
- element: add option to avoid styles injecting
- thumbs: init thumbs on their appearance in DOM
- element: add standalone styles
- controller: support updated loop
- controller: support updated loop
- controller: support updated loop
- core: "fix" loop based on touch move direction
- element: add CSS styles for modules for Swiper Element
- core: add --swiper-wrapper-transition-timing-function CSS var
- navigation: more CSS vars
- paginatrion: more CSS vars
- scrollbar: more CSS vars
- virtual: renderSlide to support slide outer HTML and HTML element
- core: support for "swiper-slide-transform" element for better effects
- compatability with CSS mode
- core: remove Dom7
- core: don't prevent slidePrev/Next when animating in loop mode
- autoplay: correct support for virtual slides delay + fix for stopping autoplay on
- click
- element: support for comples parameters via attrs in a form of autoplay-delay
- virtual: support for DOM virtual slides
- virtual: support loop mode with virtual slides
- autoplay: all new Autoplay module
- core: remove unused slide*DuplicateClass parameters
- core: all new loop mode without slides duplication
- remove postinstall script
- core, zoom: rework touch handling logic to PointerEvents only
- core: make threshold parameter default to 5
- lazy: simplify Lazy module in favor of native loading="lazy"
- move new Lazy module to the Core
- react: add boolean lazy prop to SwiperSlide to render lazy prelaoder
- remove Images loading functionality: preloadImages, updateOnImagesReady
- vue: add boolean lazy prop to SwiperSlide to render lazy prelaoder
- add new Swiper Custom Element
- remove Angular and SolidJS components
- remove Swiper Svelte components
- Bug Fixes
v8.4.7 (2023-01-30)
- Bugfixes
v8.4.5 (2022-10-12)
- add direct JS/CSS core and bundle exports
v8.4.1/2 (2022-09-15)
- Bug Fixes
v8.4.0 (2022-09-14)
- Bug Fixes
- cards-effect: add perSlideRotate and perSlideOffset parameters
- core: new loopedSlidesLimit parameter to re-duplicate slides
- react: Allow SwiperSlide children as long as displayName includes SwiperSlide
- solid: keep solid components incompiled
v8.3.1 (2022-07-14)
- Bugfixes
v8.2.6 (2022-07-01)
- core: add changeLanguageDirection method to change it to RTL/LTR after init
- navigation: add navigationPrev and navigationNext events
- bugfixed
v8.2.4 (2022-06-13)
- Bugfix for Angular 13
v8.2.3 (2022-06-10)
- a11y: allow slideLabelMessage: null
- angular: update to angular v14
- scrollbar: add directional classes to scrollbar container
v8.1.4 (2022-04-24)
- Bug Fixes
v8.1.3 (2022-04-20)
- Bug Fixes
v8.1.2 (2022-04-20)
- Bug Fixes
- cube-effect: set --swiper-cube-translate-z CSS property on swiper-wrapper
v8.1.1 (2022-04-16)
- lazy: fix lazy preloader in iOS 15
- virtual: fix leaked effects translate when Virtual enabled
v8.1.0 (2022-04-11)
- a11y: add id parameter
- angular: support [ngClass] in swiperSlide
- effect-cards: add rotate parameter
- bugfixes
v8.0.7 (2022-03-04)
- bugfixes
- effect-cards: support for use with Virtual Slides
- virtual: better support for other effects rather than slide
v8.0.6 (2022-02-14)
- bugfixes
v8.0.5 (2022-02-10)
- core: fix short swipes
v8.0.4 (2022-02-10)
- angular: events types
- angular: lock output
- core: allow nesting of styles in SASS
- core: rewind on swipes
- bugfix
v8.0.3 (2022-02-05)
- bugfixes
v8.0.2 (2022-02-03)
- core: fix slider freeze with enabled observer
v8.0.1 (2022-02-02)
- angular: fix angular export
- react: types for useSwiper and useSwiperSlide hooks
- angular: remove deprecated index property
v8.0.0 (2022-02-01)
- a11y: automatically switch slides on focus (Tab) in inactive slides
- angular: update to angular 13 and enable tsconfig strict
- autoplay: autoplayPause and autoplayResume events
- free-mode: stop scrolling when touch event happens in freeMode
- react: useSwiper and useSwiperSlide context hooks
- svelte: swiper and swiperSlide context
- svelte: import index
- virtual: support rewind functionality with Virtual slides
- vue: useSwiper and useSwiperSlide context hooks
- new maxBackfaceHiddenSlides param to prevent flicker in Safari
- new maxBackfaceHiddenSlides param to prevent flicker in Safari
- svelte: types for Swiper slot virtualData
- svelte: types for SwiperSlide slot data
- vue: provide - inject swiper and swiperSlide context
- update dom7 to latest
- bugfixes
v7.4.1 (2021-12-25)
- Bugs fixed
v7.4.0 (2021-12-24)
- Bugs fixed
v7.3.4 (2021-12-22)
- fix(angular): fix nativeElement check
- Added "svelte": "./swiper.esm.js"
- fix(angular): setElement checks
- refactor(core/slide/slideTo): remove duplicate code
v7.3.3 (2021-12-16)
- Bug Fixes
v7.3.2 (2021-12-13)
- fix(angular): toggle input
- feat: update dom7 and ssr-window to latest
- feat(a11y): add aria-current to current bullet
v7.3.1 (2021-12-09)
- angular: support on params
- Fixed can not get clicked slider in shadow root issue
v7.3.0 (2021-11-20)
- react: export SwiperProps and SwiperSlideProps types
- Bugfixes
v7.2.0 (2021-10-27)
- add "main" and "module" package fields
- update dom7 and ssr-window to latest
v7.1.0 (2021-10-25)
- Bug Fixes
- core: add support to loop with slotted elements
v7.0.8 (2021-10-18)
- bugfixes
v7.0.8 (2021-10-04)
- virtual: improve behavior with cssMode
v7.0.7 (2021-09-29)
- Bug Fixes
v7.0.6 (2021-09-20)
- Bug Fixes
- angular: enabled prop
- angular: support data-swiper-autoplay
v7.0.5 (2021-09-10)
- Bug Fixes
v7.0.4 (2021-09-09)
- bugfix
- pagination: more pagination bullet CSS variables
v7.0.3 (2021-09-06)
- bugfix
v7.0.1 (2021-08-26)
- autoplay: add missing .start() method
- pagination: check for bullets before destroy
v7.0.0 (2021-08-25)
- Refactor and Bug Fixes
v6.8.2 (2021-08-20)
- Bug Fixes
v6.8.1 (2021-08-06)
- Bug Fixes
- pagination: hide 1 dot pagination
v6.8.0 (2021-07-23)
- Bug Fixes
v6.7.1 (2021-06-30)
- core: add ParallaxOptions types
- core: allow wrapperClass to be multiple classes
- core: make focusableElements configurable
- core: use window.matchMedia to detect window width for breakpoints
- bugfixes
v6.7.0 (2021-05-31)
- core: starter html layout can be optional with new createElements: true parameter
- autoplay: if disableOnInteraction and pauseOnMouseEnter, it will stop autoplay on interaction
- vue: add support for use Swiper as async component
- bugfixes
v6.6.2 (2021-05-23)
- core: fix breakpoints enabled detection
v6.6.1 (2021-05-12)
- core: fix breakpoints enabled detection
v6.6.0 (2021-05-12)
- core: make autoHeight work with Virtual slides
- zoom: don't toggle zoom on slides without zoom-container
- autoplay: new pauseOnMouseEnter parameter to pause autoplay on mouse enter over container
- core: new parameters and methods to enable/disable Swiper dynamically
- react: add the missing render function type
v6.5.5 (2021-04-09)
- Bug Fixes
v6.5.4 (2021-04-06)
- Bug Fixes
- a11y.slideLabelMessage
- custom html element support
- navigation: set disabled prop on nav element if it is a <button> element
v6.5.0 (2021-03-06)
- components: added "resizeObserver" boolean option/prop to enable ResizeObserver
- core: added support to use ResizeObserver with new "resizeObserver" parameter
- core: possible to enable breakpoints based on container width (instead of window width)
- Bugs fixed
v6.4.15 (2021-02-27)
- Bug Fixes
v6.3.1 - v6.3.5 (2020-11-06)
- Bugs fixed
v6.2.0 (2020-09-04)
- A11y: Added new parameters containerMessage, containerRoleDescriptionMessage and itemRoleDescriptionMessage
- React & Vue components updated
v6.2.0 (2020-09-04)
- All new Swiper Vue.js (v3) components
v6.1.3 (2020-09-03)
- Pagination: Now it won't set a11y attributes on customly rendered bullets
v6.0.0 - v6.1.2 (2020-08-19)
- All scripts transpiled to ES5
v5.4.1 (2020-05-26)
- Fixed dependencies versions
v5.4.0 (2020-05-21)
- Added hashChange and hashSet events
- Added support for <picture> lazy loading
- Potentially improved vertical scrolling issues on Windows/Linux OS
- Minor fixes
v5.3.7 (2020-04-15)
- Fixed cssMode behavior in RTL layout
- Fixed issue with not working double-tap to toggle with virtual slides
v5.3.7 (2020-02-29)
- Fixed wrong auto height calculation with centeredSlides enabled
- Now it will update auto height (if enabled) on lazy image loaded
- Fixed issue when previously active slide could be zoomed with zoom.in() API
- Fixed issue when zoom didn't work on <picture> element
- Added support for custom zoom-target element by adding swiper-zoom-target class to such elements
- stretch parameter now can be set in %
v5.3.1 (2020-02-09)
- New slidesPerGroupSkip behavior
v5.3.0 (2020-01-15)
- New slidesPerGroupSkip behavior
- New ratio-based breakpoints
- Added SCSS interpolation
- Mousehweel: Fixed issue when it can fail on load
- Minor fixes
v5.2.1 (2019-11-18)
- New loop events beforeLoopFix and loopFix
- New parameter updateOnWindowResize (by default true) that will update/recalc swiper on window resize/orientationchange
- Added SCSS interpolation for --swiper-theme-color variable when not building from source
- Quote SCSS color names
- Fixed issue when .once could be called more than once
- Fixed scroll wheel unwanted frozen effect
- New multipleActiveThumbs (by default true) option to control whether multiple thumbnail slides may get activated or not.
- Minor fixes
v5.2.0 (2019-10-26)
- New centeredSlidesBounds parameter that when enabled will keep first and last slides at bounds
- Fixed issue when freeMode could break position on resize
- Fixed transitin duration issue with freeModeSticky
- Fixed issue with wrong row/column if not full groups
- Fixed issue when watchOverflow and slidesOffsetBefore/slidesOffsetAfter couldn't work together
- Faster & smoother mousewheel inertial scrolling
- Added source maps to package builds
- Added minified version of browser.esm.bundle
- Minor fixes
v5.1.0 (2019-10-16)
- Fixed issues with touch on iOS 13
- New translateTo method
- Improved dynamic bullets behavior when loop: true
- Fixed issue with pinch to zoom on Android
- Minor fixes
v5.0.4 (2019-09-30)
- Now on short swipes over navigation buttons, it will treat it like nav button click
- Fixed issue when passing float slidesPerView could break loop mode
- Fixed issue with wrong "pointer" position calculation on scroll bar tap
- Fixed issue when it was paused after returning from hidden tab
- Minor fixes
v5.0.3 (2019-09-19)
- touchEventsTarget defaults back to container
- Added handling of touchcancel event
- Fixed issue with wrong order calculation in slidesPerColumnFill: 'row' mode
- Fixed issue with slides missplacing when prepending slides in virutal mode
- Fixed issue when zoomed image still swiped to another slide on mobiles
v5.0.0 (2019-09-19)
- All new CSS Scroll Snap mode (can be enabled with cssMode: true). It doesn't support all of Swiper's features, but potentially should bring a much better performance in simple configurations
- Fully removed Internet Explorer support
- breakpointsInverse parameter has been removed and now breakpoints behave like with breakpointsInverse: true before.
- touchMoveStopPropagation parameter now defaults to false
- click event won't be fired with 300ms delay anymore. Now it will be fired at the same time as tap event
- When slidesPerColumnFill: 'column' it now uses flex-direction: column layout which requires specified height on swiper-container
- touchEventsTarget now defaults to wrapper (rather than container like before)
- slidesPerColumn now can be used with breakpoints
- Now Swiper styles use CSS Custom Properties (CSS Custom Variables) to specify swiper's color theme (color of navigation buttons/pagination). It is now --swiper-theme-color: #007aff;
- Improved es module "tree-shake-ability"
- New swiper.esm.browser.bundle.js package that can be used directly in browser (import Swiper from 'swiper.esm.browser.bundle.js')
- Now it will be paused when document becomes hidden (in not active tab) and continued again when document becomes visible
- Swiper preloader image replaced with a little bit simpler loader. Now its color can be changed with --swiper-preloader-color CSS custom property (which is defaults to --swiper-theme-color)
- Active pagination bullets and pagination theme colors now use CSS Custom Properties. It can be defined with --swiper-pagination-color property (which is defaults to --swiper-theme-color)
- Navigation icons reworked with built-in (base64) icon font. It allows to apply any color and size without replacing image
- Navigation buttons colors now use CSS Custom Properties. It can be defined with --swiper-navigation-color property (which is defaults to --swiper-theme-color)
- With --swiper-navigation-size (defaults to 44px) it is now possible to change size of the navigation buttons (and icons)
- Minor fixes and improvements
This awesome jQuery plugin is developed by nolimits4web. For more Advanced Usages, please check the demo page or visit the official website.