Fully Responsive & Flexible jQuery Carousel Plugin - slick
File Size: | 53.8 KB |
---|---|
Views Total: | 539420 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
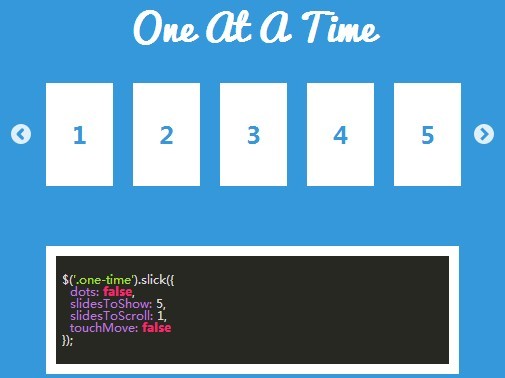
Slick is a feature-rich jQuery slider plugin for creating highly customizable, fully responsive, and mobile friendly carousels/sliders that work with any html elements.
Features:
- Fully responsive. Works with any devices.
- Uses CSS3 when available. Fully functional when not.
- Swipe enabled. Or disabled, if you prefer.
- Infinite looping.
- Horizontal & vertical scrolling.
- Lazy load & responsive image delivery.
- Autoplay, dots, arrows, callbacks, etc...
See Also:
How to use it:
1. Include the core and theme CSS in the head section of the web page.
<link rel="stylesheet" href="/path/to/slick.css"> <link rel="stylesheet" href="/path/to/slick-theme.css">
2. Wrap the Html contents you want to slide in DIV elements.
<div class="slider demo"> <div> Slide 1 </div> <div> Slide 2 </div> <div> Slide 3 </div> ... </div>
3. Include latest version of jQuery library and slick.js at the bottom of the page.
<script src="/path/to/jquery.min.js"></script> <script src="/path/to/slick.js"></script>
4. Call the plugin on the carousel wrapper you just created.
$(document).ready(function(){ $('.demo').slick(); });
5. Available options to customize the carousel.
$('.demo').slick({ // Enables tabbing and arrow key navigation accessibility: true, // Adapts slider height to the current slide adaptiveHeight: false, // Change where the navigation arrows are attached (Selector, htmlString, Array, Element, jQuery object) appendArrows: $(element), // Change where the navigation dots are attached (Selector, htmlString, Array, Element, jQuery object) appendDots: $(element), // Enable Next/Prev arrows arrows: true, // Sets the slider to be the navigation of other slider (Class or ID Name) asNavFor: null, // prev arrow prevArrow: '<button type="button" data-role="none" class="slick-prev">Previous</button>', // next arrow nextArrow: '<button type="button" data-role="none" class="slick-next">Next</button>', // Enables auto play of slides autoplay: false, // Auto play change interval autoplaySpeed: 3000, // Enables centered view with partial prev/next slides. // Use with odd numbered slidesToShow counts. centerMode: false, // Side padding when in center mode. (px or %) centerPadding: '50px', // CSS3 easing cssEase: 'ease', // Custom paging templates. customPaging: function(slider, i) { return '<button type="button" data-role="none">' + (i + 1) + '</button>'; }, // Current slide indicator dots dots: false, // Class for slide indicator dots container dotsClass: 'slick-dots', // Enables desktop dragging draggable: true, // animate() fallback easing easing: 'linear', // Resistance when swiping edges of non-infinite carousels edgeFriction: 0.35, // Enables fade fade: false, // Focus on select and/or change focusOnSelect: false, focusOnChange: false, // Infinite looping infinite: true, // Initial slide initialSlide: 0, // Accepts 'ondemand' or 'progressive' for lazy load technique lazyLoad: 'ondemand', // Mobile first mobileFirst: false, // Pauses autoplay on hover pauseOnHover: true, // Pauses autoplay on focus pauseOnFocus: true, // Pauses autoplay when a dot is hovered pauseOnDotsHover: false, // Target containet to respond to respondTo: 'window', // Breakpoint triggered settings /* eg responsive: [{ breakpoint: 1024, settings: { slidesToShow: 3, infinite: true } }, { breakpoint: 600, settings: { slidesToShow: 2, dots: true } }, { breakpoint: 300, settings: "unslick" // destroys slick }] */ responsive: null, // Setting this to more than 1 initializes grid mode. // Use slidesPerRow to set how many slides should be in each row. rows: 1, // Change the slider's direction to become right-to-left rtl: false, // Slide element query slide: '', // # of slides to show at a time slidesToShow: 1, // With grid mode intialized via the rows option, this sets how many slides are in each grid row. slidesPerRow: 1, // # of slides to scroll at a time slidesToScroll: 1, // Transition speed speed: 500, // Enables touch swipe swipe: true, // Swipe to slide irrespective of slidesToScroll swipeToSlide: false, // Enables slide moving with touch touchMove: true, // To advance slides, the user must swipe a length of (1/touchThreshold) * the width of the slider. touchThreshold: 5, // Enable/Disable CSS Transitions useCSS: true, // Enable/Disable CSS Transforms useTransform: true, // Disables automatic slide width calculation variableWidth: false, // Vertical slide direction vertical: false, // hanges swipe direction to vertical verticalSwiping: false, // Ignores requests to advance the slide while animating waitForAnimate: true, // Set the zIndex values for slides, useful for IE9 and lower zIndex: 1000 });
5. API methods.
// destroys the plugin $('.demo').unslick(); // Triggers next slide $('.demo').slickNext(); // Triggers previous slide $('.demo').slickPrev(); // Pauses Autoplay $('.demo').slickPause(); // Starts Autoplay $('.demo').slickPlay(); // Goes to slide by index, skipping animation if second parameter is set to true $('.demo').slickGoTo(index,dontAnimate); // Returns the current slide index $('.demo').slickCurrentSlide(); // Adds a slide. If an index is provided, will add at that index, or before if addBefore is set. // If no index is provided, add to the end or to the beginning if addBefore is set. // Accepts HTML String $('.demo').slickAdd(element,index,addBefore); // Removes slide by index. If removeBefore is set true, remove slide preceding index, or the first slide if no index is specified. // If removeBefore is set to false, remove the slide following index, or the last slide if no index is set. $('.demo').slickRemove(index,removeBefore); // Filters slides using jQuery .filter syntax $('.demo').slickFilter(filter); // Removes applied filter $('.demo').slickUnfilter(); // Gets an option value. $('.demo').slickGetOption(option); // Changes a single option to given value; refresh is optional. $('.demo').slickSetOption(option,value,refresh); // Gets Slick Object $('.demo').getSlick();
6. Event handlers.
// Before slide change callback $('.demo').on('afterChange', function(event, slick, currentSlide){ // do something }); // Before slide change callback $('.demo').on('beforeChange', function(event, slick, currentSlide, nextSlide){ // do something }); // Fires after a breakpoint is hit $('.demo').on('breakpoint', function(event, slick, breakpoint){ // do something }); // When slider is destroyed, or unslicked. $('.demo').on('destroy', function(event, slick){ // do something }); // Fires when an edge is overscrolled in non-infinite mode. $('.demo').on('edge', function(event, slick, direction){ // do something }); // When Slick initializes for the first time callback. // Note that this event should be defined before initializing the slider. $('.demo').on('init', function(event, slick){ // do something }); // Every time Slick (re-)initializes callback $('.demo').on('reInit', function(event, slick){ // do something }); // Every time Slick recalculates position $('.demo').on('setPosition', function(event, slick){ // do something }); // Fires after swipe/drag $('.demo').on('swipe', function(event, slick, direction){ // do something }); // Fires after image loads lazily $('.demo').on('lazyLoaded', function(event, slick, image, imageSource){ // do something }); // Fires after image fails to load $('.demo').on('lazyLoadError', function(event, slick, image, imageSource){ // do something });
Changelog:
2022-11-22
- Doc updated
- Demos updated
v1.9.0 (2018-04-19)
- update.
v1.5.9 (2016-02-05)
- update.
v1.4.0 (2015-01-26)
- update.
v1.3.3 (2014-04-09)
- Fixing menu for firefox
v1.3.2 (2014-04-03)
- Changing prev/next anchors to button tags
- updating styles
- updating placeholder code
v1.2.8 (2014-03-31)
- Adding Fade
v1.2.7 (2014-03-30)
- Add/Remove items patch
v1.2.4 (2014-03-29)
- Removing sprites, adding icon font
v1.2.1 (2014-03-28)
- update.
This awesome jQuery plugin is developed by kenwheeler. For more Advanced Usages, please check the demo page or visit the official website.