Minimalist Background Image Carousel with jQuery and CSS3
File Size: | 5.84 KB |
---|---|
Views Total: | 1273 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
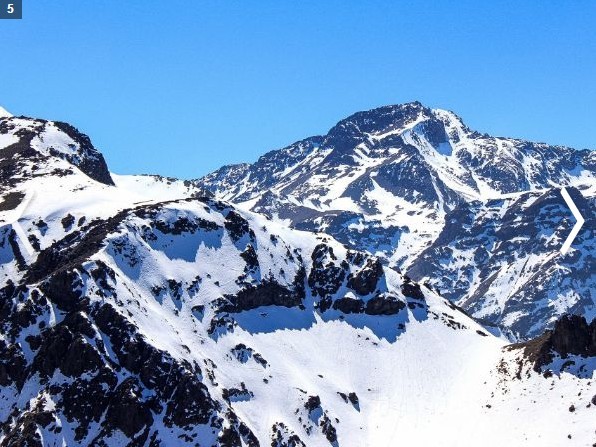
A dead simple jQuery slideshow / carousel component that allows for looping infinitely through a group of background images with a fadeIn / fadeOut transition effect based on CSS3 transitions.
How to use it:
1. Add background images to slides and the wrap them together with counter and navigation into a container.
<div class="fadeImgs"> <span class="thumb active" style="background-image: url('1.jpg');"></span> <span class="thumb" style="background-image: url('2.jpg')"></span> <span class="thumb" style="background-image: url('3.jpg')"></span> <span class="thumb" style="background-image: url('4.jpg')"></span> <span class="thumb" style="background-image: url('5.jpg')"></span> <div class="photoCount">5</div> <div class="photoNext"></div> <div class="photoPrev"></div> </div>
2. Add the following carousel styles into your webpage.
.fadeImgs { width: 600px; height: 450px; position: absolute; margin: -4px 0 0 -10px; } .fadeImgs .thumb { width: 600px; height: 450px; background-size: cover; background-position: center center; background-repeat: no-repeat; position: absolute; top: 0; opacity: 0; transition: opacity .25s ease-in-out; } .fadeImgs .thumb.active { opacity: 1; transition: opacity .25s ease-in-out; } .fadeImgs .photoCount { position: absolute; top: 0; left: 0; padding: 3px 8px 3px 8px; background-color: rgba(0, 0, 0, 0.5); color: #fff; font-weight: bold; font-size: 12px; overflow: hidden; z-index: 5; } .fadeImgs .photoNext { position: absolute; bottom: 188px; right: 10px; background-image: url(right-thin.png); width: 30px; height: 74px; opacity: .7; transition: opacity .25s ease-in-out; z-index: 5; cursor: pointer; } .fadeImgs .photoPrev { position: absolute; bottom: 188px; left: 10px; background-image: url(left-thin.png); width: 30px; height: 74px; opacity: .7; transition: opacity .25s ease-in-out; z-index: 5; cursor: pointer; } .fadeImgs .photoNext:hover { opacity: 1; } .fadeImgs .photoPrev:hover { opacity: 1; }
3. Include the latest version of jQuery JavaScript library at the bottom of the webpage.
<script src="//code.jquery.com/jquery-latest.min.js"></script>
4. The core JavaScript (jQuery script) to active the carousel.
$( document ).ready(function() { $( ".photoNext" ).click(function( event ) { event.preventDefault(); $(this).siblings( ".active" ).addClass( "fadeFrom" ); $( ".fadeFrom" ).nextOrFirst('.thumb').addClass( "fadeTo" ); $( ".fadeFrom" ).removeClass( "active" ); $( ".fadeFrom" ).removeClass( "fadeFrom" ); $( ".fadeTo" ).addClass( "active" ); $( ".fadeTo" ).removeClass( "fadeTo" ); }); $( ".photoPrev" ).click(function( event ) { event.preventDefault(); $(this).siblings( ".active" ).addClass( "fadeFrom" ); $( ".fadeFrom" ).prevOrLast('.thumb').addClass( "fadeTo" ); $( ".fadeFrom" ).removeClass( "active" ); $( ".fadeFrom" ).removeClass( "fadeFrom" ); $( ".fadeTo" ).addClass( "active" ); $( ".fadeTo" ).removeClass( "fadeTo" ); }); }); $.fn.nextOrFirst = function(selector){ var next = this.next(selector); return (next.length) ? next : this.prevAll(selector).last(); }; $.fn.prevOrLast = function(selector){ var prev = this.prev(selector); return (prev.length) ? prev : this.nextAll(selector).last(); };
This awesome jQuery plugin is developed by stevenoone. For more Advanced Usages, please check the demo page or visit the official website.