jQuery Auto Rotating Image Slider with CSS3 Wipe Effect
File Size: | 2.26 KB |
---|---|
Views Total: | 8119 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
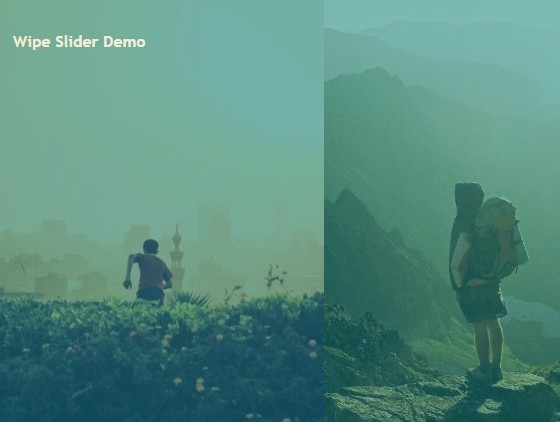
A jQuery based auto-rotating background image carousel/slideshow/slider that uses CSS background-size:cover
and background-position:center
for smooth wipe transition effects.
How to use it:
1. Create an image carousel/slider from an Html unordered list.
<ul class="cover-slider"> <li class="cover-slider-slide"> ... </li> <li class="cover-slider-slide"> ... </li> <li class="cover-slider-slide"> ... </li> <li class="cover-slider-slide"> ... </li> <li class="cover-slider-slide"> ... </li> </ul>
2. The primary CSS/CSS3 styles for the image carousel/slider.
.cover-slider-wrap { position: relative; max-width: 640px; height: 480px; margin: 2em auto; padding: 5em 1em; color: #fff; line-height: 2; font-weight: bold; box-shadow: 0 0 0.5em rgba(0, 0, 0, 0.5); } .cover-slider-inner { position: relative; min-height: 40em; text-align: center; z-index: 10; } .cover-slider { -webkit-backface-visibility: hidden; backface-visibility: hidden; } .cover-slider-slide { position: absolute; top: 0; bottom: 0; left: 0; right: 100%; padding: 0; margin: 0; background-size: cover; background-position: center; list-style: none; z-index: 0; opacity: .5; }
3. Add background images to the slides.
.cover-slider-slide:nth-child(1) { background-image: url("1.jpg"); } .cover-slider-slide:nth-child(2) { background-image: url("2.jpg"); } .cover-slider-slide:nth-child(3) { background-image: url("3.jpg"); } .cover-slider-slide:nth-child(4) { background-image: url("4.jpg"); }
4. The core CSS/CSS3 for the wipe transition effects.
.cover-slider-slide.active { -webkit-animation-duration: 2500ms; animation-duration: 2500ms; -webkit-animation-name: slidein; animation-name: slidein; -webkit-animation-fill-mode: forwards; animation-fill-mode: forwards; } .cover-slider-slide.inactive { -webkit-animation-duration: 2500ms; animation-duration: 2500ms; -webkit-animation-name: slideout; animation-name: slideout; -webkit-animation-fill-mode: forwards; animation-fill-mode: forwards; } @-webkit-keyframes slidein { from { left: 0; right: 100%; } to { left: 0; right: 0; } } @keyframes slidein { from { left: 0; right: 100%; } to { left: 0; right: 0; } } @-webkit-keyframes slideout { from { left: 0; right: 0; } to { left: 100%; right: 0; } } @keyframes slideout { from { left: 0; right: 0; } to { left: 100%; right: 0; } }
5. Load the latest version of jQuery library at the end of the document.
<script src="//code.jquery.com/jquery-2.1.3.min.js"></script>
6. The core JavaScript to enable the image carousel/slider.
jQuery(function($){ $('.cover-slider').each(function(){ var $slides = $(this).find('.cover-slider-slide'); var numSlides = $slides.length - 1; var i = 0; var rotate = function(){ $slides.removeClass('active inactive'); $slides.eq(i).addClass('inactive'); if(i == numSlides){ i = -1; } $slides.eq(++i).addClass('active'); var timer = window.setTimeout(rotate, 5000); }; rotate(); }); });
This awesome jQuery plugin is developed by tcmulder. For more Advanced Usages, please check the demo page or visit the official website.