Minimalist Navigatable Calendar In jQuery
File Size: | 3.86 KB |
---|---|
Views Total: | 4898 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
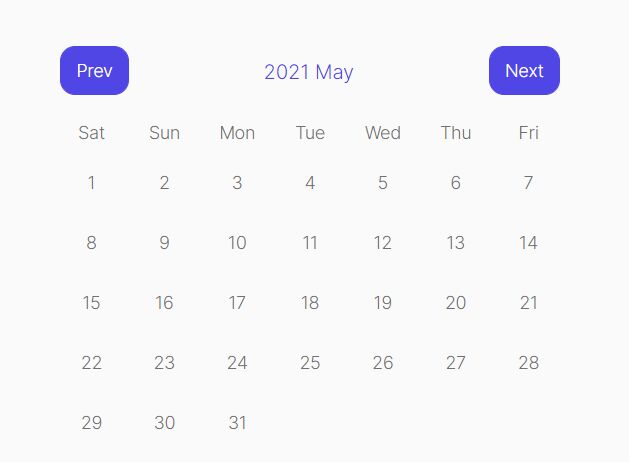
A minimal, clean, and responsive calendar UI component that enables the user to switch between months with prev/next buttons.
Written in jQuery and CSS Grid Layout.
How to use it:
1. Build the HTML structure for the calendar.
<section id="myCalendar"> <div class="hbContainer"> <div class="calendarYearMonth center"> <p class="left calBtn" onclick="prevMonth()"> Prev </p> <p id="yearMonth"> Jan 2021 </p> <p class="right calBtn" onclick="nextMonth()"> Next </p> </div> <div> <ol class="calendarList1"> <li class="day-name">Sat</li> <li class="day-name">Sun</li> <li class="day-name">Mon</li> <li class="day-name">Tue</li> <li class="day-name">Wed</li> <li class="day-name">Thu</li> <li class="day-name">Fri</li> </ol> <ol class="calendarList2" id="calendarList"> </ol> </div> </div> </section>
2. The necessary CSS styles for the calendar UI.
.hbContainer { max-width: 400px; margin: auto; } .left { float: left; } .right { float: right; } .center { text-align: center; } .calendarList1 { list-style: none; width: 100%; margin: 0; padding: 0; text-align: center; display: grid; grid-template-columns: repeat(7, 1fr); grid-template-rows: repeat(1, 40px); align-items: center; justify-items: center; grid-gap: 8px; font-size: 14px; color: #707070; } .calendarList2 { list-style: none; margin: 0; padding: 0; text-align: center; display: grid; grid-template-columns: repeat(7, 1fr); grid-template-rows: repeat(6, 40px); align-items: center; justify-items: center; grid-gap: 8px; font-size: 14px; color: #707070; } .calendarYearMonth { margin-top: 24px; } .calendarYearMonth p { display: inline-block; vertical-align: middle; } .calBtn { user-select: none; cursor: pointer; background: white; margin: 8px 0; padding: 8px 12px; border-radius: 12px; font-size: 14px; line-height: 22px; color: #707070; border: 1px solid #eaeaea; }
3. Load the main JavaScript calendar.js
after jQuery and done.
<script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/js/calendar.js"></script>
This awesome jQuery plugin is developed by SalehBUD. For more Advanced Usages, please check the demo page or visit the official website.