Simple Image Magnification Effect - Aui-core
File Size: | 159 KB |
---|---|
Views Total: | 5583 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
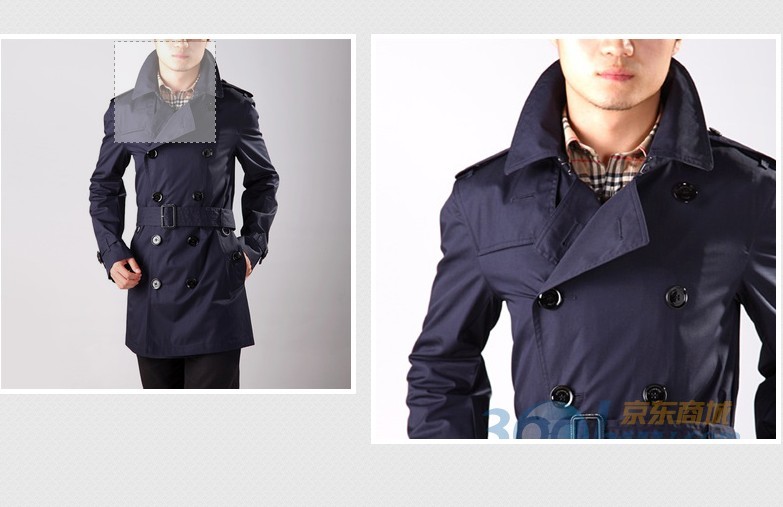
A simple and easy-to-use Image Magnification Effect using Aui-core js library that displays high resolution images with a magnifying glass effect when mouse hovers over the original images. It is very useful for e-commerce website that allows visitors to view the product details.
How to use it:
1. CSS
<style> #demo { margin: 0 auto; width: 350px; } #wraper { position: relative; width: 350px; height: 350px; background: #fff url(images/loading.gif) no-repeat center center; border: 5px solid #fff; } #small { width: 350px; height: 350px; overflow: hidden; } #mask { display: none; position: absolute; left: 0; top: 0; z-index: 2; height: 100px; overflow: hidden; width: 100px; border: 1px dashed #999; cursor: move; } #mask div { background: #fff; opacity: .4; filter:alpha(opacity:40); height: 100px; } #big { height: 400px; width: 400px; position: absolute; top: -5px; left: 370px; z-index: 1; border: 5px solid #fff; background: #fff url(images/loading.gif) no-repeat center center; overflow: hidden; } #bg { background: black; position: absolute; left: 0; top: 0; height: 350px; width: 350px; z-index: 1; opacity: 0; filter:alpha(opacity:0 ); } #big img { position: absolute; left: 0; top: 0; z-index: 0; } </style>
2. Include Aui-core.js
<script src="js/Aui-core-1.42-min.js" language="javascript" type="text/javascript"></script>
3. Call the plugin with options
<script> Aui.ready( function() { var oDemo = Aui( "#demo" ); var opt = { smallSrc : "images/small.jpg", smallWidth : 350, smallHeight : 350, bigSrc : "images/big.jpg", bigWidth : 800, bigHeight : 800 }; var oWin = Aui( window ); var owraper = Aui( "#wraper" ) var oSmall = Aui( "#small" ); var oBig = Aui( "#big" ); var obg = Aui( "#bg" ); var oMask = Aui( "#mask" ); var oBigImg = null; var oBigImgWidth = opt.bigWidth; var oBigImgHeight = opt.bigHeight; var iBwidth = oBig.width(); var iBheight = oBig.height(); oBig.setStyle( "display", "none" ); var iTop = owraper.top(); var iLeft = owraper.left(); var iWidth = owraper.width(); var iHeight = owraper.height(); var iSpeed = 200; var setOpa = function( o ) { o.style.cssText = "opacity:0;filter:alpha(opacity:0);" return o; }; var imgs = function( opt ) { if( Aui.typeOf( opt ) !== "object" ) return false; var oBig = new Image(); oBig.src = opt.bigSrc; oBig.width = opt.bigWidth; oBig.height = opt.bigHeight; var oSmall = new Image(); oSmall.src = opt.smallSrc; oSmall.width = opt.smallWidth; oSmall.height = opt.smallHeight; oBigImg = Aui( oBig ); return { bigImg : setOpa( oBig ), smallImg : setOpa( oSmall ) }; }; var append = function( o, img ) { o.append( img ); Aui( img ).fx( { opacity : 1 }, iSpeed*2, null , function() { this.style.cssText = ""; }); }; var eventMove = function( e ) { var e = e || window.event; var w = oMask.width(); var h = oMask.height(); var x = e.clientX - iLeft + oWin.scrollLeft() - w/2; var y = e.clientY - iTop + oWin.scrollTop() - h/2; var l = iWidth - w - 10; var t = iHeight - h - 10; if( x < 0 ) { x = 0; } else if( x > l ) { x = l; }; if( y < 0 ) { y = 0; } else if( y > t ) { y = t; }; oMask.setStyle( { left : x < 0 ? 0 : x > l ? l : x, top : y < 0 ? 0 : y > t ? t : y }); var bigX = x / ( iWidth - w ); var bigY = y / ( iHeight - h ); oBigImg.setStyle( { left : bigX * ( iBwidth - oBigImgWidth ), top : bigY * ( iBheight - oBigImgHeight ) }); return false; }; var eventOver = function() { oMask.show(); obg.stop() .fx( { opacity : .1 }, iSpeed ); oBig.show() .stop() .fx( { opacity : 1 }, iSpeed/2 ); return false; }; var eventOut = function() { oMask.hide(); obg.stop() .fx( { opacity : 0 }, iSpeed/2); oBig.stop() .fx( { opacity : 0 }, iSpeed, null, function() { Aui( this ).hide(); }); return false; }; var _init = function( object, oB, oS, callback ) { var num = 0; oBig.setStyle( "opacity",0 ); append( oB, object.bigImg ); append( oS, object.smallImg ); object.bigImg.onload = function() { num += 1; if( num === 2 ) { callback.call( object.smallImg ); }; }; object.smallImg.onload = function() { num += 1; if( num === 2 ) { callback.call( object.smallImg ); }; }; }; _init( imgs( opt ), oBig, oSmall, function() { oWin.resize( function() { iTop = owraper.top(); iLeft = owraper.left(); iWidth = owraper.width(); iHeight = owraper.height(); }); oSmall.hover( eventOver, eventOut ) .mousemove( eventMove ); }); }); </script>
4. Markup
<div id="demo"> <div id="wraper"> <div id="small"> <span id="mask"> <div></div> </span> <samp id="bg"></samp> </div> <div id="big"> </div> </div> </div>
This awesome jQuery plugin is developed by unknown. For more Advanced Usages, please check the demo page or visit the official website.