Generic Sliding Panel In jQuery - SlidePanel.js
File Size: | 15.8 KB |
---|---|
Views Total: | 2216 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
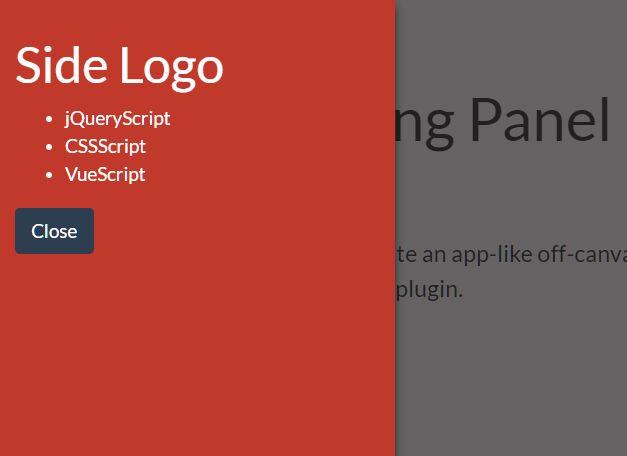
A lightweight yet versatile jQuery plugin used to create customizable sliding panels for site navigation on mobile, settings panes on dashboard, notification bars, feedback forms, social sharing widgets, and much more.
Features:
- Slides out from top, bottom, left, or right of the page.
- Configurable animations.
- Auto closes the sliding panel when clicking a link inside it.
- Push the body content to the other side similar to off-canvas push menu.
- Prevents page scroll when the sliding panel is activated.
- Useful callback functions.
How to use it:
1. Place the minified version of the SlidePanel.js plugin after loading jQuery.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/src/SlidePanel.min.js"></script>
2. Add your content (like menu items) to the sliding panel.
<section id="example" class="myPanel"> ... Any Content Here ... </section>
3. Initialize the plugin on the top container.
var instance = $('.myPanel').SlidePanel({ // options here });
4. Activate the sliding panel.
instance.activate();
5. Apply CSS styles to the sliding panel.
.myPanel { width: 400px; background-color: #fff; overflow: hidden !important; z-index: 150000; box-shadow: 8px 0 8px -10px #000000,-8px 0 8px -10px #000000; position: fixed; top: 0; left: 0; bottom: 0; z-index: 1999999999; text-align: left; background: #fff; pointer-events: auto; max-height: 100vh; display: flex; flex-direction: column; padding-top: 1rem; }
6. Display a background overlay when the sliding panel is activated.
<div class="overlay"></div>
var instance = $('.myPanel').SlidePanel({ onSlideOpening: function () { $(".overlay").removeClass("is-hidden").addClass("is-visible"); }, onSlideClosing: function () { $(".overlay").removeClass("is-visible").addClass("is-hidden"); } });
.navigation-overlay { position: fixed; top: 0; right: 0; bottom: 0; left: 0; height: 100vh; background-color: rgba(34,32,32,.7); z-index: 298; cursor: pointer; } .is-visible { visibility: visible; } .is-hidden { display: none; }
7. Insert a close button into the sliding panel.
<button class="slider-exit"> Close </button>
var instance = $('.myPanel').SlidePanel({ exit_selector: ".slider-exit" });
8. Create a trigger button to launch the sliding panel.
<button id="sliderpanel-toggle"> Close </button>
var instance = $('.myPanel').SlidePanel({ toggle: "#sliderpanel-toggle" });
9. Determine the placement of the sliding panel: top, right (default), bottom, left.
var instance = $('.myPanel').SlidePanel({ place: "left" });
10. More configuration options with default values.
var instance = $('.myPanel').SlidePanel({ // animation options animation_duration: "0.5s", animation_curve: "cubic-bezier(0.54, 0.01, 0.57, 1.03)", // push the main content to the other side body_slide: true, // prevent body scroll when active no_scroll: false, // auto dismiss when clicking a link auto_close: false, // set title here title_selector: '', // set the type of the HTML element that gets the role of the close action // you can use a button type for example close_selector: undefined, // set dynamic content to display in the panel content_selector: undefined });
11. Callback functions.
var instance = $('.myPanel').SlidePanel({ onSlideOpening: function (callback) { if (callback !== undefined) { callback.call(this); } }, onSlideOpened: function (callback) { if (callback !== undefined) { callback.call(this); } }, onSlideClosing: function (callback) { if (callback !== undefined) { callback.call(this); } }, onSlideClosed: function (callback) { if (callback !== undefined) { callback.call(this); } } });
Changelog:
2020-10-29
- v1.1
This awesome jQuery plugin is developed by jeromep01. For more Advanced Usages, please check the demo page or visit the official website.