Generating A Random Maze Background with jQuery
File Size: | 2.22 KB |
---|---|
Views Total: | 1128 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
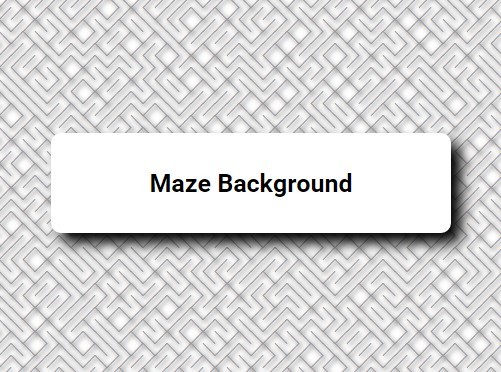
Maze is a small jQuery script that helps you generate a random maze background for your webpage using JavaScript and a little CSS magic.
How to use it:
1. Create a container for the maze background.
<div id="maze-bg"> </div>
2. The required CSS styles for the maze background. Large font = faster loop.
#maze-bg { font-size: 10px; line-height: 1; position: absolute; overflow: hidden; z-index: -1; color: #999; text-shadow: 2px 2px 6px #000; cursor: default; -moz-user-select: -moz-none; -khtml-user-select: none; -webkit-user-select: none; -ms-user-select: none; user-select: none; }
3. Include the needed jQuery JavaScript library on the web page.
<script src="//code.jquery.com/jquery-2.1.4.min.js"></script>
4. The core JavaScript (jQuery script) to generate a random maze background.
var maze; var el; var el_breedte; var el_hoogte; $(document).ready(function(){ el=$("#maze-bg"); calc_wh(); make_maze(el_breedte,el_hoogte); draw_maze(); $(window).on('resize',function(){ if(el.width() < $(window).width() || el.height() < $(window).height()){ calc_wh(); expand_maze(el_breedte,el_hoogte); draw_maze(); } el.width($(window).width()); el.height($(window).height()); }); }); function calc_wh(){ var el_content = el.hmtl; el.width("auto"); el.height("auto"); el.html('╱╲'); el_breedte=Math.ceil($(window).width()/(el.width()/2)); el_hoogte=Math.ceil($(window).height()/el.height()); el.html(el_content); el.width($(window).width()); el.height($(window).height()); } function make_maze(w,h){ maze = {}; for (i = 0; i < h; i++){ maze[i]=[]; for (j = 0; j < w; j++){ maze[i][j]=(Math.random()<.5?"\u2571":"\u2572"); } } } function expand_maze(w,h){ for (i = Object.keys(maze).length; i < h; i++){ maze[i]=[]; for (j = 0; j < w; j++){ maze[i][j]=(Math.random()<.5?"\u2571":"\u2572"); } } for (i = 0; i < Object.keys(maze).length; i++){ for (j = Object.keys(maze[i]).length; j < w; j++){ maze[i][j]=(Math.random()<.5?"\u2571":"\u2572"); } } } function draw_maze(){ el.empty(); for(i=0;i<el_hoogte;i++){ for(j=0;j<el_breedte;j++){ el.append(maze[i][j]); } el.append("<br\>"); } }
This awesome jQuery plugin is developed by cnomes. For more Advanced Usages, please check the demo page or visit the official website.