Simple Shopping Cart Plugin With jQuery And Bootstrap - mycart
File Size: | 499 KB |
---|---|
Views Total: | 108358 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
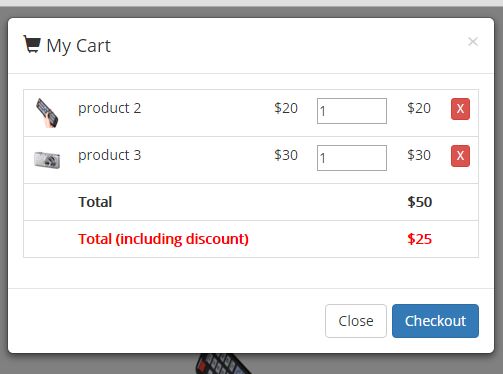
mycart is a jQuery plugin used to create a shopping cart interface with Bootstrap styles that features add to cart, payout modal and add/remove/edit cart items.
How to use it:
1. Load the required jQuery library and Bootstrap's JS & CSS files into your project.
<link rel="stylesheet" href="/path/to/bootstrap.min.css"> <script src="/path/to/jquery.min.js"></script> <script src="/path/to/bootstrap.min.js"></script>
2. Load the jQuery mycart plugin's script after jQuery library.
<script src="js/jquery.mycart.js"></script>
3. Insert a shopping cart icon into your webpage that will display how many products have been added to the cart.
<span class="glyphicon glyphicon-shopping-cart my-cart-icon"><span class="badge badge-notify my-cart-badge"></span></span>
4. Add your products with 'add to cart' and 'details' buttons into the webpage.
- data-id: product ID
- data-name: product name
- data-summary: product summary
- data-price: product price
- data-quantity: product quantity
- data-image: product image
<div class="row"> <div class="col-md-3 text-center"> <img src="images/img_1.png" width="150px" height="150px"> <br> product 1 - <strong>$10</strong> <br> <button class="btn btn-danger my-cart-btn" data-id="1" data-name="product 1" data-summary="summary 1" data-price="10" data-quantity="1" data-image="images/img_1.png">Add to Cart</button> <a href="#" class="btn btn-info">Details</a> </div> <div class="col-md-3 text-center"> <img src="images/img_2.png" width="150px" height="150px"> <br> product 2 - <strong>$20</strong> <br> <button class="btn btn-danger my-cart-btn" data-id="2" data-name="product 2" data-summary="summary 2" data-price="20" data-quantity="1" data-image="images/img_2.png">Add to Cart</button> <a href="#" class="btn btn-info">Details</a> </div> <div class="col-md-3 text-center"> <img src="images/img_3.png" width="150px" height="150px"> <br> product 3 - <strong>$30</strong> <br> <button class="btn btn-danger my-cart-btn" data-id="3" data-name="product 3" data-summary="summary 3" data-price="30" data-quantity="1" data-image="images/img_3.png">Add to Cart</button> <a href="#" class="btn btn-info">Details</a> </div> <div class="col-md-3 text-center"> <img src="images/img_4.png" width="150px" height="150px"> <br> product 4 - <strong>$40</strong> <br> <button class="btn btn-danger my-cart-btn" data-id="4" data-name="product 4" data-summary="summary 4" data-price="40" data-quantity="1" data-image="images/img_4.png">Add to Cart</button> <a href="#" class="btn btn-info">Details</a> </div> <div class="col-md-3 text-center"> <img src="images/img_5.png" width="150px" height="150px"> <br> product 5 - <strong>$50</strong> <br> <button class="btn btn-danger my-cart-btn" data-id="5" data-name="product 5" data-summary="summary 5" data-price="50" data-quantity="1" data-image="images/img_5.png">Add to Cart</button> <a href="#" class="btn btn-info">Details</a> </div> </div>
5. The JavaScript to enable the shopping cart plugin.
$(function () { var goToCartIcon = function($addTocartBtn){ var $cartIcon = $(".my-cart-icon"); var $image = $('<img width="30px" height="30px" src="' + $addTocartBtn.data("image") + '"/>').css({"position": "fixed", "z-index": "999"}); $addTocartBtn.prepend($image); var position = $cartIcon.position(); $image.animate({ top: position.top, left: position.left }, 500 , "linear", function() { $image.remove(); }); } $('.my-cart-btn').myCart({ classCartIcon: 'my-cart-icon', classCartBadge: 'my-cart-badge', affixCartIcon: true, checkoutCart: function(products) { $.each(products, function(){ console.log(this); }); }, clickOnAddToCart: function($addTocart){ goToCartIcon($addTocart); }, getDiscountPrice: function(products) { var total = 0; $.each(products, function(){ total += this.quantity * this.price; }); return total * 0.5; } }); });
6. Default options & callback functions.
classCartIcon: 'my-cart-icon', classCartBadge: 'my-cart-badge', classProductQuantity: 'my-product-quantity', classProductRemove: 'my-product-remove', classCheckoutCart: 'my-cart-checkout', affixCartIcon: true, showCheckoutModal: true, clickOnAddToCart: function($addTocart) { }, clickOnCartIcon: function($cartIcon, products, totalPrice, totalQuantity) { }, checkoutCart: function(products, totalPrice, totalQuantity) { }, getDiscountPrice: function(products, totalPrice, totalQuantity) { return null; }
Changelog:
2020-12-03
- v1.9: JS & CSS updated
2018-03-08
- v1.7
2017-09-06
- Checkout cart click event bind with document
2017-05-09
- numberOfDecimals can set for showing and calculating price
2017-05-07
- Bug fix for floating point calculation
2017-05-02
- Cart state remains same if cartItems field does not exists
2017-02-21
- Cart state remains same if cartItems field does not exists
- Integrate afterAddOnCart event for getting current cart state
2016-07-20
- Initial cart items set when myCart loaded
2016-04-21
- Option added for hide and show cart modal.
This awesome jQuery plugin is developed by Asraf-Uddin-Ahmed. For more Advanced Usages, please check the demo page or visit the official website.