Minimal Shopping Cart Plugin For jQuery - shopkart.js
File Size: | 6.68 KB |
---|---|
Views Total: | 7623 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
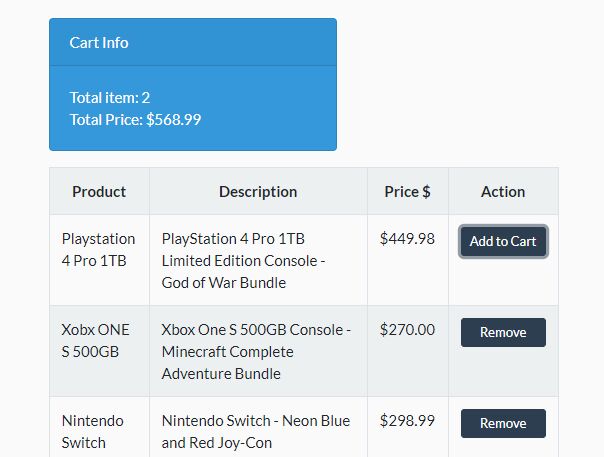
shopkart.js is a basic shopping cart plugin for your eCommerce website that enables the users to add existing product(s) into your shopping cart and automatically updates the quantity and total price for your product(s).
How to use it:
1. Create the html for the shopping cart.
<div class="cart"> <div class="cart-header">Cart Info</div> <div class="cart-body" data-kart="display"> <div>Total item: <span data-kart-total-item="0">0</span></div> <div>Total Price: $<span data-kart-total-price="0">0.00</span></div> </div> </div>
2. Create 'Add To Cart' buttons for your products as follows:
<button data-kart="item-button" data-kart-item-status="add-item" data-kart-item='{"id": 1, "price": 449.98}'> Add to Cart </button> <button data-kart="item-button" data-kart-item-status="add-item" data-kart-item='{"id": 2, "price": 270.98}'> Add to Cart </button> <button data-kart="item-button" data-kart-item-status="add-item" data-kart-item='{"id": 3, "price": 39.98}'> Add to Cart </button> ...
3. Place jQuery JavaScript library and the jQuery shopkart.js script at the bottom of the page.
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"> </script> <script src="shopkart.js"></script>
4. Initialize the shopping cart and done.
$(function () { $.shopkart(); });
5. Possible plugin options.
$.shopkart({ // custom button text kartItemButtonText: { add: "Add to Cart", remove: "Remove" }, kartItemButtonStatus: { add: "add-item", remove: "remove-item" }, /** * Allow to format the total items text. * @return {string} The total of items */ formatTotalItem: function () {}, /** * Allow to format the total price. * @return {string} The total price formatted. */ formatTotalPrice: function () {}, /** * Allow to customize the add button * @param {Object} The add button * @return {void} */ styleButtonAddItem: function () {}, /** * Allow to customize the remove button * @param {Object} $button The remove button * @return {void} */ styleButtonRemoveItem: function () {} });
This awesome jQuery plugin is developed by eduvnsm. For more Advanced Usages, please check the demo page or visit the official website.