Responsive Image Carousel With jQuery And CSS3
File Size: | 4.56 KB |
---|---|
Views Total: | 5787 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
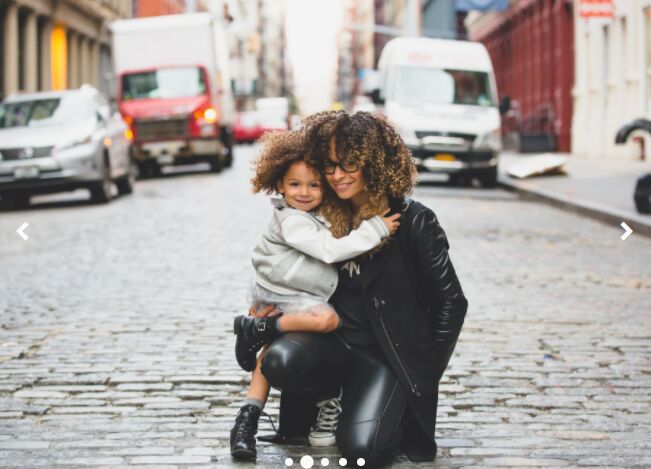
Just another responsive, flexible, full-width image carousel/slider built in jQuery, Font Awesome (OPTIONAL) and CSS3 animations.
Features:
- Auto-rotation with a countdown bar.
- Navigation arrows and pagination dots.
- Auto adjusts the size of your image to fit the screen width.
- Auto slides back to the first image as you reach the last one.
How to use it:
1. Add images together with carousel controls to the webpage.
<div class="trent-slider"> <div class="t-slide current-t-slide"> <img src="https://picsum.photos/1800/900?image=868" alt="" /> </div> <div class="t-slide"> <img src="https://picsum.photos/1800/900?image=838" alt="" /> </div> <div class="t-slide"> <img src="https://picsum.photos/1800/900?image=841" alt="" /> </div> <div class="t-slide"> <img src="https://picsum.photos/1800/900?image=839" alt="" /> </div> <div class="t-slide"> <img src="https://picsum.photos/1800/900?image=821" alt="" /> </div> <div class="t-slider-controls"> <div class="arrow right-arrow"> <div class="arrow-container"> <div class="arrow-icon"><i class="fa fa-chevron-right" aria-hidden="true"></i></div> </div> </div> <div class="arrow left-arrow"> <div class="arrow-container"> <div class="arrow-icon"><i class="fa fa-chevron-left" aria-hidden="true"></i></div> </div> </div> <div class="t-load-bar"> <div class="inner-load-bar"></div> </div> <div class="t-dots-container"> <div class="t-slide-dots-wrap"> <div class="t-slide-dots"> </div> </div> </div> </div> </div>
2. Load the Font Awesome library for the navigation icons (OPTIONAL).
<link rel="stylesheet" href="https://netdna.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
3. The basic styling for the image carousel.
.trent-slider { width: 100%; position: relative; overflow: hidden; } .t-slide { position: absolute; left: 0; right: 0; bottom: 0; top: 0; transition: .65s; } .t-slide img { width: 100%; height: 100%; }
4. The CSS for the carousel controls.
.t-slider-controls { position: absolute; top: 0; bottom: 0; left: 0; right: 0; } .t-slider-controls .arrow { min-height: 100%; width: 50px; position: relative; transition: .25s; } .t-slider-controls .arrow:hover { cursor: pointer; } .t-slider-controls .right-arrow { float: right; } .t-slider-controls .left-arrow { float: left; } .t-slider-controls .arrow .arrow-container { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); font-size: 20px; } .t-slider-controls .arrow .arrow-container .arrow-icon { position: relative; width: 42px; height: 42px; color: #fafafa; border-radius: 50%; } .t-slider-controls .arrow .arrow-container .arrow-icon i { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); } .t-slider-controls .t-load-bar { width: 100%; height: 5px; } .t-slider-controls .t-load-bar .inner-load-bar { background: rgba(200,200,200,0.65); height: 100%; } .t-slider-controls .t-dots-container { position: absolute; bottom: 0; left: 50%; transform: translateX(-50%); height: 20px; min-width: 350px; } .t-slider-controls .t-slide-dots-wrap { height: 100%; width: 100%; position: relative; } .t-slider-controls .t-slide-dots { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); display: table; content: ""; clear: both; } .t-slider-controls .t-slide-dots .t-dot { background: #fafafa; width: 8px; height: 8px; margin: 5px; float: left; border-radius: 50%; transition: .65s; } .t-slider-controls .t-slide-dots .t-dot.current-dot, .t-slider-controls .t-slide-dots .t-dot:hover { transform: scale(1.65); cursor: pointer; } @media screen and (min-width:768px) { .t-slider-controls .arrow:hover { background: rgba(0,0,0,0.32); } } @keyframes load { 0% { width:0%; } 100% { width:100%; } }
5. Load jQuery JavaScript library and the main JavaScript file main.js
at the end of the document.
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"> </script> <script src="js/main.js"></script>
Change log:
2018-02-24
- bugfix
This awesome jQuery plugin is developed by tmac9494. For more Advanced Usages, please check the demo page or visit the official website.