Create A Swipeable Bootstrap 4 Carousel With TouchSwipe Plugin
File Size: | 3.01 KB |
---|---|
Views Total: | 9787 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
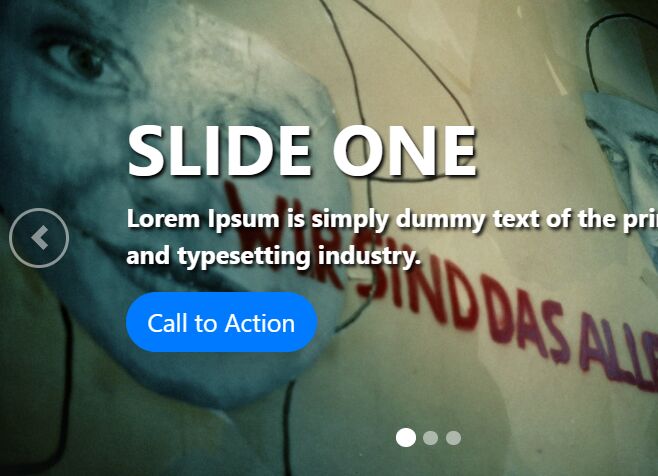
This is an example that shows how to integrate the jQuery touchSwipe plugin into the native Bootstrap 4 carousel component so that your visitors can navigate between carousel items on mobile devices using swipe events.
How to use it:
1. Download and insert the jQuery touchSwipe plugin into your Bootstrap project.
<!-- Bootstrap Files --> <link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/cdn/bootstrap.bundle.min.js"></script> <!-- jquery touchSwipe Plugin --> <script src="/path/to/cdn/jquery.touchSwipe.min.js"></script>
2. Create the HTML for the Bootstrap 4 carousel.
<div id="myCarousel" class="carousel slide carousel--fade shadow" data-ride="carousel"> <div class="carousel-inner"> <ol class="carousel-indicators"> <li data-target="#myCarousel" data-slide-to="0" class="active"></li> <li data-target="#myCarousel" data-slide-to="1"></li> <li data-target="#myCarousel" data-slide-to="2"></li> </ol> <div class="carousel-item active"> <img class="img-fluid" src="https://source.unsplash.com/random/1366x768?sig=123" width="1366" height="768" alt="First slide"> <div class="container"> <div class="carousel-caption text-left"> <h2 class="display-4 font-weight-bold">SLIDE ONE</h2> <p class="lead font-weight-bold">Lorem Ipsum is simply dummy text of the printing and typesetting industry.</p> <p><a class="btn btn-lg btn-primary rounded-pill shadow" href="#" role="button">Call to Action</a></p> </div> </div> </div> <div class="carousel-item"> <img class="img-fluid" src="https://source.unsplash.com/random/1366x768?sig=234" width="1366" height="768" alt="Second slide"> <div class="container"> <div class="carousel-caption"> <h2 class="display-4 font-weight-bold">SLIDE TWO</h2> <p class="lead font-weight-bold">Lorem Ipsum is simply dummy text of the printing and typesetting industry.</p> <p><a class="btn btn-lg btn-primary rounded-pill shadow" href="#" role="button">Call to Action</a></p> </div> </div> </div> <div class="carousel-item"> <img class="img-fluid" src="https://source.unsplash.com/random/1366x768?sig=456" width="1366" height="768" alt="Third slide"> <div class="container"> <div class="carousel-caption text-right"> <h2 class="display-4 font-weight-bold">SLIDE THREE</h2> <p class="lead font-weight-bold">Lorem Ipsum is simply dummy text of the printing and typesetting industry.</p> <p><a class="btn btn-lg btn-primary rounded-pill shadow" href="#" role="button">Call to Action</a></p> </div> </div> </div> <a class="carousel-control-prev" href="#carouselExampleControls" role="button" data-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="sr-only">Previous</span> </a> <a class="carousel-control-next" href="#carouselExampleControls" role="button" data-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="sr-only">Next</span> </a> </div> </div>
3. The example CSS to style the Bootstrap 4 carousel.
.carousel-item { background: #eee; } .carousel-item:hover { cursor: grab; } .carousel-caption { display: flex; flex-direction: column; justify-content: center; top: 0; bottom: 0; } .carousel-caption h2, .carousel-caption p.lead { text-shadow: 2px 2px 3px rgba(0, 0, 0, 1); } .carousel-indicators li { width: 0.75rem; height: 0.75rem; border-radius: 100%; align-self: center; transition: all 0.6s ease-in-out; } .carousel-indicators li.active { width: 1rem; height: 1rem; } .carousel-control-prev, .carousel-control-next { top: 50%; transform: translateY(-50%); width: 3rem; height: 3rem; border: 2px solid #fff; border-radius: 100%; } .carousel-control-prev { left: 10px; } .carousel-control-next { right: 10px; } .carousel-control-prev:hover, .carousel-control-next:hover { background: #000; color: #000; }
4. Initialize the Bootstrap 4 carousel component.
// init $(".carousel").carousel({ interval: false, pause: true, touch: true }); // enable prev/next navigation $(".carousel .carousel-control-prev").on("click", function () { $(".carousel").carousel("prev"); }); $(".carousel .carousel-control-next").on("click", function () { $(".carousel").carousel("next"); });
5. Add touch swipe to the Bootstrap 4 carousel component.
$(".carousel .carousel-inner").swipe({ swipeLeft: function (event, direction, distance, duration, fingerCount) { this.parent().carousel("next"); }, swipeRight: function () { this.parent().carousel("prev"); }, threshold: 0, tap: function (event, target) { window.location = $(this).find(".carousel-item.active a").attr("href"); }, excludedElements: "label, button, input, select, textarea, .noSwipe" });
This awesome jQuery plugin is developed by xcartmods. For more Advanced Usages, please check the demo page or visit the official website.