jQuery Plugin To Create Carousels Within Frames - FrameCarousel
File Size: | 6.41 MB |
---|---|
Views Total: | 5859 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
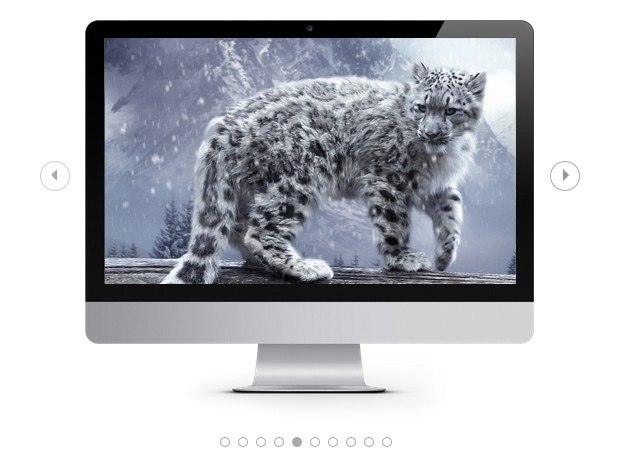
FrameCarousel is a lightweight jQuery plugin used to create responsive, mobile-friendly, CSS3 animated carousels within specified frames.
Features:
- Resizes itself for different screen sizes. Can even remove frame beyond a certain screen size.
- Highly responsive to touch gestures on smart devices.
- Takes advantage of CSS3 3D acceleration so it runs smooth on every device.
- A good number of customization options to make sure it fits nicely into your project.
Basic usage:
1. Load jQuery library and the jQuery FrameCarousel plugin's stylesheet and JavaScript in the web project.
<link href="jquery.frameCarousel.css" rel="stylesheet"> <script src="//code.jquery.com/jquery-1.11.3.min.js"></script> <script src="jquery.frameCarousel.js"></script>
2. Load the jQuery touchSwipe plugin for touch gestures support
<script src="jquery.touchSwipe.min.js"></script>
3. Create a DIV element to place the carousel. In this sample, the plugin will generate a carousel within an iMac display.
<div class="fc-mac"></div>
4. Initialize the plugin and set an array of images to be displayed into the iMac display.
$('.fc-mac').frameCarousel({ images: ['1.png', '2.png', '3.png',] });
5. Default plugin options.
// Enabled/disables debug mode. Debug mode allows you to print // debug messages on top of the frame in cases when console.log/alert aren't // readily available. debug: false, // first screen when the plugin intializes. Must be an integer between 0 // and image_count - 1, where image_count is total number of images you're // displaying inside the frame. first: 0, /* * swipeThreshold -- percentage of screen width * Determines when to intiate a next transition when user * is sliding a screen. * * For example, a value of 10 says that * initiate a move-to-next screen transition, if user has lifted * her finger(s) after having dragged current screen towards left by * a distance of 10% or more. */ swipeThreshold: 10, /** * Frame image that you'd like to use in the background. * The default value points to a phone frame included within * download package. */ frame: 'path/to/frame-carousel/sample/frame.png', /** * Width/height of the frame in pixels you'd like to apply in relation to different * screen sizes. * * If this option isn't specified at all, original dimensions of the frame image * will be used for all screen sizes. * * It takes an array of objects, each specifying frame dimensions in relation to a * particular screen width. For a given screen width, the plugin will iterate through * the array, and apply dimensions of the first matching object. * * If width isn't specified, but height is there, width would be calculated * using original image's aspect ratio. Same is true when height isn't specified, * but width is there. If neither height nor width are specified, original dimensions * of the image will be used. * * If width is set as -1, the frame will be removed for screens which match the criteria. */ frameSize: [{ /** * If width isn't specified, but height is specified, width would be calculated, * using original image's aspect ratio. If both height and width aren't */ width: [calculated at runtime], height: [calculated at runtime], minScreenWidth: 768, maxScreenWidth: 1024 }], /** * Marks bounding box within frame image where images are to be rendered. * All values are in percentages, so remain true no matter what size frame ends * up with. */ boundingBox: { left: '4.3%', top: '4.48%', width: '91.4%', height: '61.1%' }, // allows the plugin to trim itself down beyond given screensize collapseThreshold: 450, /** * An array of image links to be rendered within frame. Images don't have to adhere * to any size, but it'll help if their aspect ratio is same as aspect ratio of * boundingBox. */ images: ['path/to/frame-carousel/sample/1.jpg', 'path/to/frame-carousel/sample/2.jpg', ... 'path/to/frame-carousel/sample/10.jpg'], controlsPosition: { top: '35%' }, // autoplay autoplay: false, autoplayInterval: 2000
6. Methods.
// Grab the frameCarousel instance from host element var frameCarousel = $('.host-element').data('frameCarousel'); // The argument 'options' is optional in all of the following functions. // It is a hash/map with following possible properties (and defaults): // animate: true (determines if you want transition immediate or with animation) // Goes to next screen. If user is already at last screen, // it'll stay intact. frameCarousel.next( options ); // Goes to previous screen. If user is already at first screen, // it'll stay intact. frameCarousel.previous( options ); // Goes to screen identified by index. Stays intact if index // is out of bounds. frameCarousel.goto( index, options ); // Resizes the frame to given 'width' and 'height'. if no argument // is passed, it'd resize to original applicable size. // If only 'width' is passed, it'd determine 'height' by original // aspect ratio. Same is true when only 'height' is passed // and not 'width'. frameCarousel.resize( options ); // Call this if you'd like remove frame image. // Can be useful on smaller screens. frameCarousel.removeFrame(); // Gracefully destructs the instance. frameCarousel.destroy(); // If 'debug' mode is enabled (see options), any string passed to this method // will be printed over screen. Useful for testing in situations // when console.log/alert are not conveniently available. frameCarousel.debug( message );
7. Events.
// Grab the frameCarousel instance from host element. // Each callback receives a hash/map 'e' with relevant // information. $('.host-element') // triggers when a frameCarousel instance is ready .on('fc-ready', function( e ){ }) // triggers when a frameCarousel instance is destroyed .on('fc-destroy', function( e ){ }) // triggers when a screen transition begins .on('fc-transition-start', function( e ){ // e.from => screen index from where transition begun // e.to => screen index to where transition has to end }) // triggers when a screen transition ends .on('fc-transition-end', function( e ){ // e.from => screen index from where transition begun // e.to => screen index to where transition ended });
Change logs:
2017-02-18
- fixed event.
2015-09-28
- Adds support for auotplay
2015-09-18
- Adds new option 'collapseThreshold' that allows the plugin to trim itself down beyond given screensize
2015-08-10
- Fixes: Pagination button position gets messed up when it grows beyond one line
2015-06-17
- Implements previous, next and destroy methods
This awesome jQuery plugin is developed by Eastros. For more Advanced Usages, please check the demo page or visit the official website.