Advanced Keyboard Shortcut Plugin With jQuery - Chordly.js
File Size: | 45 KB |
---|---|
Views Total: | 731 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
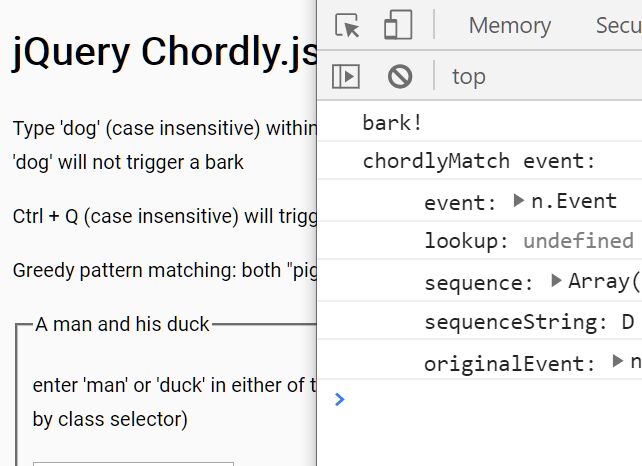
Chordly.js is an advanced, cross-browser key binding jQuery plugin that allows you to define and capture a sequence of key presses and then fire an event in the web application.
Perfect for cheatcodes, hotkeys, keyboard navigation, easter eggs, etc.
More features:
- Allows multiple key keyboard combinations on the page.
- Allows to ignore input fields.
- Allows to pause, resume, toggle the key bindings.
- Allows to customize the key buffer.
How to use it:
1. Include the Chordly.js plugin after you've loaded the latest jQuery JavaScript library.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/js/chordly.js"></script>
2. Initialize the plugin and bind key sequences using the sequenceMap
parameter:
$(document).chordly({ sequenceMap:[{ sequence: [ $.chordly.literalStringToSequence('dog'), $.chordly.literalStringToSequence('wolf'), ], matched: function () { console.log('bark!') } }, { sequence: $.chordly.stringToSequence('[66] A [84]'), // B A T matched: function () { console.log('na na na na na') } },{ sequence: [ $.chordly.makeSequencePart($.chordly.scanCodeMap.Q, null, null, true), ], matched: function () { console.log('ctrl + (q | Q)!') } },{ sequence: [ $.chordly.makeSequencePart($.chordly.scanCodeMap.H, true, null, false), ], matched: function () { console.log('shift + H!') } }] })
3. The example to bind a konami cheatcode.
$(document).chordly('bind', [{ sequence: [ $.chordly.makeSequencePart($.chordly.scanCodeMap.UpArrow), $.chordly.makeSequencePart($.chordly.scanCodeMap.UpArrow), $.chordly.makeSequencePart($.chordly.scanCodeMap.DownArrow), $.chordly.makeSequencePart($.chordly.scanCodeMap.DownArrow), $.chordly.makeSequencePart($.chordly.scanCodeMap.LeftArrow), $.chordly.makeSequencePart($.chordly.scanCodeMap.RightArrow), $.chordly.makeSequencePart($.chordly.scanCodeMap.LeftArrow), $.chordly.makeSequencePart($.chordly.scanCodeMap.RightArrow), $.chordly.makeSequencePart($.chordly.scanCodeMap.B), $.chordly.makeSequencePart($.chordly.scanCodeMap.A) ] matched: function () { console.log('cheat codes!') } }]); // or $(document).chordly('bindSequence', 'UpArrow UpArrow DownArrow DownArrow LeftArrow RightArrow LeftArrow RightArrow B A', function () { alert("Konami Code!"); });
4. All possible options to customize the plugin.
$(document).chordly({ // don't treat shift as a individual key press captureShift: false, // don't treat alt as a individual key press captureAlt: false, // don't treat ctrl as a individual key press captureCtrl: false, // don't capture key events on form elements ignoreFormElements: true, // event(s) to trigger the hot key check keyEvent: 'keyup', // default buffer length maxBufferLength: 5, // clear buffer on match clearBufferOnMatch: true, // mapping of key sequences sequenceMap: [], // true the pause the key listening paused: false, // timeout length for key buffer clearing (0 means no timeout) bufferTimeoutMs: 0, // timeout length since last recording of a key press before executing a match event (reset by next key press) greedyTimeoutMs: 0 });
5. Fire an event after you type the correct key squence.
$(document).chordly() .on('chordlyMatch', function (e) { console.log('chordlyMatch event:'); console.log('\t event:', e); console.log('\t lookup:', e.lookup); console.log('\t sequence:', e.sequence); console.log('\t sequenceString:', e.sequenceString); console.log('\t originalEvent:', e.originalEvent); })
6. API methods.
// pause $(document).chordly('pause'); // resume $(document).chordly('resume'); // toggle $(document).chordly('togglePause'); // destroy $(document).chordly('destroy'); // push a key squence to the buffer $(document).chordly('pushSequence', $.chordly.literalStringToSequence('dog')) // unbind $(document).chordly('unbind', $.chordly.literalStringToSequence('dog')); // act on butter $(document).chordly('actOnBuffer'); // clear sequence buffer $(document).chordly('clearSequenceBuffer'); // push and act a key squence $(document).chordly('pushSequenceAndAct', $.chordly.literalStringToSequence('dog'));
This awesome jQuery plugin is developed by sandialabs. For more Advanced Usages, please check the demo page or visit the official website.