jQuery Plugin To Show Tweetable Random Quotes On The Webpage - newQuotes
File Size: | 5.39 KB |
---|---|
Views Total: | 1236 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
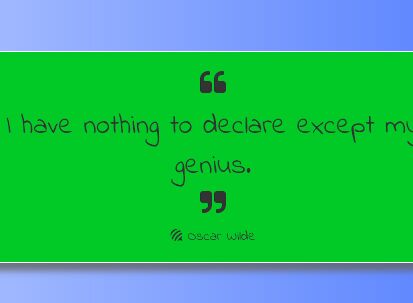
jQuery newQuotes helps you display tweetable random famous quotes on the webpage using Andruxnet's Quoto API.
How to use it:
1. Create a button to share the quote on Twitter.
<button id="btn1">Tweet</button>
2. Create a button to generate a new quote via AJAX.
<button id="btn2">New Quote</button>
3. Create a container to place the randomly generated quote.
<div id="quoteArea"> <div id="quoteItself"></div> <div id="quoteAuthor">author</div> </div>
4. Include the necessary jQuery library on the webpage.
<script src="jquery.min.js"></script>
5. The JavaScript to fetch and display a random famous quote on your webpage.
$(document).ready(function(){ // housekeeping: setup vars, 1st quote var quote = ""; var author = ""; changeBackground(); createQuote(); // buttons $("#btn1").on("click",function() { var twitterURL = "https://twitter.com/intent/tweet?text=" + quote + " " + author; window.open(twitterURL, 'twitter'); return false; }); $("#btn2").on("click",function(){ changeBackground(); createQuote(); }); // generate numbers for changing background colors function generateRandomNum(min,max){ return Math.floor(Math.random()*(max-min+1)+min); } function changeBackground(){ var colorR = generateRandomNum(0,255); var colorG = generateRandomNum(0,255); var colorB = generateRandomNum(0,255); var colorA = generateRandomNum(0,4); $("#quoteArea").css("background-color","rgba("+ colorR + "," + colorG + "," + colorB + "," + colorA + ")"); } //generate quote thru ajax call function createQuote(){ var output = $.ajax({ // The URL to the API. You can get this in the API page of the API you intend to consume url: 'https://andruxnet-random-famous-quotes.p.mashape.com/cat=famous', // The HTTP Method, can be GET POST PUT DELETE etc type: 'GET', // Additional parameters here data: {}, dataType: 'json', success: function(data) { quote = data.quote; author = data.author; $("#quoteItself").html("" + quote + ""); $("#quoteAuthor").html(" " + author); console.dir((data.source)); }, error: function(err) { console.log(err); }, beforeSend: function(xhr) { xhr.setRequestHeader("X-Mashape-Authorization", "8vdyiCzX3MmshyDl3zGkJz72KPySp1NguEbjsn8C4C1XHEwyec"); // Enter here your Mashape key } }); }; });
This awesome jQuery plugin is developed by davethemaker. For more Advanced Usages, please check the demo page or visit the official website.