Simple Online Notepad Using jQuery And Session Storage
File Size: | 4.84 KB |
---|---|
Views Total: | 702 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
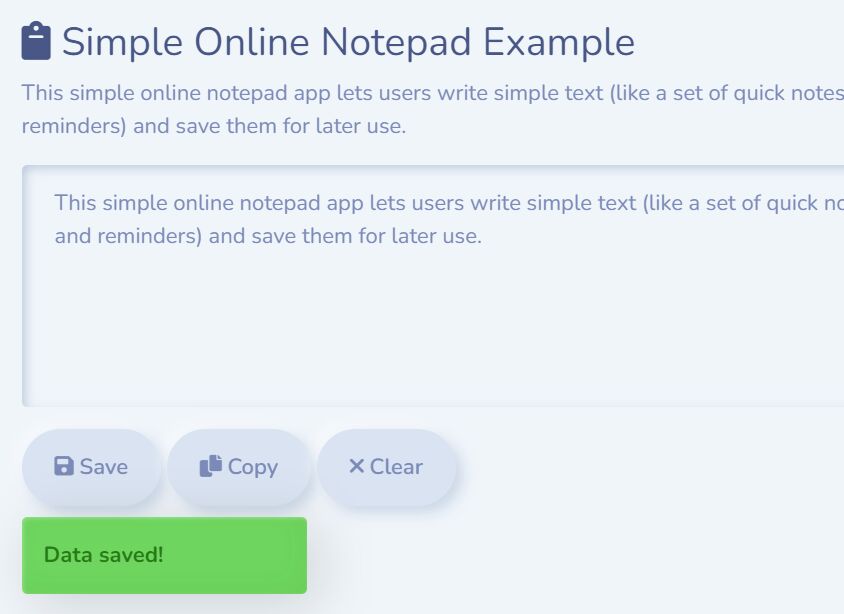
This simple online notepad app lets users write simple text (like a set of quick notes and reminders) and save them for later use. It's a very simple concept, but it can prove useful for anyone who may want to quickly write down some text or notes from their browser and have them saved locally, so they can access them at any time they please.
We use JQuery Javascript library to implement a text area which acts as online text notepad. The main advantage of this solution is that you can work with your notes in web browser and the typed text will be stored in the browser windows internal storage a.k.a session storage for temporary (session) use only.
How to use it:
1. Create a textarea for the notepad.
<textarea id="notepad" name="notepad" placeholder="Type or paste your text here..."> ... </textarea>
2. Create save/copy/clear buttons next to the notepad.
<a href="#" id="save-btn"> Save </a> <a href="#" class="copy-btn" data-clipboard-target="#notepad"> Copy </a> <a href="#" class="clear-btn"> Clear </a>
3. Load the needed jQuery and Session Storage Manipulation libraries in the document.
<script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="assets/js/jquery.session.js"></script>
4. The main script to activate the notepad.
$( document ).ready(function() { // get current session data var session_note = $.session.get('session_notepad');; if(session_note !== ""){ $("#notepad").val(session_note); } // save bnutton $("#save-btn").click(function() { //get current text var note = $("#notepad").val(); //set session data $.session.set('session_notepad', note); // show success message for 1 second $(".success-message").show(); setTimeout( function() { $(".success-message").hide(); }, 1000); }); //clear session data on button click $(".clear-btn").click(function() { $.session.remove('session_notepad'); $("#notepad").val(""); }); });
5. Enable the Copy button to copy your note in the clipboard.
<script src="https://cdnjs.cloudflare.com/ajax/libs/clipboard.js/2.0.8/clipboard.min.js"></script>
var clipboard = new ClipboardJS('.copy-btn');
This awesome jQuery plugin is developed by akassama. For more Advanced Usages, please check the demo page or visit the official website.