Terminal Input And Output Animations - jQuery Terminal-Emulator
File Size: | 57.1 KB |
---|---|
Views Total: | 1160 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
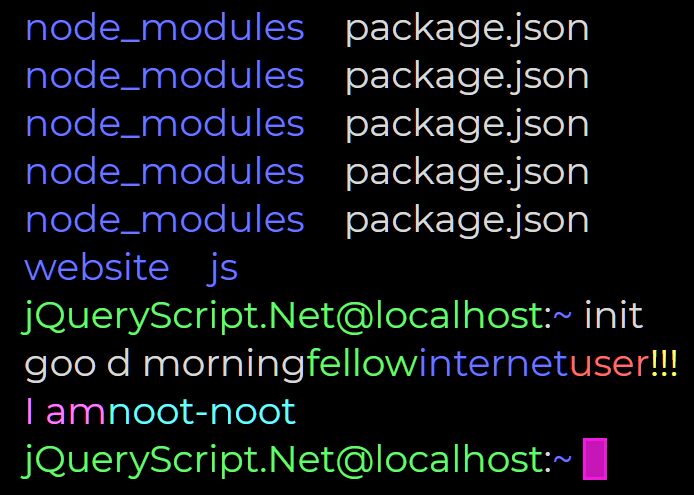
Yet another jQuery terminal emulator that simply emulates terminal input and output animations on the webpage.
Good for demonstrating how your codes or programs work just like in a command-line shell.
How to use it:
1. Load the minified version of the jQuery Terminal Emulator plugin after jQuery.
<script src="/path/to/cdn/jquery.slim.min.js"></script> <link rel="stylesheet" href="/path/to/dist/terminal-emulator.css" /> <script src="/path/to/dist/terminal-emulator.min.js"></script>
2. Create a container to hold the terminal emulator.
<div class="emulator-container"> <div id="example" class="emulator"></div> </div>
3. Define your commands in a JavaScript array as follows:
const myCommands = [ new Command( new Input( new Keystroke("init", "white", 0) ), new Output([ new Line([ new Keystroke("good ", "white", 0), new Keystroke("morning ", "white", 0), new Keystroke("fellow ", "green", 0), new Keystroke("internet ", "blue", 0), new Keystroke("user", "red", 0), new Keystroke("!!! ", "yellow", 0) ]), new Line([ new Keystroke("I am ", "magenta", 0), new Keystroke("noot-noot ", "cyan", 0) ]) ]) ), new Command( new Input( new Keystroke("ls", "magenta", 0) ), new Output([ new Line([ new Keystroke("css", "blue", 1), new Keystroke("index.html", "white", 1), new Keystroke("less", "blue", 0) ]), new Line([ new Keystroke("node_modules", "blue", 1), new Keystroke("package.json", "white", 1) ]), new Line([ new Keystroke("node_modules", "blue", 1), new Keystroke("package.json", "white", 1) ]), new Line([ new Keystroke("node_modules", "blue", 1), new Keystroke("package.json", "white", 1) ]), new Line([ new Keystroke("node_modules", "blue", 1), new Keystroke("package.json", "white", 1) ]), new Line([ new Keystroke("node_modules", "blue", 1), new Keystroke("package.json", "white", 1) ]), new Line([ new Keystroke("node_modules", "blue", 1), new Keystroke("package.json", "white", 1) ]), new Line([ new Keystroke("website", "blue", 1), new Keystroke("js", "blue", 0) ]) ]) ), new Command( new Input( new Keystroke("init", "white", 0) ), new Output([ new Line([ new Keystroke("goo d ", "white", 0), new Keystroke("morning ", "white", 0), new Keystroke("fellow ", "green", 0), new Keystroke("internet ", "blue", 0), new Keystroke("user", "red", 0), new Keystroke("!!! ", "yellow", 0) ]), new Line([ new Keystroke("I am ", "magenta", 0), new Keystroke("noot-noot ", "cyan", 0) ]) ]) ) ]
4. Initialize the terminal emulator. Possible parameters:
- commands: an array of commands
- element: where to place the terminal emulator
- user: username
- machine: machine name
- emulationSpeed: animation speed in ms
- readingSpeed: animation speed in ms
// Emulator(commands, element, user, machine, emulationSpeed, readingSpeed) emulator = new Emulator(myCommands, $("#example"), "root", "localhost", 160, 200);
5. Start the terminal emulator.
emulator.emulate();
This awesome jQuery plugin is developed by nout-kleef. For more Advanced Usages, please check the demo page or visit the official website.