Configurable Text Fader/Rotator In jQuery - PostillonTicker
File Size: | 14.5 KB |
---|---|
Views Total: | 2011 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
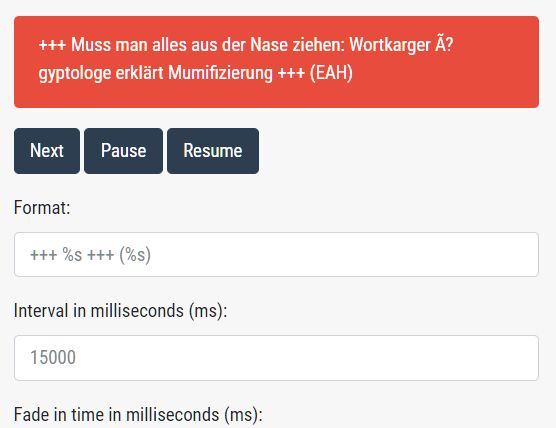
PostillonTicker is a jQuery news ticker plugin which makes it possible to rotate through text container with configurable fadeIn & fadeOut animations.
More features:
- Fetches content from a JSON file through AJAX.
- Custom display format.
- Allows you to turn off the fade animations.
- Lots of options, API methods and events.
How to use it:
1. Download and import the jquery.postillonTicker.js script into the document, after the latest jQuery JavaScript library.
<script src="/path/to/jquery.min.js"></script> <script src="/path/to/jquery.postillonTicker.js"></script>
2. Define your data (e.g. breaking news) in a JSON file.
{ "tickers": [{ "text": "Breaking News 1", "short": "BBC" }, { "text": "Breaking News 2", "short": "CNN" }, { "text": "Breaking News 3", "short": "Yahoo" }, { "text": "Breaking News 4", "short": "Google" }, { "text": "Breaking News 5", "short": "Fox" }] }
3. Create a container in which you want to generate the news ticker.
<div id="ticker"></div>
4. Initialize the news ticker and specify the path to the JSON file.
$("#ticker").postillonTicker({ tickerUrl: "data.json" });
5. Customize the animation speed.
$("#ticker").postillonTicker({ tickerUrl: "data.json", fadeInTime: 1000, fadeOutTime: 1000 });
6. Customize the display format.
$("#ticker").postillonTicker({ tickerUrl: "data.json", format: "+++ %s +++ (%s)" });
7. Specify the time to wati before transitioning to the next news. Default: 15000.
$("#ticker").postillonTicker({ tickerUrl: "data.json", interval: 5000 });
8. Full plugin options with default values.
$("#ticker").postillonTicker({ autoStart: true, fadeInTime: 1000, fadeOutTime: 1000, format: "+++ %s +++ (%s)", instantStart: true, interval: 15000, showLinks: true, tickerUrl: "data.json", _paused: false, _tickerIdx: 0, _tickers: [], _timer: null, });
9. API methods.
// returns the ticker object $("#ticker").getCurrentTicker(); // returns the option value $("#ticker").getSetting(option); // returns the option object $("#ticker").getSettings(); // checks if is running $("#ticker").isRunning(); // merges options $("#ticker").mergeSettings(options); // pauses the ticker $("#ticker").pause(); // restarts the ticker $("#ticker").restart(); // resumes the ticker $("#ticker").resume(); // updates options $("#ticker").setSetting(name, value); // sets options $("#ticker").setSettings(settings); // starts the ticker $("#ticker").start(); // stops the ticker $("#ticker").stop(); // updates the ticker $("#ticker").update([callback]);
10. Events.
$("#ticker").on('intervalChanged.postillonTicker', function(){ // after interval changed }) $("#ticker").on('paused.postillonTicker ', function(){ // after paused }) $("#ticker").on('resumed.postillonTicker', function(){ // after resumed }) $("#ticker").on('settingsChanged.postillonTicker', function(){ // after options changed }) $("#ticker").on('started.postillonTicker', function(){ // after started }) $("#ticker").on('starting.postillonTicker', function(){ // when starting }) $("#ticker").on('stopped.postillonTicker', function(){ // after stopped }) $("#ticker").on('stopping.postillonTicker', function(){ // when stopping }) $("#ticker").on('updated.postillonTicker', function(){ // after updated }) $("#ticker").on('updating.postillonTicker', function(){ // when updating })
This awesome jQuery plugin is developed by krrico. For more Advanced Usages, please check the demo page or visit the official website.