Digital & Analog Clock Using jQuery
File Size: | 12.8 KB |
---|---|
Views Total: | 1564 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
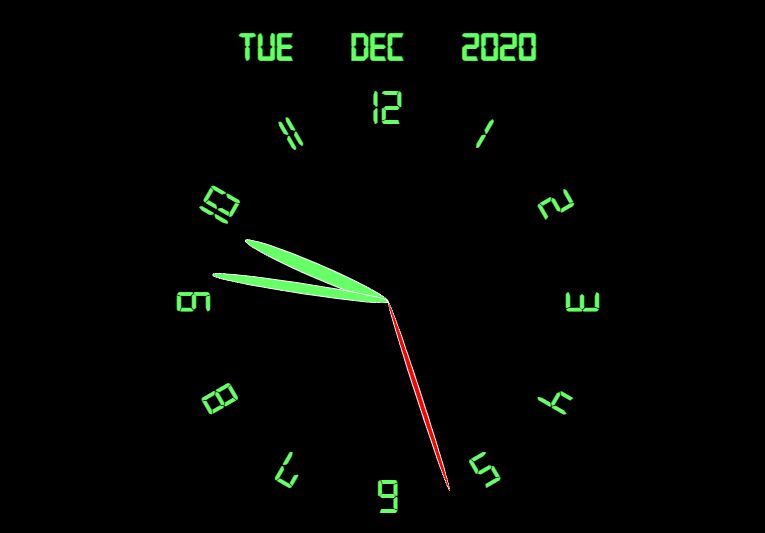
Digital Clock and Analog Clock implemented in jQuery, HTML, and CSS/CSS3.
How to use it:
1. Create the HTML for the digital & analog clocks.
<!-- Analog Clock --> <div class="clock tm"> <div class="hand hour"></div> <div class="hand minute"></div> <div class="hand second"></div> <div class="number number1">1</div> <div class="number number2">2</div> <div class="number number3">3</div> <div class="number number4">4</div> <div class="number number5">5</div> <div class="number number6">6</div> <div class="number number7">7</div> <div class="number number8">8</div> <div class="number number9">9</div> <div class="number number10">10</div> <div class="number number11">11</div> <div class="number number12">12</div> </div> <!-- Digital Clock --> <div class="digital"> <h1 class="digits hours"></h1> <h1 class='digits'>:</h1> <h1 class="digits minutes"></h1> <h1 class='digits'>:</h1> <h1 class="digits seconds"></h1> </div> <div id="date"> <h1 class="date day"></h1> <h1 class="date month"></h1> <h1 class="date year"></h1> </div>
2. The necessary CSS styles.
.clock { width: 350px; height: 350px; border-radius: 50%; border: 20x solid black; margin: 80px 0; position: relative; } .clock .number { --rotation: 0; position: absolute; width: 100%; height: 100%; text-align: center; font-size: 40px; transform: rotate(var(--rotation)); } .clock .number1 { --rotation: 30deg; } .clock .number2 { --rotation: 60deg; } .clock .number3 { --rotation: 90deg; } .clock .number4 { --rotation: 120deg; } .clock .number5 { --rotation: 150deg; } .clock .number6 { --rotation: 180deg; } .clock .number7 { --rotation: 210deg; } .clock .number8 { --rotation: 240deg; } .clock .number9 { --rotation: 270deg; } .clock .number10 { --rotation: 300deg; } .clock .number11 { --rotation: 330deg; } .clock .hand { --rotation: 0; position: absolute; bottom: 50%; left: 50%; border: 1px solid white; border-radius: 50%; transform-origin: bottom; transform: translateX(-50%) rotate(calc(var(--rotation) * 1deg)); } .clock .hand.second { width: 3px; height: 45%; background-color: red; } .clock .hand.minute { width: 7px; height: 40%; background-color: #66ff66; } .clock .hand.hour { width: 10px; height: 35%; background-color: #66ff66; } .digital { position: absolute; bottom: 5px; } #date { position: absolute; top: 50px; } .date { display: inline-block; margin: 0 20px; } .digits { display: inline-block; margin: 0 20px; }
3. Include the needed jQuery library on the page.
<script src="/path/to/cdn/jquery.min.js"></script>
4. The main JavaScript to enable the digital & analog clocks.
var hh=document.querySelector('.hour') var mh=document.querySelector('.minute') var sh=document.querySelector('.second') function Time(){ var date=new Date() var sec=date.getSeconds()/60 var min=(sec + date.getMinutes())/60 var hr=(min + date.getHours())/12 var weekNames=['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri','Sat'] var monthNames=['Jan', 'Feb', 'Mar', 'Apr', 'May','Jun','Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'] rotate(sh,sec) rotate(mh,min) rotate(hh,hr) var h=date.getHours() var m=date.getMinutes() var s=date.getSeconds() var d=date.getDay() var mo=date.getMonth() var y=date.getFullYear() if(h===0){ h=12 } if (h>12) { h=h-12 session='PM' } h=(h<10)? '0'+h:h m=(m<10)? '0'+m:m s=(s<10)? '0'+s:s $('.hours').text(h) $('.minutes').text(m) $('.seconds').text(s) $('.day').text(weekNames[d]) $('.month').text(monthNames[mo]) $('.year').text(y) setInterval(Time,1000) } function rotate(tag,ratio){ $(tag).css('--rotation',ratio*360) } Time()
This awesome jQuery plugin is developed by Techdevweb. For more Advanced Usages, please check the demo page or visit the official website.