Create An Analog Clock With jQuery And Bootstrap
File Size: | 64.3 KB |
---|---|
Views Total: | 1569 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
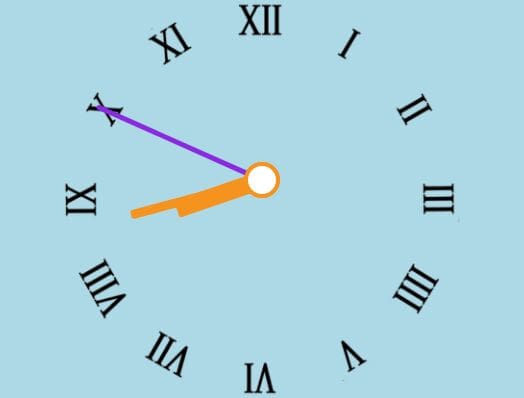
A realistic animated analog clock built on top of jQuery, Bootstrap, and CSS transforms.
How to use it:
1. Load the required jQuery library and Bootstrap's stylesheet in the document.
<link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <script src="/path/to/cdn/jquery.slim.min.js"></script>
2. Build the HTML structure for the analog clock.
<div class="container-fluid d-flex flex-column justify-content-center"> <!-- Clock Hands --> <div class="mx-auto axis"> <div class="mx-auto first common"></div> <div class="mx-auto second common"></div> <div class="mx-auto third common"></div> </div> <!-- Pivot --> <div class="mx-auto axis up"></div> </div>
3. The custom CSS styles for the analog clock.
/* content panel */ .container-fluid { height: 100vh; overflow: hidden; background-image: url("img/back.png"); background-attachment: fixed; background-repeat: no-repeat; background-position: center center; background-size: contain; background-color: lightblue; } /* Common properties to hands of clock */ .common { position: relative; transition: transform 0.5s linear; transform: rotate(0deg); transform-origin: bottom center; border-radius: 2px; transform-style: preserve-3D; box-shadow: 0 0 0.5px 0.5px transparent; overflow: hidden; } /* Pivot - Axis */ .axis { width: 32px; height: 32px; background-color: white; border-radius: 50%; border: 4px solid #f6931e; position: relative; z-index: 1050 !important; } /* Upper Pivot */ .up { bottom: 32px; } /* Hour hand */ .first { width: 16px; height: 80px; bottom: 68px; transform: rotate(0deg); background-color: #f6931e; } /* Minute hand */ .second { width: 8px; height: 120px; bottom: 188px; background-color: #f6931e; } /* Second hand */ .third { width: 4px; height: 160px; bottom: 348px; background-color: blueviolet; }
4. Update the clock using jQuery.
// Clock rotation $(document).ready(() => { // Get Date const date = new Date(); let h = date.getHours() % 12; let m = date.getMinutes(); let s = date.getSeconds(); // Initialize Clock let hA = updateClock($('.first'), Math.round((h * 360) / 12), 0); let mA = updateClock($('.second'), Math.round((m * 360) / 60), 0); let sA = updateClock($('.third'), Math.round((s * 360) / 60), 0); // Update Second setInterval(() => { sA = updateClock($('.third'), sA, 6); }, 1000); // Update Minute setInterval(() => { mA = updateClock($('.second'), mA, 1); }, 10000) // Update Hour setInterval(() => { hA = updateClock($('.first'), hA, 1); }, 50000); // Prevent overflow (Refresh After 6 hrs) setTimeout(() => { window.location.reload(); }, 21600000); }); // Update Time function updateClock(ref, start, add) { start += add; ref.css("transform", `rotate(${start}deg)`); return start; }
This awesome jQuery plugin is developed by Accelerator-One. For more Advanced Usages, please check the demo page or visit the official website.