Pick Hours of Availability For Each Day - Mark Your Calendar
File Size: | 9.13 MB |
---|---|
Views Total: | 22901 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
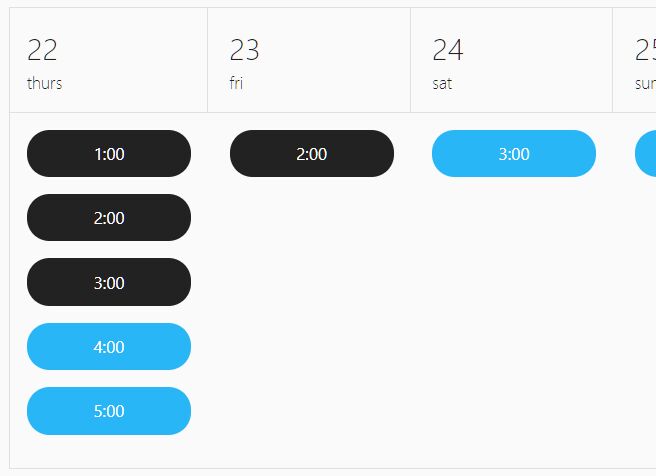
A tiny jQuery scheduler plugin to create a calendar with week view where users can pick a single or multiple hours of availability for each day of the week.
It provides an easy way to schedule events, appointments, or reservations by selecting available time slots on a pretty clean calendar interface.
How to use it:
1. Put the jQuery Mark Your Calendar plugin's JavaScript and CSS files on the HTML page.
<link rel="stylesheet" href="/path/to/css/mark-your-calendar.css" /> <script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/js/mark-your-calendar.js"></script>
2. Create a container in which the plugin renders the calendar.
<div id="picker"></div>
3. Define the available hours of availability for each day of the week.
const myHours: = [ ['1:00', '2:00', '3:00', '4:00', '5:00'], ['2:00'], ['3:00'], ['4:00'], ['5:00'], ['6:00'], ['7:00'] ],
4. Call the plugin on the calendar container and done.
$('#picker').markyourcalendar({ availability: myAvailability });
5. Determine whether to allow the user to select multiple time slots. Default: false.
$('#picker').markyourcalendar({ availability: myAvailability, isMultiple: true });
6. Localize the day/month names.
$('#picker').markyourcalendar({ availability: myAvailability, months: ['jan', 'feb', 'mar', 'apr', 'may', 'jun', 'jul', 'aug', 'sep', 'oct', 'nov', 'dec'], weekdays: ['sun', 'mon', 'tue', 'wed', 'thurs', 'fri', 'sat'] });
7. Determine the start date.
$('#picker').markyourcalendar({ availability: myAvailability, startDate: new Date() });
8. Specify an array of pre-selected dates.
$('#picker').markyourcalendar({ availability: myAvailability, selectedDates: [] });
9. Customize the prev/next navigation buttons.
$('#picker').markyourcalendar({ availability: myAvailability, prevHtml: prevHtml, nextHtml: nextHtml });
10. Get the selected date(s) using the onClick
callback.
$('#picker').markyourcalendar({ availability: myAvailability, onClick: function(ev, data) { // data is a list of datetimes console.log(data); var html = ``; $.each(data, function() { var d = this.split(' ')[0]; var t = this.split(' ')[1]; html += `<p>` + d + ` ` + t + `</p>`; }); $('#output').html(html); }, });
11. Trigger a function when users click navigation buttons.
$('#picker').markyourcalendar({ availability: myAvailability, onClickNavigator: function(ev, instance) { var arr = [ [ ['4:00', '5:00', '6:00', '7:00', '8:00'], ['1:00', '5:00'], ['2:00', '5:00'], ['3:30'], ['2:00', '5:00'], ['2:00', '5:00'], ['2:00', '5:00'] ], [ ['2:00', '5:00'], ['4:00', '5:00', '6:00', '7:00', '8:00'], ['4:00', '5:00'], ['2:00', '5:00'], ['2:00', '5:00'], ['2:00', '5:00'], ['2:00', '5:00'] ], [ ['4:00', '5:00'], ['4:00', '5:00'], ['4:00', '5:00', '6:00', '7:00', '8:00'], ['3:00', '6:00'], ['3:00', '6:00'], ['3:00', '6:00'], ['3:00', '6:00'] ], [ ['4:00', '5:00'], ['4:00', '5:00'], ['4:00', '5:00'], ['4:00', '5:00', '6:00', '7:00', '8:00'], ['4:00', '5:00'], ['4:00', '5:00'], ['4:00', '5:00'] ], [ ['4:00', '6:00'], ['4:00', '6:00'], ['4:00', '6:00'], ['4:00', '6:00'], ['4:00', '5:00', '6:00', '7:00', '8:00'], ['4:00', '6:00'], ['4:00', '6:00'] ], [ ['3:00', '6:00'], ['3:00', '6:00'], ['3:00', '6:00'], ['3:00', '6:00'], ['3:00', '6:00'], ['4:00', '5:00', '6:00', '7:00', '8:00'], ['3:00', '6:00'] ], [ ['3:00', '4:00'], ['3:00', '4:00'], ['3:00', '4:00'], ['3:00', '4:00'], ['3:00', '4:00'], ['3:00', '4:00'], ['4:00', '5:00', '6:00', '7:00', '8:00'] ] ] var rn = Math.floor(Math.random() * 10) % 7; instance.setAvailability(arr[rn]); } });
This awesome jQuery plugin is developed by makmac213. For more Advanced Usages, please check the demo page or visit the official website.