Terminal Text Typing Effect In JavaScript - Typed.js
File Size: | 1.29 MB |
---|---|
Views Total: | 22421 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
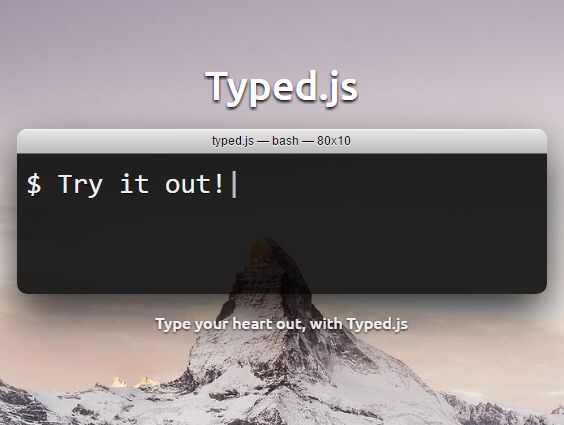
Yet another jQuery Vanilla JavaScript plugin for emulating the terminal typing effect that enables you to print html or plain text as if it's being typed on the screen.
Features:
- Supports both backspacing and fading effects.
- Custom typing speed.
- Has the ability to shuffle the html or text strings.
- Infinite loop is supported as well.
- Custom cursor character & animation.
- Callback functions.
Installation:
# NPM $ npm install typed.js # Bower $ bower install typed.js
How to use it:
1. Put the main JavaScript file typed.js
at the bottom of the webpage.
<!-- From Local --> <script src="lib/typed.js"></script> <!-- From CDN --> <script src="https://cdn.jsdelivr.net/npm/typed.js@latest/lib/typed.min.js"></script>
2. Or import the Typed.js as an ES module.
import Typed from 'typed.js';
3. Create an element in which you want to print the strings.
<div class="container"></div>
4. Call the function Typed()
on the container element and define an array of strings you'd like to print out.
var typed = new Typed('.container', { strings: ['<i>jQuery</i> Script.', 'A jQuery Plugin Website'] });
5. All default options to customize the text typing effect.
var typed = new Typed('.container', { /** * @property {array} strings strings to be typed * @property {string} stringsElement ID of element containing string children */ strings: [ 'These are the default values...', 'You know what you should do?', 'Use your own!', 'Have a great day!' ], stringsElement: null, /** * @property {number} typeSpeed type speed in milliseconds */ typeSpeed: 0, /** * @property {number} startDelay time before typing starts in milliseconds */ startDelay: 0, /** * @property {number} backSpeed backspacing speed in milliseconds */ backSpeed: 0, /** * @property {boolean} smartBackspace only backspace what doesn't match the previous string */ smartBackspace: true, /** * @property {boolean} shuffle shuffle the strings */ shuffle: false, /** * @property {number} backDelay time before backspacing in milliseconds */ backDelay: 700, /** * @property {boolean} fadeOut Fade out instead of backspace * @property {string} fadeOutClass css class for fade animation * @property {boolean} fadeOutDelay Fade out delay in milliseconds */ fadeOut: false, fadeOutClass: 'typed-fade-out', fadeOutDelay: 500, /** * @property {boolean} loop loop strings * @property {number} loopCount amount of loops */ loop: false, loopCount: Infinity, /** * @property {boolean} showCursor show cursor * @property {string} cursorChar character for cursor * @property {boolean} autoInsertCss insert CSS for cursor and fadeOut into HTML <head> */ showCursor: true, cursorChar: '|', autoInsertCss: true, /** * @property {string} attr attribute for typing * Ex: input placeholder, value, or just HTML text */ attr: null, /** * @property {boolean} bindInputFocusEvents bind to focus and blur if el is text input */ bindInputFocusEvents: false, /** * @property {string} contentType 'html' or 'null' for plaintext */ contentType: 'html' });
6. Callback functions.
var typed = new Typed('.container', { /** * Before it begins typing * @param {Typed} self */ onBegin: (self) => {}, /** * All typing is complete * @param {Typed} self */ onComplete: (self) => {}, /** * Before each string is typed * @param {number} arrayPos * @param {Typed} self */ preStringTyped: (arrayPos, self) => {}, /** * After each string is typed * @param {number} arrayPos * @param {Typed} self */ onStringTyped: (arrayPos, self) => {}, /** * During looping, after last string is typed * @param {Typed} self */ onLastStringBackspaced: (self) => {}, /** * Typing has been stopped * @param {number} arrayPos * @param {Typed} self */ onTypingPaused: (arrayPos, self) => {}, /** * Typing has been started after being stopped * @param {number} arrayPos * @param {Typed} self */ onTypingResumed: (arrayPos, self) => {}, /** * After reset * @param {Typed} self */ onReset: (self) => {}, /** * After stop * @param {number} arrayPos * @param {Typed} self */ onStop: (arrayPos, self) => {}, /** * After start * @param {number} arrayPos * @param {Typed} self */ onStart: (arrayPos, self) => {}, /** * After destroy * @param {Typed} self */ onDestroy: (self) => {} });
7. API methods.
// toggle the typing animation typed.toggle(); // start the typing animation typed.start(); // stop the typing animation typed.stop(); // reset the typing animation typed.reset(restart?); // destroy the instance typed.destroy();
Changelog:
2020-03-29
- Currently works as a Vanilla JavaScript plugin.
- Doc updated accordingly.
2017-06-25
- fixes new backspace existing text feature
This awesome jQuery plugin is developed by mattboldt. For more Advanced Usages, please check the demo page or visit the official website.