Filterable Portfolio Wall with jQuery and CSS3
File Size: | 2.37 KB |
---|---|
Views Total: | 16851 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
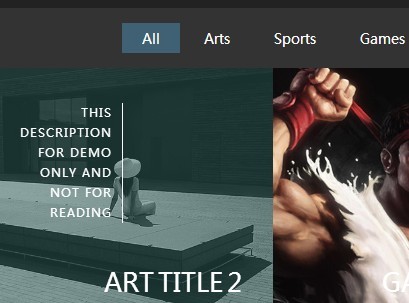
An amazing jQuery portfolio wall which allows the visitor to filter & shuffle a grid of images with cool CSS3 based image hover effects.
How to use it:
1. Add a filter control for the portfolio wall.
<div id="filter"> <button class="all active">All</button> <button class="cat-1">Cateogrize 1</button> <button class="cat-2">Cateogrize 2</button> <button class="cat-3">Cateogrize 3</button> </div>
2. Create the markup structure a portfolio wall as follows.
<div id="posts"> <div class="post cat-2"><img src="1.jpg"/> <div class="post-content"> <h2>Title 1</h2> <p>Description 1</p> <a href="#">View more</a> </div> </div> <div class="post cat-1"><img src="2.jpg"/> <div class="post-content"> <h2>Title 2</h2> <p>Description 2</p>> <a href="#">View more</a> </div> </div> <div class="post cat-3"><img src="3.jpg"/> <div class="post-content"> <h2>Title 3</h2> <p>Description 3</p> <a href="#">View more</a> </div> </div> <div class="post cat-1"><img src="4.jpg"/> <div class="post-content"> <h2>Title 4</h2> <p>Description 4</p> <a href="#">View more</a> </div> </div> <div class="post cat-2"><img src="5.jpg"/> <div class="post-content"> <h2>Title 5</h2> <p>Description 5</p> <a href="#">View more</a> </div> </div> <div class="post cat-3"><img src="6.jpg"/> <div class="post-content"> <h2>Title 6</h2> <p>Description 6</p> <a href="#">View more</a> </div> </div> </div>
3. The required CSS styles for the portfolio wall.
* { margin: 0; padding: 0; outline: 0; -webkit-box-sizing: border-box; -moz-box-sizing: border-box; box-sizing: border-box; } body { font-family: 'Open Sans', sans-serif; background-color: #222; color: #FFF; font-size: 14px; line-height: 20px; letter-space: .05px; } .hidden { display: none; } #filter { text-align: center; background-color: #333; padding: 15px; } #filter button { border: 0; background-color: #333; font-family: 'Open Sans', sans-serif; font-size: 14px; color: #FFF; cursor: pointer; padding: 5px 20px; } #filter button.active { background-color: #406174; } #filter button:focus { outline: 0; } #posts { max-width: 1200px; margin: 0 auto; } #posts .post { width: 25%; float: left; height: 250px; overflow: hidden; text-align: center; cursor: pointer; position: relative; z-index: 1; display: inline-block; background: #2e5d5a; } #posts .post img { position: relative; display: block; width: -webkit-calc(100% + 60px); width: calc(100% + 60px); opacity: 1; -webkit-transition: opacity 0.35s, -webkit-transform 0.35s; transition: opacity 0.35s, transform 0.35s; -webkit-transform: translate3d(-30px, 0, 0) scale(1.12); transform: translate3d(-30px, 0, 0) scale(1.12); -webkit-backface-visibility: hidden; backface-visibility: hidden; min-height: 100%; } #posts .post:hover img { opacity: 0.5; -webkit-transform: translate3d(0, 0, 0) scale(1); transform: translate3d(0, 0, 0) scale(1); } #posts .post .post-content { padding: 2em; color: #fff; text-transform: uppercase; font-size: 1.25em; -webkit-backface-visibility: hidden; backface-visibility: hidden; } #posts .post .post-content::before, #posts .post .post-content::after { pointer-events: none; } #posts .post .post-content, #posts .post a { position: absolute; top: 0; left: 0; width: 100%; height: 100%; } #posts .post a { z-index: 1000; text-indent: 200%; white-space: nowrap; font-size: 0; opacity: 0; } #posts .post h2 { word-spacing: -0.15em; font-weight: 300; position: absolute; right: 0; bottom: 0; padding: 1em 1.2em; } #posts .post h2, #posts .post p { margin: 0; } #posts .post p { letter-spacing: 1px; font-size: 68.5%; padding: 0 10px 0 0; width: 50%; border-right: 1px solid #fff; text-align: right; opacity: 0; -webkit-transition: opacity 0.35s, -webkit-transform 0.35s; transition: opacity 0.35s, transform 0.35s; -webkit-transform: translate3d(-40px, 0, 0); transform: translate3d(-40px, 0, 0); } #posts .post:hover p { opacity: 1; -webkit-transform: translate3d(0, 0, 0); transform: translate3d(0, 0, 0); } @media screen and (max-width: 600px) { #posts .post { width: 50%; } }
4. Include the latest version of jQuery library at the bottom of the document.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
5. The Javascript to enable the filterable portfolio wall.
$(document).ready(function(){ $('.hidden').css('display','none'); $( "#filter button" ).each(function() { $(this).on("click", function(){ var filter = $(this).attr('class'); if ( $(this).attr('class') == 'all' ) { $('.hidden').contents().appendTo('#posts').hide().show('slow'); $( "#filter button" ).removeClass('active'); $(this).addClass('active'); $("#filter button").attr("disabled", false); $(this).attr("disabled", true); } else { $('.post').appendTo('.hidden'); $('.hidden').contents().appendTo('#posts').hide().show('slow'); $('.post:not(.' + filter + ')').appendTo('.hidden').hide('slow'); $( "#filter button" ).removeClass('active'); $(this).addClass('active'); $("#filter button").attr("disabled", false); $(this).attr("disabled", true); }; }); }); })
This awesome jQuery plugin is developed by midoghranek. For more Advanced Usages, please check the demo page or visit the official website.