Pinterest Style Dynamic Layout jQuery Plugin - Masonry
File Size: | 46.7 KB |
---|---|
Views Total: | 11676 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
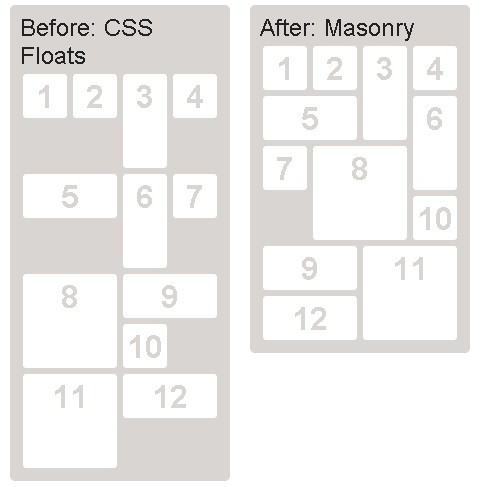
Masonry is a Pinterest Style Dynamic Grid Layout jQuery & Vanilla JavaScript Plugin for modern web & mobile design.
Whereas floating arranges elements horizontally then vertically, Masonry arranges elements vertically, positioning each element in the next open spot in the grid. The result minimizes vertical gaps between elements of varying height, just like a mason fitting stones in a wall.
Also can be used as a standalone JavaScript library without any 3rd dependencies.
Looking for more alternatives to create Masonry style layout? Don't forget to check out our 10 Best jQuery/JavaScript Masonry Layout Plugins and 10 Best Grid Layout Systems In JavaScript And CSS.
How to use it:
1. Install & download the Masonry via package managers.
# NPM $ npm install masonry --save
2. Or directly load the main script from a CDN.
<script src="https://unpkg.com/browse/masonry-layout/dist/masonry.pkgd.min.js"></script> <!-- or --> <script src="https://cdn.jsdelivr.net/npm/masonry-layout/masonry.min.js"></script>
3. Insert your own items to the grid.
<div class="grid"> <div class="grid-item">Item 1</div> <div class="grid-item">Item 2</div> <div class="grid-item">Item 3</div> ... </div>
4. Initialize the Masonry as a jQuery plugin (Requires jQuery library).
<script src="/path/to/cdn/jquery.min.js"></script>
$('.grid').masonry({ itemSelector: '.grid-item' });
5. Initialize the Masonry as a vanilla JavaScript plugin.
var elem = document.querySelector('.grid'); var msnry = new Masonry( elem, { itemSelector: '.grid-item' });
6. Possible options to customize the grid layout.
$('.grid').masonry({ // selector of grid item itemSelector: '.grid-item', // column width in pixels columnWidth: 200, // sets item positions in percent values, rather than pixel values percentPosition: true, // space between grid items gutter: 10, // horizontal left-to-right order horizontalOrder: true, // specifies which elements are stamped within the layout stamp: '.stamp', // fits the available number of columns fitWidth: true, // the horizontal flow of the layout originLeft: false, // the vertical flow of the layout originTop: false, // additianal styles for the container containerStyle: null, // transition speed transitionDuration: '0.2s', // staggers item transitions in milliseconds stagger: 30, // enables/disables window resize events resize: false, // enables/disables initial layout initLayout: false });
7. API methods to control the masonry layout.
// adds items and re-init the layout msnry.appended( elements ); msnry.prepended( elements ); // remove elements msnry.remove( elements ); // re-init the layout msnry.layout(); // re-layout items // items Array of Masonry.Items // isStill Boolean Disables transitions msnry.layoutItems( items, isStill ); // stamp/unstamp elements in the layout. msnry.stamp( elements ); msnry.unstamp( elements ); // adds more items msnry.addItems( elements ); // reload items msnry.reloadItems(); // destroy the instance msnry.destroy(); // return an array of item elements. msnry.getItemElements(); // get the Masonry instance var msnry = Masonry.data( element );
9. Event handlers.
// msnry.on( eventName, listener ); // msnry.off( eventName, listener ); // msnry.once( eventName, listener ); msnry.on( 'layoutComplete', function( laidOutItems ) { do something }); msnry.on( 'removeComplete', function( removedItems ) { do something });
Changelog:
2020-02-23
- Doc updated
v4.2.2 (2018-07-05)
- use float values for position
2018-06-07
- DOC updated
This awesome jQuery plugin is developed by desandro. For more Advanced Usages, please check the demo page or visit the official website.