Versatile Grid Layout System - Packery
File Size: | 82.5 KB |
---|---|
Views Total: | 2641 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
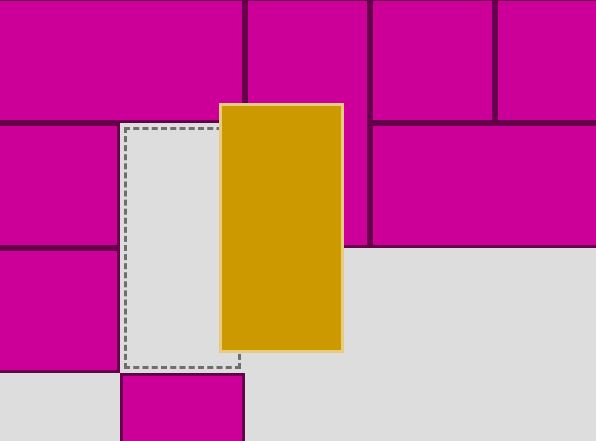
Packery is a versatile layout system designed to simplify the task of creating responsive, cross-platform grids for the modern web layout.
Features:
- Can be implemented as jQuery or Vanilla JS plugin.
- Custom horizontal and vertical space between grid items.
- Smooth transitions when arranging grid items.
- Compatible with the Draggabilly library to create a draggable grid layout.
- Supports any layout types: Masonry, Mosaic, etc.
Basic usage:
1. Install the package and import the Packery.js library into the document.
# NPM $ npm install packery --save
<!-- jQuery is OPTIONAL --> <script src="/path/to/cdn/jquery.min.js"></script> <!-- For Draggable layout --> <script src="/path/to/cdn/draggabilly.pkgd.min.js"></script> <!-- Packery.js library --> <script src="/path/to/dist/packery.pkgd.min.js"></script> <!-- Or from a CDN --> <script src="https://unpkg.com/packery/dist/packery.pkgd.min.js"></script>
2. Insert grid items to the layout.
<div class="grid"> <div class="grid-item"></div> <div class="grid-item"></div> <div class="grid-item"></div> <div class="grid-item"></div> <div class="grid-item"></div> ... </div>
3. Call the main function packery
on the top container and done.
// As a jQuery plugin var $grid = $('.grid').packery({ itemSelector: '.grid-item' }); // As a Vanilla JS plugin var grid = document.querySelector('.grid'); var pckry = new Packery( grid, { itemSelector: '.grid-item' });
4. Make all grid items draggable.
var draggies = []; var isDrag = false; $grid.find('.grid-item').each( function( i, gridItem ) { var draggie = new Draggabilly( gridItem ); draggies.push( draggie ); // bind drag events to Packery $grid.packery( 'bindDraggabillyEvents', draggie ); });
5. All possible options to customize the grid layout system.
var $grid = $('.grid').packery({ // item selector itemSelector: '.grid-item', // column width // aligns items to a horizontal grid columnWidth: 60, // row height // aligns items to a vertical grid rowHeight: 60, // space between items gutter: 10, // sets item positions in percent values percentPosition: true, // arranges items around these elements stamp: '.stamp', // arranges items horizontally horizontal: true, // false: right-to-left layout originLeft: true, // false: bottom-to-up layout originTop: true, // additional styles for container element containerStyle: null, // duration of transition transitionDuration: '0.2s', // transitions grid items incrementally after one another stagger: 30, // enable/disable window resize behavior resize: false, // false: initialize the layout manually initLayout: false });
6. API methods.
// shifts all item positions instance.shiftLayout(); // arranges specified grid items instance.layoutItems( items, isInstant ); // fits an item element within the layout instance.fit( element, x, y ); // stamps/unstamps element in the layout instance.stamp( elements ); instance.unstamp( elements ); // appends/prepend elements instance.appended( elements ); instance.prepended( elements ); // adds/removes items instance.addItems( elements ); instance.remove( elements ); // reloads all items instance.reloadItems(); // gets an array of item elements instance.getItemElements(); // destroy the instance instance.destroy(); // gets the instance via its element instance.data( element );
7. Event handlers.
instance.on( 'layoutComplete', function( laidOutItems ) { // do something }); instance.on( 'dragItemPositioned', function( draggedItem ) { // do something }); instance.on( 'fitComplete', function( item ) { // do something }); instance.on( 'removeComplete', function( removedItems ) { // do something });
This awesome jQuery plugin is developed by metafizzy. For more Advanced Usages, please check the demo page or visit the official website.