Create Draggable Bootstrap Grid Layouts With Gridstrap.js
File Size: | 156 KB |
---|---|
Views Total: | 9035 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
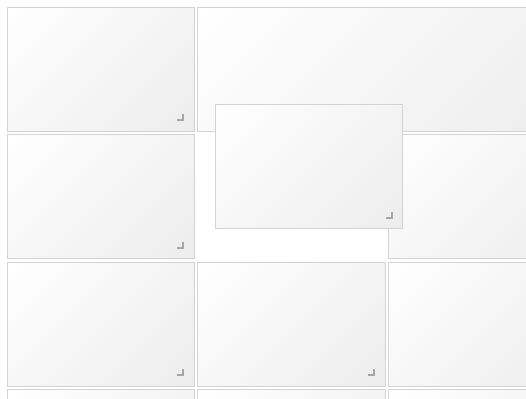
Gridstrap.js is a jQuery plugin to create a responsive, draggable, resizable Bootstrap grid layout where the users can move & rearrange grid items via drag'n'drop and touch events.
Supports both Bootstrap 4 and Bootstrap 3 frameworks. Also works with nested grid items.
See Also:
How to use it:
1. Load the jquery.gridstrap.css
after the Bootstrap's stylesheet.
<link rel="stylesheet" href="/path/to/bootstrap.min.css" /> <link href="/path/to/dist/jquery.gridstrap.css" rel="stylesheet" />
2. Load the jquery.gridstrap.js
after the latest jQuery JavaScript library.
<script src="/path/to/jquery.min.js"></script> <script src="/path/to/dist/jquery.gridstrap.js"></script>
3. Apply the function gridstrap
to the existing Bootstrap grid layout within your document. That's it.
<div id="example" class="row"> <div class="col-sm-4 item">Drag Me</div> <div class="col-sm-4 item">Drag Me</div> <div class="col-sm-4 item">Drag Me</div> </div>
$(function(){ $('#example').gridstrap({ /* options here */ }); });
4. All default configuration options to customize the draggable grid layout.
$('#example').gridstrap({ // Selector of grid cells relative to parent element. gridCellSelector: '>*', // CSS class applied to 'hidden' cells. hiddenCellClass: 'gridstrap-cell-hidden', // CSS class applied to 'visible' cells. visibleCellClass: 'gridstrap-cell-visible', // CSS class applied to non-contiguous placeholder cells. nonContiguousPlaceholderCellClass: 'gridstack-noncontiguous', // CSS class applied to the cell that is dragging. dragCellClass: 'gridstrap-cell-drag', // CSS class applied to the cell that is resizing. resizeCellClass: 'gridstrap-cell-resize', // CSS class applied to the container element. visibleCellContainerClass: 'gridstrap-container', // Where to bind mousemouse and mouseup events. mouseMoveSelector: 'body', // Where to append 'visible' cell container to. // If set to Null the plugin will use the element on which the plugin initializes. visibleCellContainerParentSelector: null, // Selector of drag handle dragCellHandleSelector: '*', // Enable draggable. draggable: true, // Rearrange grid celles on drag. rearrangeOnDrag: true, // Selector of resize handle. // null = disable resizeHandleSelector: null, // Enable resize on drag. resizeOnDrag: true, // Toggle between swap and insert modes. swapMode: false, // html for non-contiguous placeholders. nonContiguousCellHtml: null, // Auto add non-contiguous cellson drag or as needed. autoPadNonContiguousCells: true // Enable window resize event handler. updateCoordinatesOnWindowResize: true, // mouseover throttle on drag dragMouseoverThrottle: 150, // window resize debounce windowResizeDebounce: 50, // mouse move debounce mousemoveDebounce: 0, // A function that returns the html of a 'source' cell. getHtmlOfSourceCell: function getHtmlOfSourceCell($cell) { return $cell[0].outerHTML; }, // Debug mode debug: false });
5. API methods.
// attach an element to the grid // element {jQuery/String/Element}: Existing html element to attach to grid. Element must be within the grid's element itself. // index {Number}: Index within the existing ordered visible cell to attach the cell. myGrid.attachCell(element, index); // detach an existing cell from the grid. myGrid.detachCell(element); // get the div that contains the visible cells. myGrid.$getCellContainer(); // get the visible cell at certain coordinates. // clientX {Number}: Coordinate used in a document.elementFromPoint(clientX, clientY); call. // clientY {Number}: Coordinate used in a document.elementFromPoint(clientX, clientY); call. myGrid.$getCellFromCoordinates(clientX, clientY); // get the index/order position of the visible cell at certain coordinates. myGrid.getCellIndexFromCoordinates(clientX, clientY); // get the index/order position of the visible cell. myGrid.getCellIndexOfElement(element); // get the visible cells in their current order. myGrid.$getCells(); // get jQuery object selection of the .closest() visible cell from the element. myGrid.$getCellOfElement(element); // get the hidden cells in their current order. myGrid.$getHiddenCells(); // create a new cell from a html string and attach to grid. // cellHtml {String}: Html to create a new cell. // index {Number}: Index within the existing ordered visible cell to insert the html. myGrid.insertCell(cellHtml, index); // modify an existing visible and/or hidden cell. // cellIndex {Number}: Index of visible to modify. // callback {Function}: Provide a function with two function parameters for getting the visible and then hidden cell. E.g. function ($getVisibleCell, $getHiddenCell) { var $hiddenCell = $getHiddenCell(); }. A call to the first function to retrieve the visible cell will later cause its html to be copied into the hidden cell. Modify the visible cell if the goal is to modify the appearance of the cell. Modify the hidden cell if the goal is to resize or reposition the cell in some way outside of what gridstrap's API provides. myGrid.modifyCell(cellIndex, callback); // move a cell within the grid. // element {jQuery/String/Element}: Existing visible cell element to move. // toIndex {Number}: Index within visible cells to move the element to. // targetGridstrap {Gridstrap} Optional: Instance of a different gridstrap (retrievable from $().data('gridstrap');) to move cell to. Instance must be one that has been previously referenced via setAdditionalGridstrapDragTarget(). myGrid.moveCell(element, toIndex, targetGridstrap); // explicitly append non-contiguous cells to set of current cells. These cells are added automatically as required if the autoPadNonContiguousCells option is enabled, but for performance and behaviour reasons it may be better to setup a grid using this method. // callback {Function}: Provide a function with two Number parameters, cellCount and nonContiguousCellCount. Return true to append a new non-contiguous cell to the grid. The function will be continuously be called until false is returned. myGrid.padWithNonContiguousCells(callback); // detach an existing cell from the grid and then removes it from the DOM. myGrid.removeCell(element); // enable another gridstrap instance to be targetable for drag and moveCell calls. myGrid.setAdditionalGridstrapDragTarget(element); // set a visible cell's position and size. This will also trigger the 'cellredraw' event. // $cell {jQuery/String/Element}: Visible cell Html element. // positionAndSize {Object}: {left:left, top:top, width:width, height:height}. myGrid.setCellAbsolutePositionAndSize($cell, positionAndSize); // update options myGrid.updateOptions(options); // manually trigger a repositioning of the visible cell coordinates to match their respective hidden cells. myGrid.updateVisibleCellCoordinates();
6. Event handlers.
var gs = $('#example').data('gridstrap'); $('#example').on('cellresize', function (e) { // event data // width {Number}: Width of cell. // height {Number}: Height of cell. // target {Element}: Visible cell being resized. }) $('#example').on('cellredraw', function (e) { // event data // left {Number}: Left absolute position of cell. // top {Number}: Top absolute position of cell. // width {Number}: Width of cell. // height {Number}: Height of cell. // target {Element}: Visible cell being redrawn. }) $('#example').on('celldrag', function (e) { // event data // left {Number}: Left offset of mousedown cursor position relative to cell. // top {Number}: Top offset of mousedown cursor position relative to cell. // target {Element}: Visible cell being dragged. })
This awesome jQuery plugin is developed by rosspi. For more Advanced Usages, please check the demo page or visit the official website.