Neat and Clean Modal Plugin with jQuery - wModal
File Size: | 41.1KB |
---|---|
Views Total: | 2270 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
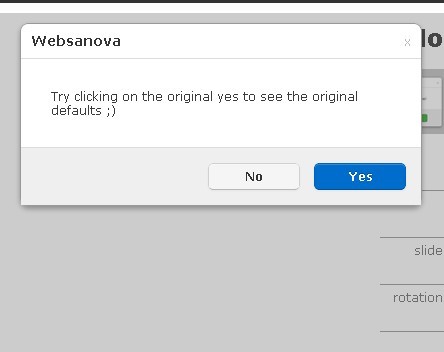
wModal is a jQuery plugin helps create neat and clean Modal dialogs with amazing animations on your web page. Modals can be any piece of html and you can create your own or build one using something like Twitter Boostrap.
Basic Usage:
1. Include jQuery library and wModal in your head section
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script> <script type="text/javascript" src="wModal.js"></script>
2. Include wModal CSS to style your plugin
<link rel="Stylesheet" type="text/css" href="wModal.css" />
3. Javascript (Dynamically load prompts)
<script type="text/javascript"> $.get('./prompts.html', function(data) { $('#templates').html(data); $('#wModal_yesNo').wModal({ btns:{ 'yes': function(){ console.log('original yes'); this.hide(); }, 'no': function(){ this.hide(); }, 'cancel': function(){ this.hide(); } } }); $('#wModal_delete').wModal({ position:'cb', offset:'100', fxShow:'slideUp', fxHide:'slideUp', btns:{ 'delete': function(){ console.log('original delete'); this.hide(); }, 'cancel': function(){ this.hide(); } } }); $('#wModal_ok').wModal({ position:'rb', offset:'30', fxShow:'slideLeft', fxHide:'slideRight', btns:{ 'ok': function(){ console.log('original ok'); this.hide(); }, 'cancel': function(){ this.hide(); } } }); $('#wModal_info').wModal({ position:'lb', offset:'5', fxShow:'slideDown', fxHide:'slideUp', btns:{ 'done': function(){ console.log('original info'); this.hide(); }, 'cancel': function(){ this.hide(); } } }); $('#wModal_exit').wModal({ position:'rm', fxShow:'slideUp', fxHide:'slideDown', btns:{ 'exit': function(){ console.log('original exit'); this.hide(); }, 'cancel': function(){ this.hide(); } } }); $('#wModal_logout').wModal({ position:'rt', offset:'30', fxShow:'slideRight', fxHide:'slideLeft', btns:{ 'logout': function(){ console.log('original logout'); this.hide(); }, 'cancel': function(){ this.hide(); } } }); }); </script>
4. Prompts.html
<script type="text/template" id="wModal_yesNo"> <div class="_wModal _wModal_yesNo"> <div class="_wModal_header"> Websanova <div class="_wModal_close _wModal_btn_cancel">x</div> </div> <div class="_wModal_msg">Are you sure you want to do that?</div> <div class="_wModal_btns_right"> <div class="_wModal_btn _wModal_btn_grey _wModal_btn_no">No</div> <div class="_wModal_btn _wModal_btn_blue _wModal_btn_yes">Yes</div> </div> </div> </script> <script type="text/template" id="wModal_delete"> <div class="_wModal _wModal_delete"> <div class="_wModal_header"> Websanova <div class="_wModal_close _wModal_btn_cancel">x</div> </div> <div class="_wModal_msg">Are you sure you want to delete?</div> <div class="_wModal_btns_right"> <div class="_wModal_btn _wModal_btn_grey _wModal_btn_cancel">Cancel</div> <div class="_wModal_btn _wModal_btn_red _wModal_btn_delete">Delete</div> </div> </div> </script> <script type="text/template" id="wModal_ok"> <div class="_wModal _wModal_yesNo"> <div class="_wModal_header"> Websanova <div class="_wModal_close _wModal_btn_cancel">x</div> </div> <div class="_wModal_msg">You are awesome!</div> <div class="_wModal_btns_center"> <div class="_wModal_btn _wModal_btn_green _wModal_btn_ok">Ok</div> </div> </div> </script> <script type="text/template" id="wModal_info"> <div class="_wModal _wModal_info"> <div class="_wModal_header"> Websanova <div class="_wModal_close _wModal_btn_cancel">x</div> </div> <div class="_wModal_msg">wModal is fantastic!</div> <div class="_wModal_btns_center"> <div class="_wModal_btn _wModal_btn_lightblue _wModal_btn_done">Done</div> </div> </div> </script> <script type="text/template" id="wModal_exit"> <div class="_wModal _wModal_test"> <div class="_wModal_header"> Websanova <div class="_wModal_close _wModal_btn_cancel">x</div> </div> <div class="_wModal_msg">Foo Bar Foo</div> <div class="_wModal_btns_center"> <div class="_wModal_btn _wModal_btn_black _wModal_btn_exit">Exit</div> </div> </div> </script> <script type="text/template" id="wModal_logout"> <div class="_wModal _wModal_logout"> <div class="_wModal_header"> Websanova <div class="_wModal_close _wModal_btn_cancel">x</div> </div> <div class="_wModal_msg">Are you sure you want to logout?</div> <div class="_wModal_btns_right"> <div class="_wModal_btn _wModal_btn_grey _wModal_btn_cancel">Cancel</div> <div class="_wModal_btn _wModal_btn_orange _wModal_btn_logout">Logout</div> </div> </div> </script>
5. Markup html structure and the call the plugin
<input type="button" value="yesNo Original" onclick="$('#wModal_yesNo').wModal('show');"/> <input type="button" value="yesNo Overwrite" onclick="yesNoOverwrite()"/> <input type="button" value="yesNo Multi" onclick="yesNoMulti();"/> <input type="button" value="yesNo Rotate" onclick="yesNoRotate();"/> <input type="button" value="delete" onclick="$('#wModal_delete').wModal('show');"/> <input type="button" value="ok" onclick="$('#wModal_ok').wModal('show');"/> <input type="button" value="info" onclick="$('#wModal_info').wModal('show');"/> <input type="button" value="exit" onclick="$('#wModal_exit').wModal('show');"/> <input type="button" value="logout" onclick="$('#wModal_logout').wModal('show');"/> <script type="text/javascript"> function yesNoOverwrite() { $('#wModal_yesNo').wModal('show', { position:'lt', offset:'50', fxShow:'fade', fxHide:'fade', msg:'Try clicking on the original yes to see the original defaults ;)', btns:{ 'yes': function() { console.log('yes left top'); this.hide(); } } }); } function yesNoMulti() { $('#wModal_yesNo').wModal('show', { position:'rb', fxShow:'slideLeft', fxHide:'slideRight', msg:'Keep pushing the <span style="color:blue;">"Yes"</span> button to see the prompts cycle recursively.', btns:{ 'yes': function() { $('#wModal_info').wModal('show', { btns:{ 'done': function(){ yesNoMulti(); }, 'cancel': function(){ yesNoMulti(); } } }); } } }); } function yesNoRotate() { $('#wModal_yesNo').wModal('show', { fxShow:'rotateLeft', fxHide:'rotateRight' }); } </script> <div id="templates"></div>
6. More Options
$('#_wModal_yesNo').wTooltip({ position : 'cm', // position of modal (lt, ct, rt, rm, rb, cb, lb, lm, cm) offset : '10', // offset of modal from edges if not in "m" or "c" position fxShow : 'none', // show effects (fade, slideUp, slideDown, slideLeft, slideRight, rotateUp, rotateDown, rotateLeft, rotateRight) fxHide : 'none', // hide effects (fade, slideUp, slideDown, slideLeft, slideRight, rotateUp, rotateDown, rotateLeft, rotateRight) btns : {}, // button callbacks msg : null // optional message to set if "_wModal_msg" class is set });
This awesome jQuery plugin is developed by unknown. For more Advanced Usages, please check the demo page or visit the official website.