Display Mixed Media In Lightbox Galleries With jQuery
File Size: | 12.5 KB |
---|---|
Views Total: | 936 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
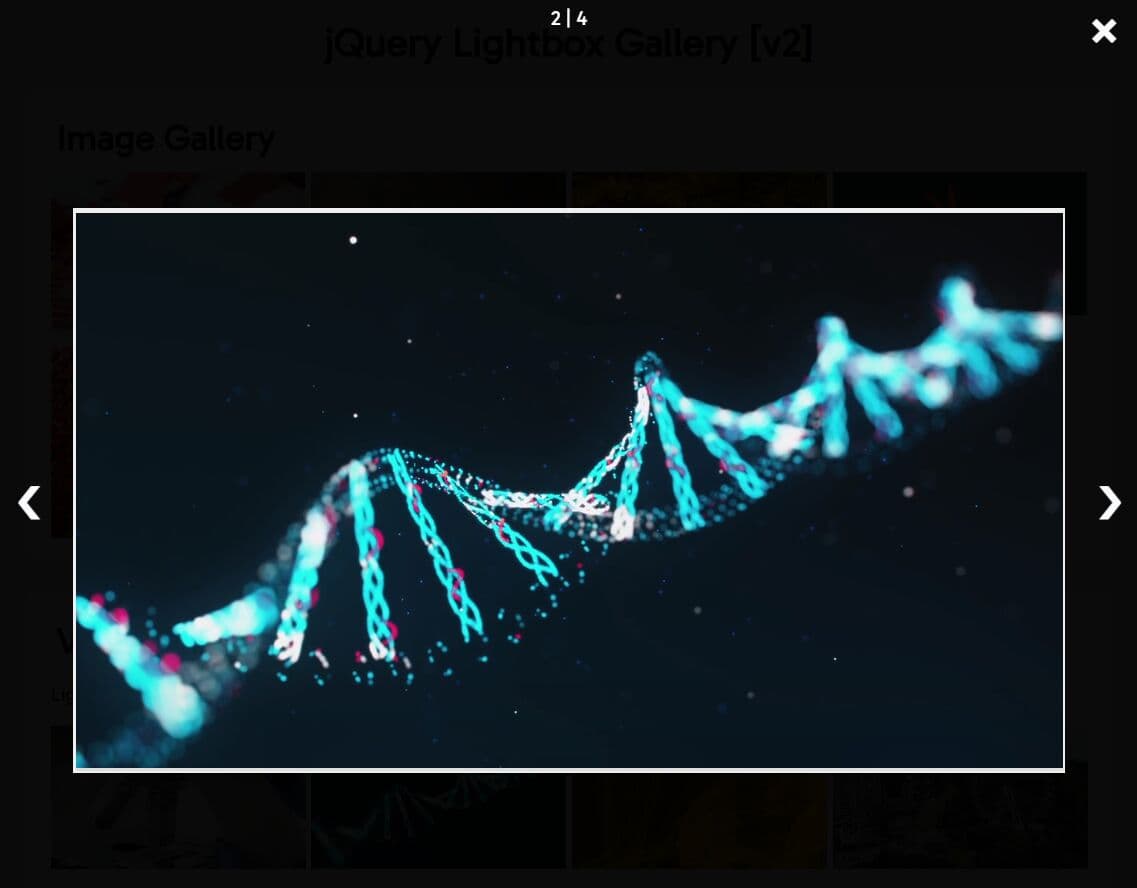
This plugin represents a significant upgrade from its predecessor, the cwa_lightbox. The upgraded lightbox gallery jQuery plugin enables users to display not only images but also videos, iframes, and a mix of these media types.
It works by transforming a simple linked media gallery into a modal-style navigation experience. When users click on a thumbnail, that media pops up in a sleek overlay covering the whole browser window. Next/previous arrows then allow seamless navigation between gallery items. This creates a user-friendly showcase for photos, art, videos, iframe embeds like Youtube videos/Google Maps, and mixed multi-media stories.
How to use it:
1. Load the needed jQuery library and cwa-lightbox.css stylesheet in the document.
<!-- jQuery is required --> <script src="/path/to/cdn/jquery.min.js"></script> <!-- Core Stylesheet --> <link href ="lightbox-css/cwa-lightbox.css" rel ="stylesheet" />
2. Create a lightbox gallery for images:
<section class="image-section"> ... </section>
<script defer src="lightbox-js/cwa-image-lightbox.js"></script>
// Image Array const arrImages = [ { src : "/path/to/image-1/", desc : "Description 1" }, { src : "/path/to/image-2/", desc : "Description 2" }, // more images here ] // Initialize the plugin const imageSectionContainer = $('.image-section'); const imageGallery = $(`<div class="image-gallery"></div>`); $.each(arrImages, function(key, image){ let imageSrc = image.src; let imageLink = $(` <a class="thumbnail-box gallery-images" href="${imageSrc}"> <img src="${imageSrc}" alt="gallery-img_${key}" loading="lazy"/> </a>`); imageLink.on('click', function(e){ e.preventDefault(); e.stopImmediatePropagation(); imageLightbox(arrImages, key); }); imageGallery.append(imageLink); imageSectionContainer.append(imageGallery); });
3. Create a lightbox gallery for videos:
<section class="video-section"> ... </section>
<script defer src="lightbox-js/cwa-video-lightbox.js"></script>
// Video Array const arrVideos = [ { src : "/path/to/video-1/", desc : "Description 1" }, { src : "/path/to/video-2/", desc : "Description 2" }, // more videos here ] // Initialize the plugin const videoSectionContainer = $('.video-section'); const videoGallery = $(`<div class="video-gallery"></div>`); $.each(arrVideos, function(key, video){ let videoSrc = video.src; let videoLink = $(` <a class="thumbnail-box gallery-videos" href="${videoSrc}"> <video src="${videoSrc}" muted></video> </a>`); videoLink.on('click', function(e){ e.preventDefault(); e.stopImmediatePropagation(); videoLightbox(arrVideos, key); }); videoGallery.append(videoLink); videoSectionContainer.append(videoGallery); });
4. Create a lightbox gallery for mixed content:
<section class="media-section"> ... </section> <button id="btn-open-media-lightbox">Open lightbox</button>
<script defer src="lightbox-js/cwa-media-lightbox.js"></script>
// Media Array const arrMedia = [ { src : "/path/to/image", type: "image", desc : "Image Description" }, { src : "/path/to/video", type: "video", desc : "Video Description" }, { src : "/path/to/iframe/", type: "iframe", desc : "Iframe Description" } ] // Initialize the plugin const btnOpenMediaLightbox = $('#btn-open-media-lightbox'); btnOpenMediaLightbox.on('click', function(){ mediaLightbox(arrMedia); });
This awesome jQuery plugin is developed by HashBrownTTM. For more Advanced Usages, please check the demo page or visit the official website.