jQuery Based Progress Button For Async Functions - ProgressButton
File Size: | 158 KB |
---|---|
Views Total: | 2807 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
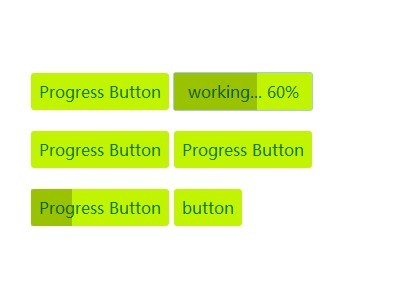
ProgressButton is a simple jQuery plugin that allows you to create a button with built in progress indicator for async functions like content loader, file uploader, etc.
Basic Usage:
1. Include the latest JQuery library and jQuery progressButton plugin in the page.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.0/jquery.min.js"></script> <script src="progressBtn.js"></script>
2. Create a progress button to present an async upload progress.
<div class="container"> <button id="upload" class="progressBtn upload" data-api="plugin-progressBtn">Progress Button</button> </div>
3. Simulating an async upload function
function uploadPic(fileName, cb) { var percent = 0; var intervalID = setInterval(function () { percent = percent + 10; // console.log(percent); cb && cb(percent); if(percent >= 100) { clearInterval(intervalID); percent = 0; } // end of if }, 400); // end of setInterval() }
4. The CSS to style the button and the progress indicator.
.progressBtn { position: relative; z-index: 0; background-color: #c0f400; font-family: sans-serif; border: 0; padding: .5em; font-size: 1em; border-radius: 3px; color: #008000 } .progressBtn .progress-bar { position: absolute; display: block; z-index: 6; top: 0; left: 0; width: 0; height: 100%; background-color: rgba(0,0,0,0.2) } .progressBtn .progress-text { position: absolute; z-index: 5; display: none; top: 0; left: 0; padding: .5em; background-color: #c0f400; width: 100%; heigh: 100%; box-sizing: border-box } .progressBtn:hover { background-color: #b0e000 } .progressBtn:active { background-color: #a3cf00 }
5. Simulating an async upload function
function uploadPic(fileName, cb) { var percent = 0; var intervalID = setInterval(function () { percent = percent + 10; // console.log(percent); cb && cb(percent); if(percent >= 100) { clearInterval(intervalID); percent = 0; } // end of if }, 400); // end of setInterval() } $(document).ready(function(){ console.log('doc ready'); $('.container').on('click','.upload', function(event){ progressBtnObj = $(this).data('plugin-' + 'progressBtn'); console.log(progressBtnObj); var updateProgress = progressBtnObj && progressBtnObj.setProgress; uploadPic('fileNamexxx', $.proxy( updateProgress, progressBtnObj)); }); // end of on() });
This awesome jQuery plugin is developed by nicolasxu. For more Advanced Usages, please check the demo page or visit the official website.