Animated Searchable List View with jQuery and CSS3
File Size: | 4.57 KB |
---|---|
Views Total: | 2440 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
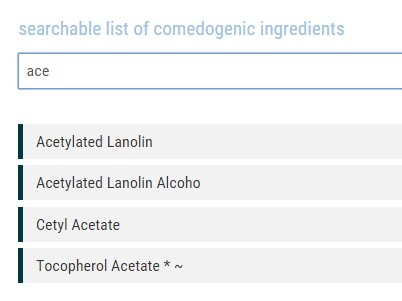
Makes use of jQuery and CSS3 to create an animated filterable list that allows you to dynamically narrow a list of DOM elements with live text search.
How to use it:
1. Create a list of DOM elements as follow.
<ul id="list"> <li>List Item 1</li> <li>List Item 2</li> <li>List Item 3</li> ... <li>List Item n</li> <span class="empty-item">no results</span> </ul>
2. Create a search box to filter the list live.
<input type="text" id="search-text" class="search-box">
3. Create a inline element to output the item count.
<span class="list-count"></span>
4. The core CSS/CSS3 rules for the filterable list.
* { -moz-box-sizing: border-box; -webkit-box-sizing: border-box; box-sizing: border-box; } body { font-family: 'Roboto Condensed', sans-serif; } .list-wrap label { float: left; color: #9bc3e9; font-family: 'Roboto Condensed', sans-serif; font-size: 1.2em; } .search-box { float: left; clear: left; width: 70%; padding: 0.4em; font-size: 1em; color: #555; font-family: 'Roboto Condensed', sans-serif; } .list-count { float: left; text-align: center; width: 30%; padding: 0.5em; color: #ddd; } li { transition-property: margin, background-color, border-color; transition-duration: .4s, .2s, .2s; transition-timing-function: ease-in-out, ease, ease; } .empty-item { transition-property: opacity; transition-duration: 0s; transition-delay: 0s; transition-timing-function: ease; } .empty .empty-item { transition-property: opacity; transition-duration: .2s; transition-delay: .3s; transition-timing-function: ease; } .hiding { margin-left: -100%; opacity: 0.5; } .hidden { display: none; } ul { float: left; width: 100%; margin: 2em 0; padding: 0; position: relative; } li { float: left; clear: left; width: 100%; margin: 0.2em 0; padding: 0.5em 0.8em; list-style: none; background-color: #f2f2f2; border-left: 5px solid #003842; cursor: pointer; color: #333; position: relative; z-index: 2; font-family: 'Roboto Condensed', sans-serif; } li:hover { background-color: #f9f9f9; border-color: #9bc3e9; } .empty-item { background: #fff; color: #ddd; margin: 0.2em 0; padding: 0.5em 0.8em; font-style: italic; border: none; text-align: center; visibility: hidden; opacity: 0; float: left; clear: left; width: 100%; } .empty .empty-item { opacity: 1; visibility: visible; }
5. Load the latest version of jQuery JavaScript library at the end of the document.
<script src="//ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
6. Enable the live search on the list.
$(document).ready(function() { var jobCount = $('#list .in').length; $('.list-count').text(jobCount + ' items'); $("#search-text").keyup(function () { var searchTerm = $("#search-text").val(); var listItem = $('#list').children('li'); var searchSplit = searchTerm.replace(/ /g, "'):containsi('") $.extend($.expr[':'], { 'containsi': function(elem, i, match, array) { return (elem.textContent || elem.innerText || '').toLowerCase() .indexOf((match[3] || "").toLowerCase()) >= 0; } }); $("#list li").not(":containsi('" + searchSplit + "')").each(function(e) { $(this).addClass('hiding out').removeClass('in'); setTimeout(function() { $('.out').addClass('hidden'); }, 300); }); $("#list li:containsi('" + searchSplit + "')").each(function(e) { $(this).removeClass('hidden out').addClass('in'); setTimeout(function() { $('.in').removeClass('hiding'); }, 1); }); var jobCount = $('#list .in').length; $('.list-count').text(jobCount + ' items'); if(jobCount == '0') { $('#list').addClass('empty'); } else { $('#list').removeClass('empty'); } }); function searchList() { var listArray = []; $("#list li").each(function() { var listText = $(this).text().trim(); listArray.push(listText); }); $('#search-text').autocomplete({ source: listArray }); } searchList(); });
This awesome jQuery plugin is developed by k8k. For more Advanced Usages, please check the demo page or visit the official website.