Flat Animated To-do List with jQuery and Animate.css
File Size: | 2.1 KB |
---|---|
Views Total: | 4737 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
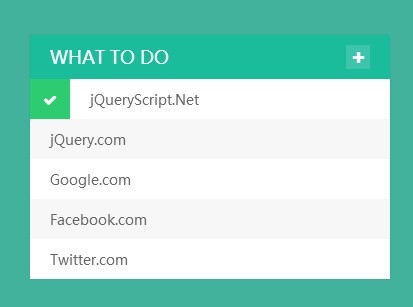
An animated and flat designed to-do list app built on top of jQuery, animate.css and CSS3 transitions. Hover over the list and click on the 'check' icon to remove a task. Click on the '+' icon to add a new task.
How to use it:
1. Add the required Font Awesome and animate.css in the head section of the document.
<link href="//netdna.bootstrapcdn.com/font-awesome/4.1.0/css/font-awesome.min.css" rel="stylesheet"> <link href="//cdnjs.cloudflare.com/ajax/libs/animate.css/3.1.1/animate.min.css" rel="stylesheet">
2. Create a to-do list with predefined tasks using Html unordered lists.
<div class="list animated tada"> <h1> What To Do <a class="add" href="#"> <div class="fa fa-plus"></div> </a> </h1> <ul> <li> <a href="#"> <div class="fa fa-check"></div> </a> Task 1 </li> <li> <a href="#"> <div class="fa fa-check"></div> </a> Task 2 </li> <li> <a href="#"> <div class="fa fa-check"></div> </a> Task 3 </li> <li> <a href="#"> <div class="fa fa-check"></div> </a> Task 4 </li> <li> <a href="#"> <div class="fa fa-check"></div> </a> Task 5 </li> </ul> </div>
3. Some CSS/CSS3 rules to style & animate the to-do list.
.list { position: absolute; width: 360px; top: 50%; left: 50%; margin: -122px 0 100px -180px; -webkit-box-shadow: 0 0 3px rgba(0, 0, 0, 0.1); -moz-box-shadow: 0 0 3px rgba(0, 0, 0, 0.1); box-shadow: 0 0 3px rgba(0, 0, 0, 0.1); } .list h1 { position: relative; background: #1abc9c; color: #fff; margin: 0; padding: 10px 20px; font-family: "Roboto"; font-size: 18px; text-transform: uppercase; font-weight: 400; } .list h1 a { position: absolute; top: 50%; right: 20px; background: #fff; background: rgba(255, 255, 255, 0.15); width: 24px; height: 24px; margin: -12px 0 0 0; color: #fff; font-size: 14px; line-height: 24px; text-align: center; text-decoration: none; -webkit-transition: 0.3s linear; -moz-transition: 0.3s linear; -ms-transition: 0.3s linear; -o-transition: 0.3s linear; transition: 0.3s linear; } .list h1 a:hover { background: rgba(255, 255, 255, 0.2); } .list ul { list-style: none; margin: 0; padding: 0; } .list ul li { background: #fff; height: 40px; color: #666; line-height: 40px; padding: 0 20px 0 0; } .list ul li a { display: inline-block; background: #2ecc71; width: 0px; height: 40px; margin-right: 16px; color: #fff; text-align: center; text-decoration: none; opacity: 0; -webkit-transition: 0.2s linear; -moz-transition: 0.2s linear; -ms-transition: 0.2s linear; -o-transition: 0.2s linear; transition: 0.2s linear; } .list ul li:nth-child(2n) { background: #f7f7f7; } .list ul li:hover a { width: 40px; opacity: 1; }
4. Add the necessary JQuery library at the bottom for faster page loading.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
5. The Javascript to active the to-do list and customize the randomized tasks.
$( ".add" ).click(function(event) { var items = Array( "My task 1", "My task 2", "My task 3"); var item = items[Math.floor(Math.random()*items.length)]; $("ul").add( "<li><a href='#'><div class='fa fa-check'></div></a> " + item + "</li>" ).fadeIn().appendTo("ul"); shuffle(item); }); $( "ul > li > a" ).click(function() { $(this).parent( "li" ).slideUp(200); });
This awesome jQuery plugin is developed by andytran. For more Advanced Usages, please check the demo page or visit the official website.