Horizontal & Vertical Scroll Progress Bars In jQuery
File Size: | 6.64 KB |
---|---|
Views Total: | 3667 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
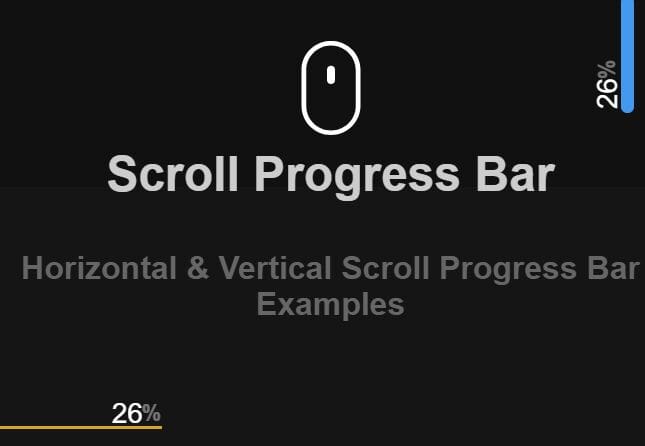
A jQuery script to create a horizontal or vertical progress bar that fills up as the user scrolls down the webpage.
Intended to create a visual reading progress indicator that keeps track of the current reading position of an article/post/page.
How to use it:
1. Create a container to hold the scroll progress bar.
<div class="scrollBar"> <span></span> </div>
2. The CSS styles for the scroll progress bar.
/* horizontal scroll bar */ .scrollBar { position: fixed; top: 95vh; height: 2px; width: 0%; background: goldenrod; -webkit-transition: width 200ms ease; transition: width 200ms ease; text-align: right; color: #fff; } .scrollBar span { position: absolute; top: -23px; right: 15px; font-size: 0.7em; font-weight: 500; display: block; text-align: center; } .scrollBar span::after { content: "%"; font-size: 0.8em; position: absolute; right: -15px; bottom: 4px; font-weight: 700; opacity: 0.4; } /* vertical scroll bar */ .scrollBar { position: fixed; top: 0; right: 2vw; height: 0%; width: 10px; background: #49e; -webkit-transition: height 200ms ease; transition: height 200ms ease; text-align: right; color: #fff; display: -webkit-box; display: flex; -webkit-box-align: center; align-items: center; border-radius: 1em; } .scrollBar span { position: absolute; bottom: 3px; left: 2px; font-size: 0.7em; font-weight: 500; display: inline-block; text-align: left; -webkit-transform: rotate(-90deg); transform: rotate(-90deg); -webkit-transform-origin: bottom right; transform-origin: bottom left; } .scrollBar span::after { content: "%"; font-size: 0.8em; position: absolute; right: -15px; bottom: 4px; font-weight: 700; opacity: 0.4; }
3. Load the necessary jQuery library at the end of the document.
<script src="/path/to/cdn/jquery.slim.min.js"></script>
4. The jQuery script to keep track of the scroll position (the whole document in this example) and update the progress bar on vertical page scroll.
$(document).scroll(function (e) { // main function var scrollAmount = $(window).scrollTop(); var documentHeight = $(document).height(); var windowHeight = $(window).height(); var scrollPercent = (scrollAmount / (documentHeight - windowHeight)) * 100; var roundScroll = Math.round(scrollPercent); // horizontal scroll bar $(".scrollBar").css("width", scrollPercent + "%"); $(".scrollBar span").text(roundScroll); // vertical scroll bar $(".scrollBar").css("height", scrollPercent + "%"); $(".scrollBar span").text(roundScroll); });
This awesome jQuery plugin is developed by EricPorter. For more Advanced Usages, please check the demo page or visit the official website.