Mouse Follow Scroll Position Indicator With JavaScript And CSS
File Size: | 2.81 KB |
---|---|
Views Total: | 1817 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
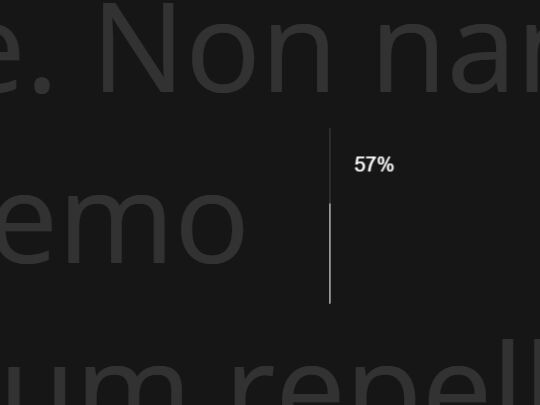
A slim vertical progress bar with percentage value that always follows the cursor to indicate the user's current scroll position on a webpage.
Written in jQuery, TweenMax.js, and CSS/CSS3. Can be used as a reading progress indicator that fills up as the user scrolls down or up your webpage.
How to use it:
1. Create the HTML for the scroll progress indicator & counter.
<div class="percent"> <div class="fill"></div> <div class="counter"> <h2></h2> </div> </div>
2. The necessary CSS/CSS3 styles.
.fill { position: absolute; bottom: 0; width: 100%; height: 100%; background: #fff; transition: background 0.15s ease; } .percent { position: fixed; display: flex; flex-direction: column; background: rgba(255, 255, 255, 0.2); height: 140px; width: 1px; margin: -60px 0 0 40px; }
3. Load the needed jQuery and TweenMax.js libraries in the document.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/cdn/TweenMax.min.js"></script>
4. Load the needed jQuery and TweenMax.js libraries in the document.
var counter = document.querySelector(".percent"); TweenLite.set(counter, { xPercent: -5, yPercent: -5, }); // mouse follow window.addEventListener("mousemove", moveCounter); function moveCounter(e) { TweenLite.to(counter, 0.5, { x: e.clientX, y: e.clientY, }); } // main function function progress() { var windowScrollTop = $(window).scrollTop(); var docHeight = $(document).height(); var windowHeight = $(window).height(); var progress = (windowScrollTop / (docHeight - windowHeight)) * 100; var $bgColor = progress > 99 ? "#fff" : "#fff"; var $textColor = progress > 99 ? "#fff" : "#333"; // update progress bar $("h2") .text(Math.round(progress) + "%") .css({ color: $textColor }); $(".fill") .height(progress + "%") .css({ backgroundColor: $bgColor }); } // keep track of scroll position progress(); $(document).on("scroll", progress);
This awesome jQuery plugin is developed by codegridweb. For more Advanced Usages, please check the demo page or visit the official website.