PDF Annotation And Drawing Markup Plugin - pdfannotate.js
File Size: | 1.34 MB |
---|---|
Views Total: | 14428 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
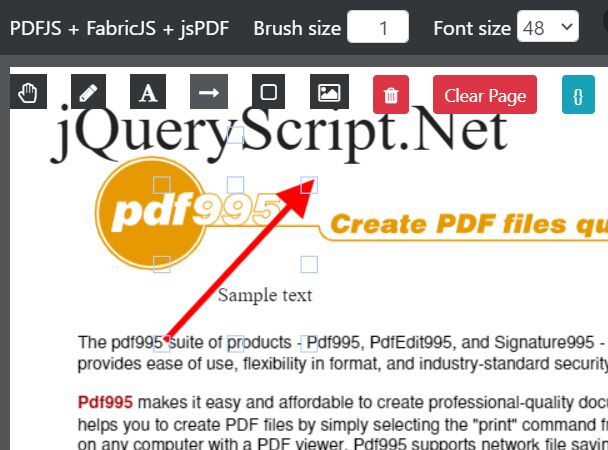
An online PDF Annotation jQuery plugin that allows your users to write comments, insert images, leave notes, and draw lines & shapes (like arrows, rectangles) on a PDF file.
More Features:
- Export & Import annotation data from JSON.
- Custom border color and border size for rectangle tool.
- Custom brush size for pencil tool.
- Custom font size for text tool.
- Export PDF with annotations.
Dependencies:
How to use it:
1. Load the required resources in the document.
<!-- jQuery --> <script src="/path/to/cdn/jquery.min.js"></script> <!-- Bootstrap 4 --> <link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <script src="/path/to/cdn/bootstrap.min.js"></script> <!-- Font Awesome --> <link rel="stylesheet" href="/path/to/cdn/font-awesome.min.css" /> <!-- PDF.js --> <script src="/path/to/cdn/pdf.min.js"></script> <script>pdfjsLib.GlobalWorkerOptions.workerSrc = '/path/to/cdn/pdf.worker.min.js';</script> <!-- fabric.js --> <script src="/path/to/cdn/fabric.min.js"></script> <script src="./arrow.fabric.js"></script> <!-- jspdf.js --> <script src="/path/to/cdn/jspdf.umd.min.js"></script>
2. Load the pdfannotate.js plugin's files into the document.
<!-- Core Stylesheet --> <link rel="stylesheet" href="./pdfannotate.css"> <!-- Custom Styles --> <link rel="stylesheet" href="./styles.css"> <!-- Core JavaScript --> <script src="./pdfannotate.js"></script>
3. Create a container to hold the PDF viewer.
<div id="pdf-container"></div>
4. Initialize the plugin and load a PDF file into the container.
var pdf = new PDFAnnotate("pdf-container", "pdf.pdf", { // options here });
5. Enable & config the PDF Annotation And Drawing Markup tools.
// enable moving tool pdf.enableSelector(); // Enable pencil tool pdf.enablePencil(); // Enable comment/note tool pdf.enableAddText(); // Enable arrow tool pdf.enableAddArrow(); // Enable rectangle tool pdf.enableRectangle(); // Add an image to the PDF pdf.addImageToCanvas(); // Delete the selected annotation pdf.deleteSelectedObject(); // Remove all annotations pdf.clearActivePage(); // Clear current page // Save the PDF with annotations pdf.savePdf(); // Serialize PDF annotation data pdf.serializePdf(); // Load annotations from JSON pdf.loadFromJSON(serializedJSON); // Set color for tools pdf.setColor(color); // Set border color for rectangle tool pdf.setBorderColor(color); // Set brush size for pencil tool pdf.setBrushSize(width); // Set font size for text tool pdf.setFontSize(font_size); // Set border size of rectangles pdf.setBorderSize(border_size);
6. Available options and callback functions.
var pdf = new PDFAnnotate("pdf-container", "pdf.pdf", { onPageUpdated(page, oldData, newData) { console.log(page, oldData, newData); }, ready() { console.log("Plugin initialized successfully"); }, scale: 1.5, pageImageCompression: "MEDIUM", // FAST, MEDIUM, SLOW });
This awesome jQuery plugin is developed by RavishaHesh. For more Advanced Usages, please check the demo page or visit the official website.