Horizontal Scroll Jacking Effect with jQuery and CSS3
File Size: | 3.47 KB |
---|---|
Views Total: | 6203 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
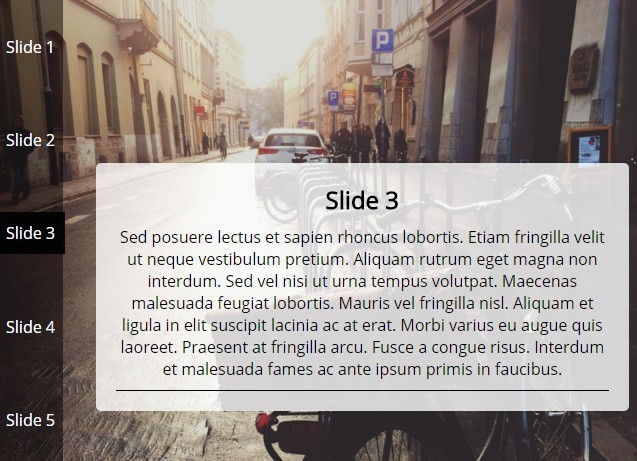
An infinite fullscreen horizontal page slider which enables you present and navigate through web content with a fancy "Scroll-Jacking" effect. Also can be used in your one page scrolling website to present web content with mouse wheel. Built with jQuery, CSS / CSS3 and jQuery mousewheel plugin.
How to use it:
1. Create sectioned web content you want to present as displayed below.
<div class="slides"> <div id="1" class="slide"> <div class="content"> <h2>Slide 1</h2> </div> </div> <div id="2" class="slide"> <div class="content"> <h2>Slide 2</h2> </div> </div> <div id="3" class="slide"> <div class="content"> <h2>Slide 3</h2> </div> </div> ... </div>
2. Create a slider navigation that allows the visitor to navigate through your web content manually.
<nav> <ul> <li><a href="#1">Slide 1</a></li> <li><a href="#2">Slide 2</a></li> <li><a href="#3">Slide 3</a></li> ... </ul> </nav>
3. The core CSS / CSS3 styles for the slider navigation and content sections.
nav { text-align: center; background: rgba(0, 0, 0, 0.5); position: fixed; z-index: 100; height: 100vh; left: 0; width: 10vw; font-weight: 300; font-size: 1rem; } nav ul { list-style-type: none; height: 100vh; display: -webkit-box; display: -webkit-flex; display: -ms-flexbox; display: flex; -webkit-box-orient: vertical; -webkit-box-direction: normal; -webkit-flex-direction: column; -ms-flex-direction: column; flex-direction: column; -webkit-justify-content: space-around; -ms-flex-pack: distribute; justify-content: space-around; } nav a, nav a:visited, nav a:active { text-decoration: none; color: #fff; } nav a { -webkit-transition: color 2s, background 1s; transition: color 2s, background 1s; padding: 10px; position: relative; z-index: 0; } nav a.active { background: rgba(0, 0, 0, 0.9); } nav a::before { content: ""; position: absolute; height: 0%; width: 0%; bottom: 0; left: 0; opacity: 1; background: #555; z-index: -1; } nav a:hover { -webkit-animation: fillColour 0.7s forwards ease-in-out; animation: fillColour 0.7s forwards ease-in-out; } nav a:hover::before { -webkit-animation: fill 0.7s forwards ease-in-out; animation: fill 0.7s forwards ease-in-out; opacity: 1; } .slides { width: 500vw; height: 100vh; -webkit-transition: -webkit-transform 0.8s ease; transition: transform 0.8s ease; } .slides .slide { height: 100vh; width: 100vw; background: pink; float: left; text-align: center; background-size: cover; } .slides .slide .content { width: 80vw; padding: 20px; background: rgba(255, 255, 255, 0.8); margin: 35vh 0 0 15vw; border-radius: 5px; } .slides .slide .content p { padding: 10px 0; border-bottom: 1px solid black; } @-webkit-keyframes fill { 0% { width: 0%; height: 1px; } 50% { width: 100%; height: 1px; } 100% { width: 100%; height: 100%; background: #fff; } } @keyframes fill { 0% { width: 0%; height: 1px; } 50% { width: 100%; height: 1px; } 100% { width: 100%; height: 100%; background: #fff; } } @-webkit-keyframes fillColour { 0% { color: #fff; } 50% { color: #fff; } 100% { color: #333; } } @keyframes fillColour { 0% { color: #fff; } 50% { color: #fff; } 100% { color: #333; } }
4. Load the necessary jQuery library and jQuery mousewheel plugin at the bottom of the webpage.
<script src="/path/to/jquery-2.1.4.min.js"></script> <script src="/path/to/jquery.mousewheel.min.js"></script>
5. The core JavaScript to active the scroll jacking effect on horizontal scroll.
$(function () { function showSlide(n) { $('body').unbind('mousewheel'); currSlide += n; currSlide = currSlide <= 0 ? 0 : currSlide >= $slide.length - 1 ? $slide.length - 1 : currSlide; var displacment = window.innerWidth * currSlide; $('.slides').css('transform', 'translateX(-' + displacment + 'px)'); setTimeout(function () { $('body').bind('mousewheel', mouseEvent); }, 800); $('nav a.active').removeClass('active'); $($('a')[currSlide]).addClass('active'); } function mouseEvent(e, delta) { showSlide(delta >= 0 ? 1 : -1); e.preventDefault(); } $('nav a').click(function (e) { var newslide = parseInt($(this).attr('href')[1]); var diff = newslide - currSlide - 1; showSlide(diff); e.preventDefault(); }); $(window).resize(function () { var displacment = window.innerWidth * currSlide; $('.slides').css('transform', 'translateX(-' + displacment + 'px)'); }); var currSlide = 0; var $slide = $('.slide'); $($('nav a')[0]).addClass('active'); $('body').bind('mousewheel', mouseEvent); });
This awesome jQuery plugin is developed by jaytauron. For more Advanced Usages, please check the demo page or visit the official website.