One Page Scroll With Parallax Effect - FullPageScroll
File Size: | 3.15 KB |
---|---|
Views Total: | 2023 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
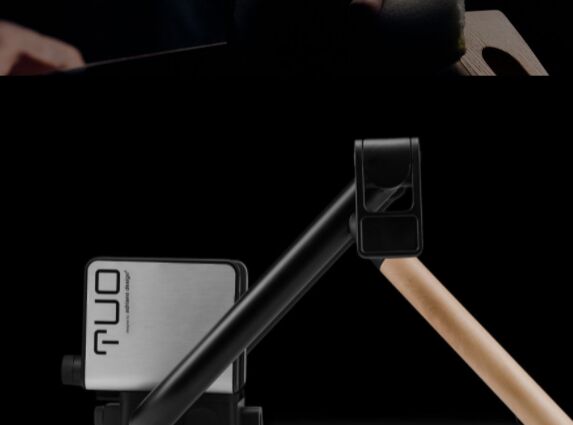
A fullscreen, touch-enabled vertical page slider that listens for Wheel/Keydown/Touch events and slides between content sections with a subtle parallax effect.
Well-suited for one page scrolling website, single page web app, full-page product presentation, and much more. Keyboard navigation (up/down keys) is supported as well.
See Also:
How to use it:
1. Add content sections as slides to the page slider.
<div class="slider"> <div class="slide active"> <div class="bg"></div> </div> <div class="slide queue"> <div class="bg"></div> </div> <div class="slide queue"> <div class="bg"></div> </div> <div class="slide queue"> <div class="bg"></div> </div> </div>
2. The main styles for the page slider.
/* Make the slider fullscreen */ html, body, .slider, .slide, .bg { margin: 0; width: 100%; height: 100%; overflow: hidden; } /* Main styles */ .slider { background: #000; } .slide { background: #000; position: absolute; top: 0; left: 0; transform: translate3d(0,0,0); transition: 800ms; } .bg { background-position: center; background-repeat: no-repeat; background-size: cover; transition: 800ms; } /* Transitions */ .queue { transform: translate3d(0, 100%, 0); } .prev { transform: translate3d(0,-50%,0); } .prev .bg { opacity: 0.5; } .bounce { transition: 300ms; } .slide:first-child.bounce { transform: translate3d(0,10%,0); } .slide:last-child.bounce { transform: translate3d(0,-10%,0); }
3. Add background images to your content.
.slide:nth-child(1) .bg { background-image: url(1.jpg); } .slide:nth-child(2) .bg { background-image: url(2.jpg); } .slide:nth-child(3) .bg { background-image: url(3.jpg); } .slide:nth-child(4) .bg { background-image: url(4.jpg); }
4. The main JavaScript to enable the page slider. Copy and paste the following JS snippets after jQuery library and you're done.
<script src="/path/to/cdn/jquery.min.js"></script>
$(document).ready(function () { var timeStart = 0; function wheely(e) { var y = e.originalEvent.deltaY; var timeStop = new Date().getTime(); var timeDiff = timeStop - timeStart; if (timeDiff > 200) { if (y > 0) { nextSlide(); } if (y < 0) { prevSlide(); } } timeStart = timeStop; } function prevSlide() { if ($(".active").is(":first-child")) { if (!$(".slider").hasClass("dragging")) { $(".slide:first-child").addClass("bounce"); setTimeout(function () { $(".slide:first-child").removeClass("bounce"); }, 300); } } else { $(".active") .removeClass("active") .addClass("queue") .prev() .removeClass("prev") .addClass("active"); } } function nextSlide() { if ($(".active").is(":last-child")) { if (!$(".slider").hasClass("dragging")) { $(".slide:last-child").addClass("bounce"); setTimeout(function () { $(".slide:last-child").removeClass("bounce"); }, 300); } } else { $(".active") .removeClass("active") .addClass("prev") .next() .removeClass("queue") .addClass("active"); } } function hotkeys(e) { if (e.keyCode == 38) { prevSlide(); } if (e.keyCode == 40) { e.preventDefault(); nextSlide(); } } function dragStart(e) { e.preventDefault(); if (e.type == "touchstart") { $(document).off("mousedown", dragStart); startPoint = e.originalEvent.touches[0].pageY; } else { startPoint = e.pageY; } dragDist = 0; $(document).on("touchmove mousemove", dragMove); } function dragMove(e) { if (e.type == "touchmove") { var movePoint = e.originalEvent.touches[0].pageY; } else { var movePoint = e.pageY; } dragDist = ((movePoint - startPoint) / $(".slider").height()) * 100; $(".slider").addClass("dragging"); $(".slide, .bg").css({ transition: "0ms", }); if (dragDist < 0) { $(".active .bg").css({ opacity: 1 + dragDist / 200, }); $(".active") .css({ transform: "translate3d(0," + dragDist / 2 + "%,0)", }) .next() .css({ transform: "translate3d(0," + (100 + dragDist) + "%,0)", }); } if (dragDist > 0) { $(".active") .css({ transform: "translate3d(0," + dragDist + "%,0)", }) .prev() .css({ animation: "none", transform: "translate3d(0," + (-50 + dragDist / 2) + "%,0)", }) .find(".bg") .css({ opacity: 0.5 + dragDist / 200, }); } } function dragEnd() { $(document).off("touchmove mousemove", dragMove); $(".slide, .bg").removeAttr("style"); if (dragDist > 20) { prevSlide(); } if (dragDist < -20) { nextSlide(); } setTimeout(function () { $(".slider").removeClass("dragging"); }, 800); } $(document).on("wheel", wheely); $(document).on("keydown", hotkeys); $(document).on("touchstart mousedown", dragStart); $(document).on("touchend mouseup", dragEnd); });
This awesome jQuery plugin is developed by Mishka-Sakhelashvili. For more Advanced Usages, please check the demo page or visit the official website.