Responsive Smooth Card Carousel With jQuery And CSS3
File Size: | 2.41 KB |
---|---|
Views Total: | 70839 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
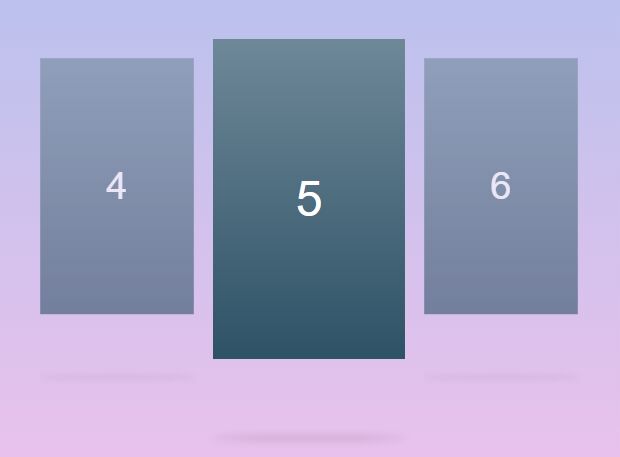
A simple, responsive card slider/carousel component written in JavaScript (jQuery) and CSS/CSS3. It enables the user to slide through a group of cards by clicking slides or pressing left & right arrow keys.
It also has the ability to enlarge the current slide while sliding through cards. Smooth transition effects based on CSS3 transform and transition properties.
How to use it:
1. Add a group slides to the card carousel.
<div class="card-carousel"> <div class="my-card"></div> <div class="my-card"></div> <div class="my-card"></div> <div class="my-card"></div> <div class="my-card"></div> ... </div>
2. The primary CSS/CSS3 styles for the card carousel.
.card-carousel { display: flex; align-items: center; justify-content: center; position: relative; } .card-carousel .my-card { height: 20rem; width: 12rem; position: relative; z-index: 1; -webkit-transform: scale(0.6) translateY(-2rem); transform: scale(0.6) translateY(-2rem); opacity: 0; cursor: pointer; pointer-events: none; background: #2e5266; background: linear-gradient(to top, #2e5266, #6e8898); transition: 1s; } .card-carousel .my-card:after { content: ''; position: absolute; height: 2px; width: 100%; border-radius: 100%; background-color: rgba(0,0,0,0.3); bottom: -5rem; -webkit-filter: blur(4px); filter: blur(4px); } .card-carousel .my-card.active { z-index: 3; -webkit-transform: scale(1) translateY(0) translateX(0); transform: scale(1) translateY(0) translateX(0); opacity: 1; pointer-events: auto; transition: 1s; } .card-carousel .my-card.prev, .card-carousel .my-card.next { z-index: 2; -webkit-transform: scale(0.8) translateY(-1rem) translateX(0); transform: scale(0.8) translateY(-1rem) translateX(0); opacity: 0.6; pointer-events: auto; transition: 1s; }
3. Apply your own CSS styles to the slides.
.card-carousel .my-card:nth-child(0):before { content: '0'; position: absolute; top: 50%; left: 50%; -webkit-transform: translateX(-50%) translateY(-50%); transform: translateX(-50%) translateY(-50%); font-size: 3rem; font-weight: 300; color: #fff; } .card-carousel .my-card:nth-child(1):before { content: '1'; position: absolute; top: 50%; left: 50%; -webkit-transform: translateX(-50%) translateY(-50%); transform: translateX(-50%) translateY(-50%); font-size: 3rem; font-weight: 300; color: #fff; } .card-carousel .my-card:nth-child(2):before { content: '2'; position: absolute; top: 50%; left: 50%; -webkit-transform: translateX(-50%) translateY(-50%); transform: translateX(-50%) translateY(-50%); font-size: 3rem; font-weight: 300; color: #fff; } .card-carousel .my-card:nth-child(3):before { content: '3'; position: absolute; top: 50%; left: 50%; -webkit-transform: translateX(-50%) translateY(-50%); transform: translateX(-50%) translateY(-50%); font-size: 3rem; font-weight: 300; color: #fff; } .card-carousel .my-card:nth-child(4):before { content: '4'; position: absolute; top: 50%; left: 50%; -webkit-transform: translateX(-50%) translateY(-50%); transform: translateX(-50%) translateY(-50%); font-size: 3rem; font-weight: 300; color: #fff; }
4. Import the necessary jQuery JavaScript library into the document.
<script src="https://code.jquery.com/jquery-3.3.1.min.js" integrity="sha384-tsQFqpEReu7ZLhBV2VZlAu7zcOV+rXbYlF2cqB8txI/8aZajjp4Bqd+V6D5IgvKT" crossorigin="anonymous"> </script>
5. The main JavaScript (jQuery script) to activate the card carousel.
$num = $('.my-card').length; $even = $num / 2; $odd = ($num + 1) / 2; if ($num % 2 == 0) { $('.my-card:nth-child(' + $even + ')').addClass('active'); $('.my-card:nth-child(' + $even + ')').prev().addClass('prev'); $('.my-card:nth-child(' + $even + ')').next().addClass('next'); } else { $('.my-card:nth-child(' + $odd + ')').addClass('active'); $('.my-card:nth-child(' + $odd + ')').prev().addClass('prev'); $('.my-card:nth-child(' + $odd + ')').next().addClass('next'); } $('.my-card').click(function() { $slide = $('.active').width(); console.log($('.active').position().left); if ($(this).hasClass('next')) { $('.card-carousel').stop(false, true).animate({left: '-=' + $slide}); } else if ($(this).hasClass('prev')) { $('.card-carousel').stop(false, true).animate({left: '+=' + $slide}); } $(this).removeClass('prev next'); $(this).siblings().removeClass('prev active next'); $(this).addClass('active'); $(this).prev().addClass('prev'); $(this).next().addClass('next'); }); // Keyboard nav $('html body').keydown(function(e) { if (e.keyCode == 37) { // left $('.active').prev().trigger('click'); } else if (e.keyCode == 39) { // right $('.active').next().trigger('click'); } });
This awesome jQuery plugin is developed by Collin Smith. For more Advanced Usages, please check the demo page or visit the official website.