Minimalist Table Sorter In jQuery
File Size: | 4.13 KB |
---|---|
Views Total: | 290 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
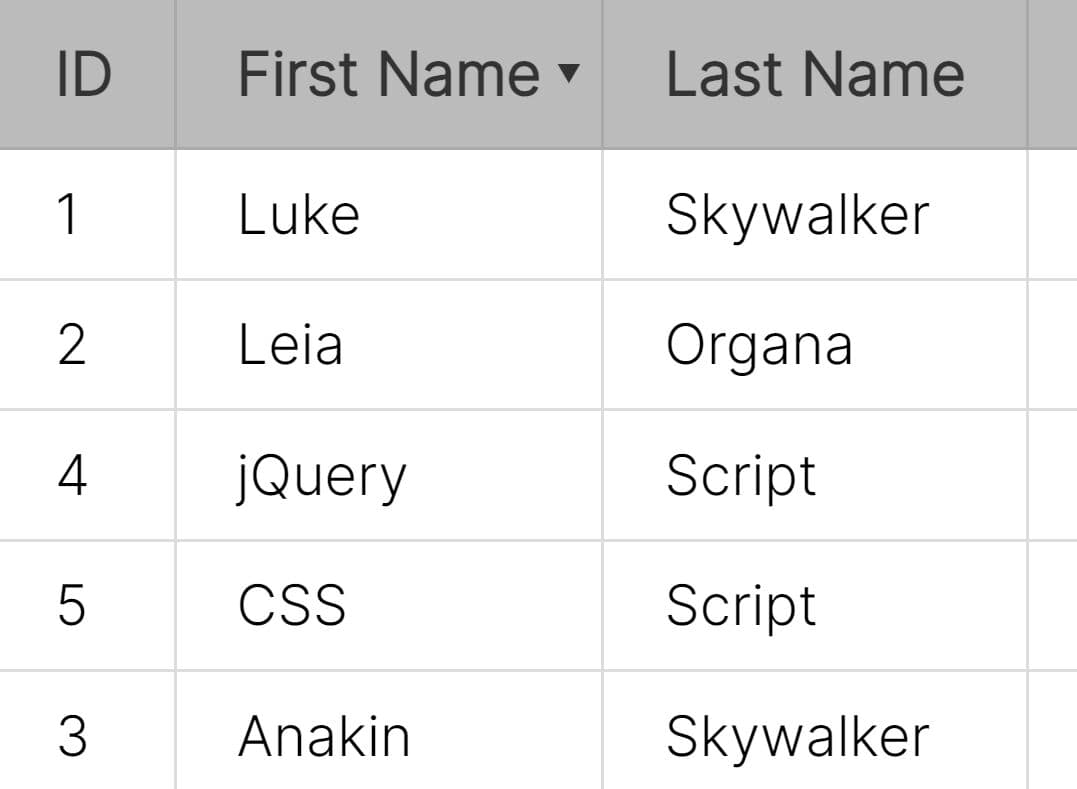
This is a jQuery-powered table sorter that allows users to instantly sort the table rows in ascending or descending order, based on the selected column's data type (alphabetical for text, numerical for numbers, or chronological for dates).
Despite being built on jQuery, it's incredibly lightweight and fast. It utilizes only around 1kb (uncompressed) of jQuery code to implement the sorting functionality that other jQuery plugins or JavaScript libraries often require several KB to achieve.
How to use it:
1. Make sure your table has a <thead>
element. You can also ignore the table sorting functionality on specific table columns by using the no-sort
class as follows:
<table> <thead> <tr> <th>ID</th> <th>First Name</th> <th>Last Name</th> <th class="no-sort">Comment</th> </tr> </thead> <tbody> Tabular data here </tbody> </table>
2. Load the necessary jQuery library at the bottom of the page.
<script src="/path/to/cdn/jquery.slim.min.js"></script>
3. This jQuery code sets up an event listener that triggers the sorting functionality when a user clicks on a table header cell (except those marked with the .no-sort
class). The subsequent code handles the intricate details of sorting the table rows based on the selected column's data type.
$(document).ready(function() { $(document).on("click", "table thead tr th:not(.no-sort)", function() { let table = $(this).parents("table"); let rows = $(this).parents("table").find("tbody tr").toArray().sort(TableComparer($(this).index())); let dir = ($(this).hasClass("sort-asc")) ? "desc" : "asc"; if (dir == "desc") { rows = rows.reverse(); } for (let i = 0; i < rows.length; i++) { table.append(rows[i]); } table.find("thead tr th").removeClass("sort-asc").removeClass("sort-desc"); $(this).removeClass("sort-asc").removeClass("sort-desc") .addClass("sort-" + dir); }); });
4. The TableComparer
function is the core of the sorting algorithm. It takes the column index as a parameter and returns a comparison function that can be used with the `sort` method. This function compares two table rows (`a` and `b`) based on the values in the specified column index.
function TableComparer(index) { return function (a, b) { let val_a = TableCellValue(a, index).replace(/\$\,/g, ""); let val_b = TableCellValue(b, index).replace(/\$\,/g, ""); let result = val_a.toString().localeCompare(val_b); if ($.isNumeric(val_a) && $.isNumeric(val_b)) { result = val_a - val_b; } if (isDate(val_a) && isDate(val_b)) { let date_a = new Date(val_a); let date_b = new Date(val_b); result = date_a - date_b; } return result; } }
5. The TableCellValue
function retrieves the textual value of a specific cell within a table row, given the row element and the column index.
function TableCellValue(row, index) { return $(row).children("td").eq(index).text(); }
6. The isDate
function is a helper function that determines whether a given value represents a valid date or not. If true, it then enables the sorting algorithm to handle date values appropriately.
function isDate(val) { let d = new Date(val); return !isNaN(d.valueOf()); }
7. Add visual feedback to the headers to indicate the current sorting direction. This makes it clear to users how the data is organized and allows them to easily switch between ascending and descending order.
table thead tr th:after { position: absolute; right: 2px; } table thead tr th.sort-asc:after { content: "\25b4"; } table thead tr th.sort-desc:after { content: "\25be"; }
This awesome jQuery plugin is developed by orangeable. For more Advanced Usages, please check the demo page or visit the official website.
- Prev: Fast JSON to Table Converter with jQuery and TailwindCSS
- Next: None