Zoomable & Scrollable Schedule Component For jQuery - skedTape
File Size: | 180 KB |
---|---|
Views Total: | 30946 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
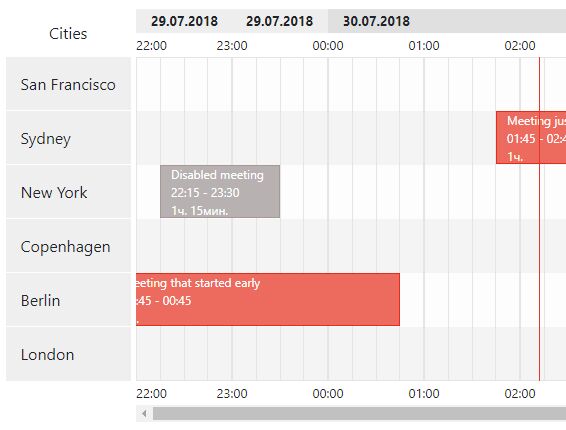
The jQuery skedTape generates a dynamic, zoomable and scrollable timeline to visualize scheduled events/tasks in a timesheet manner.
Features:
- Allows to adjust the zooming level of the timeline using +/- keys.
- Allows to scroll through the timeline with mouse wheel.
- Allows to show/hide the duration in entries.
- Allows to show/hide the date bar.
- Useful event handlers.
How to use it:
1. Add references to jQuery library and the jQuery skedTape plugin's files.
<link rel="stylesheet" href="/path/to/jquery.skedTape.css"> <script src="/path/to/jquery.min.js"></script> <script src="/path/to/jquery.skedTape.js"></script>
2. Create an array of events objects containging names, locations, and start/end times.
// helpers function today(hours, minutes) { var date = new Date(); date.setUTCHours(hours, minutes, 0, 0); return date; } function yesterday(hours, minutes) { var date = today(hours, minutes); date.setTime(date.getTime() - 24 * 60 * 60 * 1000); return date; } function tomorrow(hours, minutes) { var date = today(hours, minutes); date.setTime(date.getTime() + 24 * 60 * 60 * 1000); return date; } // events var events = [ { name: 'Meeting 1', location: 'london', start: today(4, 15), end: today(7, 30), url: null, class: '', // extra class disabled: false, // is disabled? data: {}, // data to set with $.data() method userData: {} // custom data }, { name: 'Meeting 2', location: 'london', start: today(7, 30), end: today(9, 15) }, { name: 'Meeting', location: '1', start: today(10, 0), end: today(11, 30) }, // more events here ];
3. Create a location object which will be displayed in the Y axis.
var locations = { '1': 'San Francisco', '2': 'Sydney', '3': 'New York', 'london': 'London', '5': 'Copenhagen', '6': 'Berlin' };
4. Render the schedule timeline on the webpage.
<div id="container"> ... </div>
var mySchedule = $('#container').skedTape({ caption: 'Cities', start: yesterday(22, 0), end: today(12, 0), showEventTime: true, showEventDuration: true, scrollWithYWheel: true, locations: locations, events: events, formatters: { date: function (date) { return $.fn.skedTape.format.date(date, 'l', '.'); }, duration: function (start, end, opts) { return $.fn.skedTape.format.duration(start, end, { hrs: 'ч.', min: 'мин.' });; }, } });
5. Options and defaults.
/** * caption text */ caption: '', /** * Max zoom level */ maxZoom: 10, /** * Default zooming up and down increment/decrement value. */ zoomStep: 0.5, /** * Initial zoom level. Minimum possible value is 1. */ zoom: 1, /** * Whether to show from-to dates in events. */ showEventTime: false, /** * Whether to show duration in events. */ showEventDuration: false, /** * Whether to show dates bar. */ showDates: true, /** * Minimum time between events required for the user to be able to add an event. */ minGapTimeBetween: 0, /** * Minimum gap between events to show minutes. */ minTimeGapShown: 1 * MS_PER_MINUTE, /** * Maximum gap between events to show minutes. */ maxTimeGapShown: 60 * MS_PER_MINUTE - 1, /** * Minimum gap to DO NOT highlight adjacent events. */ maxTimeGapHi: false, /** * Enables horizontal timeline scrolling with vertical mouse wheel. */ scrollWithYWheel: false, /** * Enables sorting of locations. */ sorting: false, /** * Specifies sorting columns. The property accepts two possible values: * 'order' (sorting by the property 'order' provided in location objects) * or 'name' (locale-aware case insensitive comparison by name). */ orderBy: 'order', /** * The number of minutes the dummy event will be snapped to. To * disable snapping set any falsy value. */ snapToMins: 1, /** * Right Mouse Button cancels adding a new event. */ rmbCancelsAdding: true, /** * Default timezone for locations, takes effect when you don't specify it * in location descriptor. The default value is a browser's current timezone. */ tzOffset: CURRENT_TZ_OFFSET, /** * Enables or disables showing serifs on time indicator lines. */ timeIndicatorSerifs: false, /** * Enables or disables showing intervals between events. */ showIntermission: false, /** * Interval (in minutes) between events to show intermission time when it is * enabled. */ intermissionRange: [1, 60], /** * The default behavior is to show pop-ups for events that are either too * small to be visible or partially outside the timeline. The possible * options are: "default", "always" or "never". */ showPopovers: 'default', /** * The hook invoked to determine whether an event may be added to a location. * The default implementation always returns *true*. * You should avoid mutating the arguments in this hook (that may cause * unexpected behaviour). * * @see beforeAddIntoLocation() */ canAddIntoLocation: function(location, event) { return true; }, /** * Invoked after getting a positive result from the `canAddIntoLocation()` * hook just before updating the event. Here you can place any logic that * mutates the event object given. * * @see canAddIntoLocation() */ beforeAddIntoLocation: function(location, event) {}, /** * The mixin is applied to every location's DOM element when rendering the sidebar. * The callback takes 3 arguments: jQuery text element node representing * the location, the corresponding location object and a boolean value * specifying the result of executing the `canAddIntoLocation()` function on * the location and dummy event. * The value of the last argument is undefined if the function is called * while no event is being dragged. */ postRenderLocation: function($el, location, canAdd) { SkedTape.prototype.postRenderLocation.call(this, $el, location, canAdd); }, /** * The mixin applied to every event DOM element on the timeline after * rendering is complete and before actual inserting to the DOM tree of the * document. The default implementation does nothing, you may feel free to * replace it with your own code that modifies the default representation of * events on a timeline. */ postRenderEvent: function($event, event) {},
6. Event handlers available.
/* props for all click event/contextmenu events: detail.locationId detail.date Click position converted to datetime on timeline. relatedTarget pageX, offsetX, etc */ mySchedule.on('intersection:click.skedtape', function(e) { // do something }); mySchedule.on('intersection:contextmenu.skedtape', function(e) { // do something }); mySchedule.on('timeline:click.skedtape', function(e) { // do something }); mySchedule.on('timeline:contextmenu.skedtape', function(e) { // do something }); mySchedule.on('event:click.skedtape', function(e) { // do something }); mySchedule.on('event:contextmenu.skedtape', function(e) { // do something }); mySchedule.on('event:dragStart.skedtape', function(e) { // do something }); mySchedule.on('event:dragStarted.skedtape', function(e) { // do something }); mySchedule.on('event:dragEnd.skedtape', function(e) { // do something }); mySchedule.on('event:dragEnded.skedtape', function(e) { // do something }); mySchedule.on('event:dragEndRefused.skedtape', function(e) { // do something }); mySchedule.on('skedtape:event:dragCanceled', function(e) { // do something }); mySchedule.on('skedtape:event:addingCanceled', function(e) { // do something });
Changelog:
v2.5.0 (2020-07-20)
- Added showPopovers option
v2.4.6 (2020-05-27)
- added hasIntersections() method
v2.4.2 (2019-10-07)
- fixed event's active state
v2.3.0 (2019-09-12)
- added dragEvent method
v2.2.5 (2019-09-01)
- fixed adding dummyEvent w/o location
v2.2.2 (2019-08-21)
- FIX: default date formatter now uses local time
v2.2.0 (2019-08-10)
- added intermissions
v2.1.0 (2019-08-08)
- added postRenderEvent
v2.0.1 (2019-08-07)
- fixed misspelling
v2.0.0 (2019-06-02)
- Doesn't work with dates in UTC timezone anymore. Now you should pass all your dates to the plugin in local timezone, and they'll be shown in it (or any you specify) as well.
- Added: Every location now may has individual timezone offset.
v1.4.5 (2019-05-29)
- fixed destroy method
v1.4.3 (2019-02-22)
- Fixed for IE 11
v1.4.1 (2018-11-23)
- improved events
v1.3.1 (2018-11-14)
- fixed duration output error
2018-10-17
- added beforeAddIntoLocation() callback
2018-09-09
- better forbidden location styling
2018-09-05
- showing popover for overflowing events too
2018-09-04
- fixed current time line overlapping by gap's background
2018-09-02
- added setSnapToMinutes
2018-08-21
- improved rendering feedback, added min gap
2018-08-19
- implemented dragging events
2018-08-17
- fixed canAddIntoLocation may have taken null location
2018-08-10
- v1.2.1: preliminent events
2018-08-07
- improved sorting
2018-08-03
- rows was sorted by location name
2018-08-02
- v1.0.6: fixed entry row gap rendering issue
This awesome jQuery plugin is developed by lexkrstn. For more Advanced Usages, please check the demo page or visit the official website.