Minimalist Full Window Scroll Snap Plugin With jQuery
File Size: | 2.07 KB |
---|---|
Views Total: | 3402 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
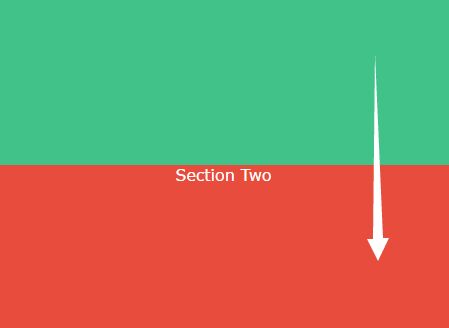
A jQuery script that provides a simple way to create a full window one page scroll website with smooth scroll snapping functionality. After scrolling, the webpage will automatically scroll and smoothly snap to the next/prev content section depending on the current scroll position.
How to use it:
1. Create a group of DIV container for the content sections.
<div id="one" class="window section-1"></div> <div id="two" class="window section-2"></div> <div id="three" class="window section-3"></div>
2. Make the content sections full window.
body, html { widht: 100%; height: 100%; margin: 0; padding: 0; } .window { width: 100%; height: 100%; }
3. Place the needed jQuery library in the webpage.
<script src="//code.jquery.com/jquery.min.js"></script>
4. Declare section & current scroll position variables:
var sections = ['#one', '#two', '#three']; var currentPos = 0;
5. The core function:
function scrollListen () { $(window).on('scroll', function(){ $(window).off('scroll'); let origin = $(window).scrollTop(); let delta = 0; $(window).on('scroll', () => { delta = origin - $(window).scrollTop(); if(Math.abs(delta) > 25){ $(window).off('scroll'); if(delta < 0 && sections[currentPos + 1]){ currentPos++; setTimeout(function() { $('html, body').animate({scrollTop: $(sections[currentPos]).offset().top}, 500, function() { scrollListen(); }); }, 500); }else if(delta > 0 && sections[currentPos - 1]){ currentPos--; setTimeout(function() { $('html, body').animate({scrollTop: $(sections[currentPos]).offset().top}, 500, function() { scrollListen(); }); }, 500); } } }); }); }
6. Calculate the window's height and apply the scroll snap functionality to the content sections.
$(function() { $('.window').css({ 'height': window.innerHeight, }); $(window).resize(() => { $('.window').css({ 'height': window.innerHeight, }); }); scrollListen(); });
This awesome jQuery plugin is developed by THEAverageSpeedBurri. For more Advanced Usages, please check the demo page or visit the official website.